How to use the try-catch statement in the driver program when instatiating a new paramerized Fraction object. You do not need to show entire programs, only the requested lines of source code. Below is my driver program: #include #include "fraction.h" using namespace std; Fraction frac1, frac2; Fraction allotFraction(Fraction& someFraction); int main() { Fraction frac3(8, 16); cout << "1st Fraction" << endl; allotFraction(frac1); cout << " " << endl; cout << "2nd Fraction" << endl; allotFraction(frac2); cout << " " << endl; cout << "1st Fraction < 2nd Fraction?: "; if (frac1.IsLessThan(frac2) == true) cout << "Yes" << endl; else cout << "No" << endl; cout << "1st Fraction = 2nd Fraction?: "; if (frac1.IsEqual(frac2) == true) cout << "Yes" << endl; else cout << "No" << endl; cout << "1st Fraction > 2nd Fraction?: "; if (frac1.IsGreaterThan(frac2) == true) cout << "Yes" << endl; else cout << "No" << endl; cout << endl; Fraction tranFraction = frac1.Add(frac2); cout << "Sum = "; tranFraction.Write(); tranFraction = frac1.Multiply(frac2); cout << "Product = "; tranFraction.Write(); tranFraction = frac1.Divide(frac2); cout << "Quotient = "; tranFraction.Write(); return 0; } Fraction allotFraction(Fraction& someFraction) { int n; int d; cout << "Enter Numerator: "; cin >> n; cout << "Enter Denominator: "; cin >> d; Fraction tranFrac(n, d); someFraction = tranFrac; cout << "Fraction: "; someFraction.Write(); cout << "Reduced: "; someFraction.Reduce(); someFraction.Write(); return someFraction; }
How to use the try-catch statement in the driver program when instatiating a new paramerized Fraction object. You do not need to show entire programs, only the requested lines of source code. Below is my driver program:
#include <iostream>
#include "fraction.h"
using namespace std;
Fraction frac1, frac2;
Fraction allotFraction(Fraction& someFraction);
int main()
{
Fraction frac3(8, 16);
cout << "1st Fraction" << endl;
allotFraction(frac1);
cout << " " << endl;
cout << "2nd Fraction" << endl;
allotFraction(frac2);
cout << " " << endl;
cout << "1st Fraction < 2nd Fraction?: ";
if (frac1.IsLessThan(frac2) == true)
cout << "Yes" << endl;
else
cout << "No" << endl;
cout << "1st Fraction = 2nd Fraction?: ";
if (frac1.IsEqual(frac2) == true)
cout << "Yes" << endl;
else
cout << "No" << endl;
cout << "1st Fraction > 2nd Fraction?: ";
if (frac1.IsGreaterThan(frac2) == true)
cout << "Yes" << endl;
else
cout << "No" << endl;
cout << endl;
Fraction tranFraction = frac1.Add(frac2);
cout << "Sum = ";
tranFraction.Write();
tranFraction = frac1.Multiply(frac2);
cout << "Product = ";
tranFraction.Write();
tranFraction = frac1.Divide(frac2);
cout << "Quotient = ";
tranFraction.Write();
return 0;
}
Fraction allotFraction(Fraction& someFraction)
{
int n;
int d;
cout << "Enter Numerator: ";
cin >> n;
cout << "Enter Denominator: ";
cin >> d;
Fraction tranFrac(n, d);
someFraction = tranFrac;
cout << "Fraction: ";
someFraction.Write();
cout << "Reduced: ";
someFraction.Reduce();
someFraction.Write();
return someFraction;
}

Step by step
Solved in 2 steps

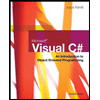
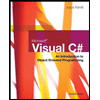