How to fix
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
How to fix?
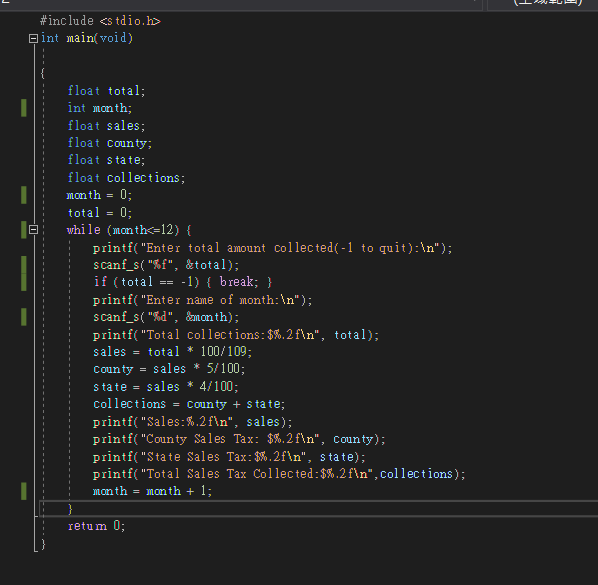
Transcribed Image Text:#include <s tdio.h>
Qint main(void)
float total;
int manth;
float sales;
float County;
float state;
float collections;
Month = 0;
total = 0;
while (month<=12) {
printf( "Enter total amount collected( -1 to quit):\n");
scanf_s( "%f", &total);
if (total
-1) { break; }
==
printf( "Enter name of month:\n");
scanf_s( "%d", &anonth);
printf( "Total collections: $%.2 f\n", total);
= total * 100/109;
sales
County = sales * 5/100;
s tate = sales * 4/100;
collections
= County + state;
printí( "Sales:%.2 f\n", sales);
printf( "County Sales Tax: $%. 2 f\n", County);
printf( "State Sales Tax: $%.2f\n", state);
printf( "Total Sales Tax Collected:$%.2 f\n", collections);
Month = month + 1;
retum U;
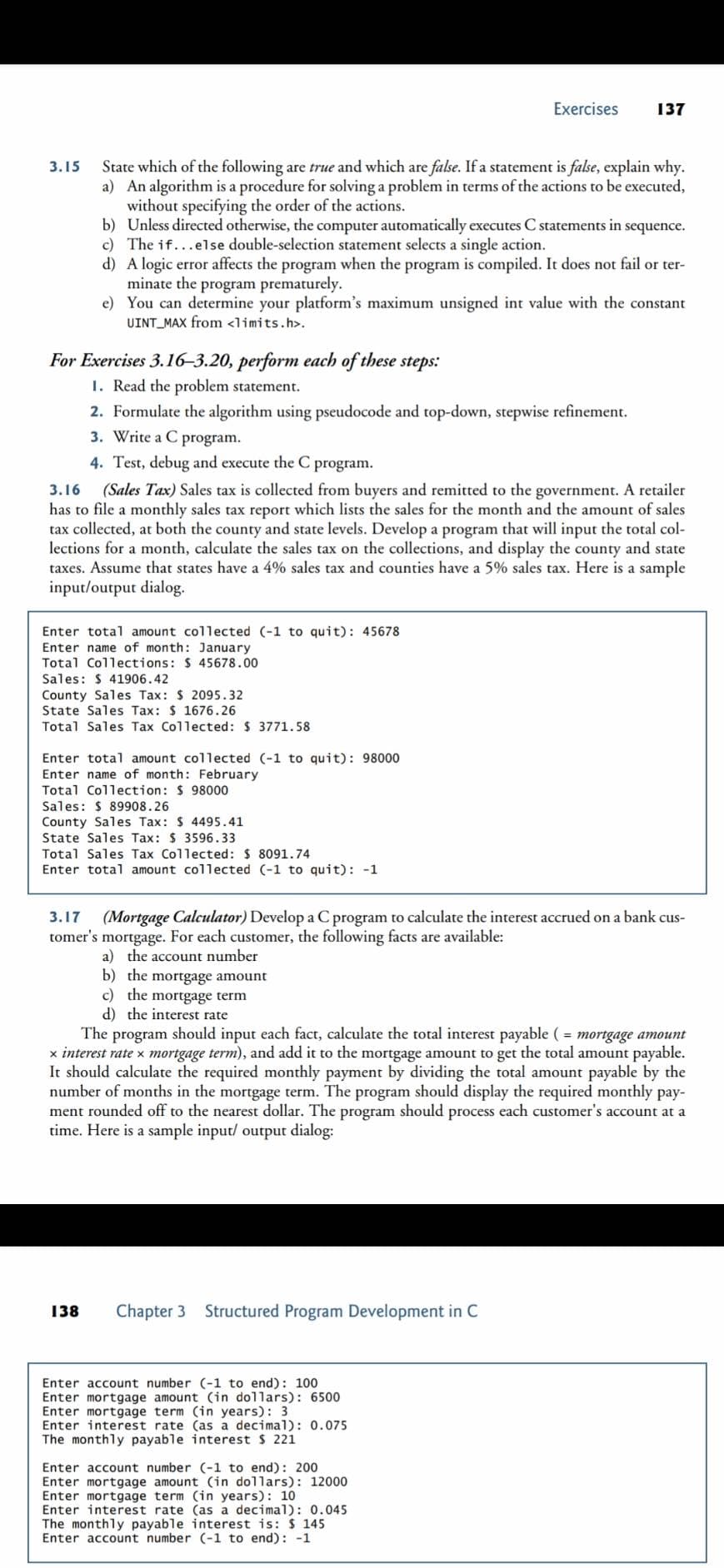
Transcribed Image Text:Exercises
137
State which of the following are true and which are false. If a statement is false, explain why.
a) An algorithm is a procedure for solving a problem in terms of the actions to be executed,
without specifying the order of the actions.
b) Unless directed otherwise, the computer automatically executes C statements in sequence.
c) The if...else double-selection statement selects a single action.
d) A logic error affects the program when the program is compiled. It does not fail or ter-
minate the program prematurely.
e) You can determine your platform's maximum unsigned int value with the constant
UINT_MAX from <limits.h>.
3.15
For Exercises 3.16-3.20, perform each of these steps:
1. Read the problem statement.
2. Formulate the algorithm using pseudocode and top-down, stepwise refinement.
3. Write a C program.
4. Test, debug and execute the C
program.
3.16
(Sales Tax) Sales tax is collected from buyers and remitted to the government. A retailer
has to file a monthly sales tax report which lists the sales for the month and the amount of sales
tax collected, at both the county and state levels. Develop a program that will input the total col-
lections for a month, calculate the sales tax on the collections, and display the county and state
taxes. Assume that states have a 4% sales tax and counties have a 5% sales tax. Here is a sample
input/output dialog.
Enter total amount collected (-1 to quit): 45678
Enter name of month: January
Total Collections: $ 45678.00
Sales: $ 41906.42
County Sales Tax: $ 2095.32
State Sales Tax: $ 1676.26
Total Sales Tax Collected: $ 3771.58
Enter total amount collected (-1 to quit): 98000
Enter name of month: February
Total Collection: $ 98000
Sales: $ 89908.26
County Sales Tax: $ 4495.41
State Sales Tax: $ 3596.33
Total Sales Tax Collected: $ 8091.74
Enter total amount collected (-1 to quit): -1
3.17
(Mortgage Calculator) Develop a C program to calculate the interest accrued on a bank cus-
tomer's mortgage. For each customer, the following facts are available:
a) the account number
b) the mortgage amount
c) the mortgage term
d) the interest rate
The program should input each fact, calculate the total interest payable ( = mortgage amount
x interest rate x mortgage term), and add it to the mortgage amount to get the total amount payable.
ould calculate the required monthly payment by dividing
number of months in the mortgage term. The program should display the required monthly pay-
ment rounded off to the nearest dollar. The program should process each customer's account at a
time. Here is a sample input/ output dialog:
It
total amount payable by the
138
Chapter 3
Structured Program Development in C
Enter account number (-1 to end): 100
Enter mortgage amount (in dollars): 6500
Enter mortgage term (in years): 3
Enter interest rate (as a decimal): 0.075
The monthly payable interest $ 221
Enter account number (-1 to end): 200
Enter mortgage amount (in dollars): 12000
Enter mortgage term (in years): 10
Enter interest rate (as a decimal): 0.045
The monthly payable interest is: $ 145
Enter account number (-1 to end): -1
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
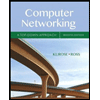
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
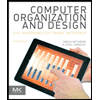
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
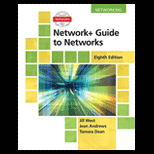
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
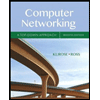
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
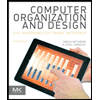
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
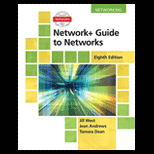
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
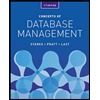
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
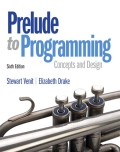
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
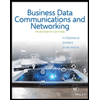
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY