Write in C++ Sam is making a list of his favorite Pokemon. However, he changes his mind a lot. Help Sam write a function insertAfter() that takes five parameters and inserts the name of a Pokemon right after a specific index. Function specifications Name: insertAfter() Parameters (Your function should accept these parameters IN THIS ORDER): input_strings string: The array containing strings num_elements int: The number of elements that are currently stored in the array arr_size int: The number of elements that can be stored in the array index int: The location to insert a new string. Note that the new string should be inserted after this location. string_to_insert string: The new string to be inserted into the array Return Value: bool: true: If the string is successfully inserted into the array false: If the array is full If the index value exceeds the size of the array
Write in C++ Sam is making a list of his favorite Pokemon. However, he changes his mind a lot. Help Sam write a function insertAfter() that takes five parameters and inserts the name of a Pokemon right after a specific index. Function specifications Name: insertAfter() Parameters (Your function should accept these parameters IN THIS ORDER): input_strings string: The array containing strings num_elements int: The number of elements that are currently stored in the array arr_size int: The number of elements that can be stored in the array index int: The location to insert a new string. Note that the new string should be inserted after this location. string_to_insert string: The new string to be inserted into the array Return Value: bool: true: If the string is successfully inserted into the array false: If the array is full If the index value exceeds the size of the array
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter8: Arrays And Strings
Section: Chapter Questions
Problem 44SA
Related questions
Question
Write in C++
Sam is making a list of his favorite Pokemon. However, he changes his mind a lot. Help Sam write a function insertAfter() that takes five parameters and inserts the name of a Pokemon right after a specific index.
Function specifications
- Name: insertAfter()
- Parameters (Your function should accept these parameters IN THIS ORDER):
- input_strings string: The array containing strings
- num_elements int: The number of elements that are currently stored in the array
- arr_size int: The number of elements that can be stored in the array
- index int: The location to insert a new string. Note that the new string should be inserted after this location.
- string_to_insert string: The new string to be inserted into the array
- Return Value: bool:
- true: If the string is successfully inserted into the array
- false:
- If the array is full
- If the index value exceeds the size of the array
![Sam is making a list of his favorite Pokemon. However, he changes his mind a lot. Help Sam write a function insertAfter() that takes five
parameters and inserts the name of a Pokemon right after a specific index.
Function specifications
• Name: insertAfter()
• Parameters (Your function should accept these parameters IN THIS ORDER):
o input_strings string: The array containing strings
• num_elements int: The number of elements that are currently stored in the array
o arr_size int: The number of elements that can be stored in the array
o index int: The location to insert a new string. Note that the new string should be inserted after this location.
string_to_insert string: The new string to be inserted into the array
• Return Value: bool:
O true : If the string is successfully inserted into the array
O
O
false :
■ If the array is full
■ If the index value exceeds the size of the array
Sample run 1: Function Call
int size = 5;
string input_strings [size] = {"pikachu", "meowth", "snorlax"};
int num_elements
int index =
2;
=
3;
string string_to_insert =
"clefairy";
// result contains the value returned by insert After
insertAfter (input_strings, num_elements, size, index, string_to_insert);
bool result =
// print result
cout << "Function returned value: "<< result << endl;
// print array contents
for(int i = 0; i < size; i++)
{
cout <<input_strings [i] << endl;](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fb1d14486-aab8-45fa-9185-3d15b7af8b4b%2F95d11a29-ef0c-470f-b152-8e47862d1254%2Fu8bov8_processed.png&w=3840&q=75)
Transcribed Image Text:Sam is making a list of his favorite Pokemon. However, he changes his mind a lot. Help Sam write a function insertAfter() that takes five
parameters and inserts the name of a Pokemon right after a specific index.
Function specifications
• Name: insertAfter()
• Parameters (Your function should accept these parameters IN THIS ORDER):
o input_strings string: The array containing strings
• num_elements int: The number of elements that are currently stored in the array
o arr_size int: The number of elements that can be stored in the array
o index int: The location to insert a new string. Note that the new string should be inserted after this location.
string_to_insert string: The new string to be inserted into the array
• Return Value: bool:
O true : If the string is successfully inserted into the array
O
O
false :
■ If the array is full
■ If the index value exceeds the size of the array
Sample run 1: Function Call
int size = 5;
string input_strings [size] = {"pikachu", "meowth", "snorlax"};
int num_elements
int index =
2;
=
3;
string string_to_insert =
"clefairy";
// result contains the value returned by insert After
insertAfter (input_strings, num_elements, size, index, string_to_insert);
bool result =
// print result
cout << "Function returned value: "<< result << endl;
// print array contents
for(int i = 0; i < size; i++)
{
cout <<input_strings [i] << endl;
![Output
Function returned value: 1
pikachu
meowth
snorlax
clefairy
Sample run 2 Function Call
int size = 5;
string input_strings [size]
int num_elements 2;
int index =
}
0;
string string_to_insert = "bulbasaur";
// result contains the value returned by insert After
bool result = insertAfter (input_strings, num_elements, size, index, string_to_insert);
// print result
cout << "Function returned value: "<< result << endl;
// print array contents
for(int i = 0; i < size; i++)
{
Output
=
cout <<input_strings[i] << endl;
Function returned value: 1
caterpie
bulbasaur
eevee
{"caterpie", "eevee"};](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fb1d14486-aab8-45fa-9185-3d15b7af8b4b%2F95d11a29-ef0c-470f-b152-8e47862d1254%2Fa3xv8zj_processed.png&w=3840&q=75)
Transcribed Image Text:Output
Function returned value: 1
pikachu
meowth
snorlax
clefairy
Sample run 2 Function Call
int size = 5;
string input_strings [size]
int num_elements 2;
int index =
}
0;
string string_to_insert = "bulbasaur";
// result contains the value returned by insert After
bool result = insertAfter (input_strings, num_elements, size, index, string_to_insert);
// print result
cout << "Function returned value: "<< result << endl;
// print array contents
for(int i = 0; i < size; i++)
{
Output
=
cout <<input_strings[i] << endl;
Function returned value: 1
caterpie
bulbasaur
eevee
{"caterpie", "eevee"};
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
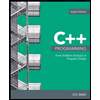
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
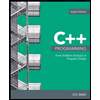
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning