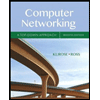
USE THE
CODE IN JAVA
1. Command Line Args
Modify problem # 1 from Lab 8 to accept the values for the key as command line arguments.
Your program may assume the arguments given at the command line are integers. If there are no
arguments, print a message. If there is at least one argument, compute and print the average of the arguments.
Note that you will need to use the parseInt method of the Integer class to extract integer values from the strings that are passed in. If any non-integer values are passed in, your program will produce an error, which is unavoidable at this point.
Test your program in NetBeans using the data for problem # 1 Lab 8 as command line arguments.
import java.util.Scanner;
import java.text.NumberFormat;
public class Quizzes
{
//----------------------------------------------
// Read in the number of questions followed by
// the key, then read in each student's answers
// and calculate the number and percent correct.
//----------------------------------------------
public static void main(String[] args)
{
int numQuestions;
int numCorrect;
String anotherQuiz;
int answer;
NumberFormat percent = NumberFormat.getPercentInstance();
Scanner scan = new Scanner (System.in);
System.out.println ("Quiz Grading");
System.out.println ();
System.out.println("Enter the number of questions on the quiz: ");
numQuestions = scan.nextInt();
//CREATE THE ARRAY FOR THE KEY
int[] key = new int[numQuestions];
System.out.println("Enter the answer key: ");
//LOAD THE ARRAY FOR THE ANSWER KEY WITH INPUT FROM THE USER
for (int i = 0; i < numQuestions; i++)
{
key[i] = scan.nextInt();
}
//OUTER LOOP TO ALLOW THE USER TO ENTER GRADES
// FOR ANY NUMBER OF QUIZZES
do
{
//INNER LOOP TO GET ANSWERS FROM THE USER AND COUNT THE NUMBER OF
// CORRECT ANSWERS
System.out.println("Enter the student answers: ");
numCorrect = 0;
for (int i = 0; i < numQuestions; i++)
{
answer = scan.nextInt();
if (answer == key[i])
{
numCorrect++;
}
}
//DISPLAY THE NUMBER OF CORRECT ANSWERS AND PERCENT
System.out.println("Number of correct answers: " + numCorrect +
" | Percent: " + percent.format((double)numCorrect/numQuestions));
System.out.println();
//ASK USER IF THEY WISH TO GRADE ANOTHER QUIZ
System.out.println("Grade another quiz? (y/n): ");
anotherQuiz = scan.next();
if (anotherQuiz.equalsIgnoreCase("y"))
{
}
else
{
System.out.println("Good-bye");
break;
}
} while (true);
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- Java code Screenshot and output is mustarrow_forwardLanguage is in Javaarrow_forwardFibonacci A fibonacci sequence is a series of numbers in which each number is the sum of the two preceding numbers. For example: 0, 1, 1, 2, 3, 5, 8, 13, 21, 34, and so on… For this, you will implement a class that takes a positive integer (n) and returns the number in the nth position in the sequence. Examples When n is 1, the returned value will be 0. When n is 4, the returned value will be 2. When n is 9, the returned value will be 21. come up with the formula and base cases Implementation Create a class Fibonacci with a public static method getValue. Create a Main class to test and run your Fibonacci class.arrow_forward
- Please include doctring, and write only in python: First, write a class named Movie that has four data members: title, genre, director, and year. It should have: an init method that takes as arguments the title, genre, director, and year (in that order) and assigns them to the data members. The year is an integer and the others are strings. get methods for each of the data members (get_title, get_genre, get_director, and get_year). Next write a class named StreamingService that has two data members: name and catalog. the catalog is a dictionary of Movies, with the titles as the keys and the Movie objects as the corresponding values (you can assume there aren't any Movies with the same title). The StreamingService class should have: an init method that takes the name as an argument, and assigns it to the name data member. The catalog data member should be initialized to an empty dictionary. get methods for each of the data members (get_name and get_catalog). a method named add_movie…arrow_forwardx = 3 + numbers[3]++; System.out.println(x); QUESTION 2: Class Rectangle has two data members, length (type of float) and width (type of float). The class Rectangle also has the following: //mutator methods void setlength (float len) } //B. length = len; H accessor methods float getLength() I return length; The following statement creates an array of Rectangle with size 2: Rectangle[] arrayRectangle= new Rectangle[2]; Is it correct if executing the following statements? What is the output? If it is not correct, write the correct one arrayRectangle. setLength (12.59); System.out.printin[rectangle Set.getLength()); arrayRectangle[1].setLength(4.15); System.out.println(arrayRectangle[1].getLength()): QUESTION 3: Use the following int array int[] numbers = { 42, 28, 36, 72, 17, 25, 81, 65, 23, 58} -Open file data.txt to write. to write the values of the array numbers to the file with the y numbers to get the valuesarrow_forwardIn C++arrow_forward
- In python and include doctring: First, write a class named Movie that has four data members: title, genre, director, and year. It should have: an init method that takes as arguments the title, genre, director, and year (in that order) and assigns them to the data members. The year is an integer and the others are strings. get methods for each of the data members (get_title, get_genre, get_director, and get_year). Next write a class named StreamingService that has two data members: name and catalog. the catalog is a dictionary of Movies, with the titles as the keys and the Movie objects as the corresponding values (you can assume there aren't any Movies with the same title). The StreamingService class should have: an init method that takes the name as an argument, and assigns it to the name data member. The catalog data member should be initialized to an empty dictionary. get methods for each of the data members (get_name and get_catalog). a method named add_movie that takes a Movie…arrow_forwardjavaarrow_forward!! E! 4 2 You are in process of writing a class definition for the class Book. It has three data attributes: book title, book author, and book publisher. The data attributes should be private. In Python, write an initializer method that will be part of your class definition. The attributes will be initialized with parameters that are passed to the method from the main program. Note: You do not need to write the entire class definition, only the initializer method lili lilıarrow_forward
- basic java please Write a method called findTip that finds the value of a meal as a double after adding the tip to the cost. Then return this value to the calling function. For example findTip(120.10, 5) would 126.105 because that is a 5% tip. While findTip(48.0, 15) would return 55.2 because that is a 15% tip. Note that the bill is a double while the tip is an int. Don’t worry about rounding to two decimal places, just return the full value as a double.arrow_forwardPlease use java only. In this assignment, you will implement a simple game in a class called SimpleGame. This game has 2 options for the user playing. Based on user input, the user can choose to either convert time, from seconds to hours, minutes, and seconds, or calculate the sum of all digits in an integer. At the beginning of the game, the user will be prompted to input either 1 or 2, to indicate which option of the game they want to play. 1 will indicate converting time, and 2 will indicate calculating the sum of digits in an integer. For converting time, the user will be prompted to input a number of seconds (as an int) and the program will call a method that will convert the seconds to time, in the format hours:minutes:seconds, and print the result. For example, if the user enters 6734, the program will print the time, 1:52:14. As another example, if the user enters 10,000, the program should print 2:46:39. For calculating the sum of digits in an integer, the user will be…arrow_forwardI already have the code for the assignment below, but the code has an error in the driver class. Please help me fix it. The assignment: Make a recursive method for factoring an integer n. First, find a factor f, then recursively factor n / f. This assignment needs a resource class and a driver class. The resource class and the driver class need to be in two separate files. The resource class will contain all of the methods and the driver class only needs to call the methods. The driver class needs to have only 5 lines of code The code of the resource class: import java.util.ArrayList;import java.util.List; public class U10E03R{ // Recursive function to // print factors of a number public static void factors(int n, int i) { // Checking if the number is less than N if (i <= n) { if (n % i == 0) { System.out.print(i + " "); } // Calling the function recursively // for the next number factors(n, i +…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
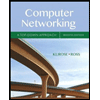
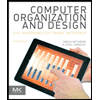
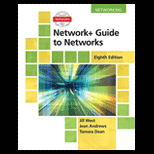
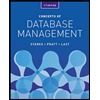
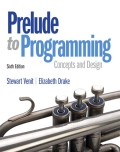
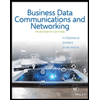