How would this look like? How do you add this table to a database?
Chapter13: Views
Section: Chapter Questions
Problem 4MC
Related questions
Question
How would this look like? How do you add this table to a database ?
![In the previous labs you built functions to respond to 2 queries:
1. CREATE DATABASE <NAME>
Where <NAME> is any single-word database name.
2. SHOW DATABASES
In this lab you will build a function to respond to 1 query:
1. CREATE TABLE <NAME> <ID> <ID>
Where <ID> is the names of table columns.
Since the system has multiple databases in it that your users can create tables in, your users must first be able to select which
database they want to create their tables in. The functionality for this has been provided for you. The query your users will enter
to select which database they want to create tables in will be:
USE <DATABASENAME>
Where <DATABASENAME> is the name of the database the user wants to create tables in.
This command will set a global variable currentDB to <DATABASENAME>. You will need to use currentDB in this lab.
Usage of this command can be seen in the example output.
LAB
Note: For this lab you will not need to edit anything in main. You will only write code for an addTable function.
CREATE TABLE <NAME> <ID> <ID>
This command will create the arrows from the Databases to the Tables.
In the main function in lab10.cpp under the case for CREATE you will notice a function call to a function called
createTable, this function creates a table in our database. If you look above the main function you will see the definition
for createTable.createTable takes in a name and a string containing options (the names the user wants to call the
columns in the table). The name it takes in is the name the user wants to call their table, and the string of options are the names
the user wants to call the columns in their table. The first thing createTable does is it turns the string of options into a
vector of options. It then calls a function called addTable which takes in a table name and a vector of column names. You
need to create this addTable function. Creating this function is described below
Above the createTable function, create a boolean-retuning function called addTable. This function should take in 2 formal
parameters, a string and a vector<string>. A prototype for your function is as follows:
bool addTable (string, const vector<string>6)
In the addTable function you need to add a table to your database. To add a table in your database:
1. Check if the table already exists in the database you are currently in.
a. Open the CSV file of tables for the current database you are in. This file
"csv/" + current DB + "_tables.csv"
b. Skip the line of headers in the CSV file from (a) using C++'s getline function.
c. Grab the first row from the CSV file from (a).
d. Call the provided function vectorizeRow (string) on the row you got from the previous step.
is located at:
i.
vectorizeRow is a vector<string>-returning function that turns the passed row from a csv file into a
vector of strings. i.e. "DB, exTable, 2" => ["DB", "exTable", "2"]
e. The vector you get back from (d) contains 3 elements:
vec[0] = The name of the database the table belongs to.
vec[1]= The name of the table.
vec[2] = How many columns are in the
If the vector's database name matches currentDB and table name matches the name of the table the user is
trying to create, the table is already in the database. Output an error message and return false from the
addTable function, The error message should say:
Error: Table already exists.
f. If (e) doesn't result in error, grab the next row from the CSV file of tables for the current database you are in
and continue from (d). If you get through the file without finding the table the user is trying to add, continue to
(2) as the table doesn't already exist and can be added.
2. If the table doesn't already exist add it to the database:
a.
Close, then open, in appendable mode, the CSV file of tables for the current database you are in.
b. Append the table the user wants to create to the CSV file of tables for the current database you are in. This can
be done by appending a new row to the file. The row you want to append is:
current DB, tableName, columnCount
Where tableName is the string and columnCount is the size of the vector passed to addTable.
c. Create a new CSV file to hold data for your table in the location:
"csv/" + current DB + "_" + name + ".csv"
d. Write the names of the headers passed to addTable via a vector<string> to the CSV file created in (c). Each
header in the file should be separated by a comma ',
e. Close all of the files opened in the function.
f. Return true as you were able to successfully add the table to the database.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fbeec80cd-dfb2-485f-bc0f-b51f7e21c377%2F69f01a45-0b82-49ae-bd12-5e6955c49365%2F0wr77_processed.jpeg&w=3840&q=75)
Transcribed Image Text:In the previous labs you built functions to respond to 2 queries:
1. CREATE DATABASE <NAME>
Where <NAME> is any single-word database name.
2. SHOW DATABASES
In this lab you will build a function to respond to 1 query:
1. CREATE TABLE <NAME> <ID> <ID>
Where <ID> is the names of table columns.
Since the system has multiple databases in it that your users can create tables in, your users must first be able to select which
database they want to create their tables in. The functionality for this has been provided for you. The query your users will enter
to select which database they want to create tables in will be:
USE <DATABASENAME>
Where <DATABASENAME> is the name of the database the user wants to create tables in.
This command will set a global variable currentDB to <DATABASENAME>. You will need to use currentDB in this lab.
Usage of this command can be seen in the example output.
LAB
Note: For this lab you will not need to edit anything in main. You will only write code for an addTable function.
CREATE TABLE <NAME> <ID> <ID>
This command will create the arrows from the Databases to the Tables.
In the main function in lab10.cpp under the case for CREATE you will notice a function call to a function called
createTable, this function creates a table in our database. If you look above the main function you will see the definition
for createTable.createTable takes in a name and a string containing options (the names the user wants to call the
columns in the table). The name it takes in is the name the user wants to call their table, and the string of options are the names
the user wants to call the columns in their table. The first thing createTable does is it turns the string of options into a
vector of options. It then calls a function called addTable which takes in a table name and a vector of column names. You
need to create this addTable function. Creating this function is described below
Above the createTable function, create a boolean-retuning function called addTable. This function should take in 2 formal
parameters, a string and a vector<string>. A prototype for your function is as follows:
bool addTable (string, const vector<string>6)
In the addTable function you need to add a table to your database. To add a table in your database:
1. Check if the table already exists in the database you are currently in.
a. Open the CSV file of tables for the current database you are in. This file
"csv/" + current DB + "_tables.csv"
b. Skip the line of headers in the CSV file from (a) using C++'s getline function.
c. Grab the first row from the CSV file from (a).
d. Call the provided function vectorizeRow (string) on the row you got from the previous step.
is located at:
i.
vectorizeRow is a vector<string>-returning function that turns the passed row from a csv file into a
vector of strings. i.e. "DB, exTable, 2" => ["DB", "exTable", "2"]
e. The vector you get back from (d) contains 3 elements:
vec[0] = The name of the database the table belongs to.
vec[1]= The name of the table.
vec[2] = How many columns are in the
If the vector's database name matches currentDB and table name matches the name of the table the user is
trying to create, the table is already in the database. Output an error message and return false from the
addTable function, The error message should say:
Error: Table already exists.
f. If (e) doesn't result in error, grab the next row from the CSV file of tables for the current database you are in
and continue from (d). If you get through the file without finding the table the user is trying to add, continue to
(2) as the table doesn't already exist and can be added.
2. If the table doesn't already exist add it to the database:
a.
Close, then open, in appendable mode, the CSV file of tables for the current database you are in.
b. Append the table the user wants to create to the CSV file of tables for the current database you are in. This can
be done by appending a new row to the file. The row you want to append is:
current DB, tableName, columnCount
Where tableName is the string and columnCount is the size of the vector passed to addTable.
c. Create a new CSV file to hold data for your table in the location:
"csv/" + current DB + "_" + name + ".csv"
d. Write the names of the headers passed to addTable via a vector<string> to the CSV file created in (c). Each
header in the file should be separated by a comma ',
e. Close all of the files opened in the function.
f. Return true as you were able to successfully add the table to the database.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
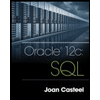
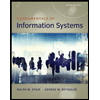
Fundamentals of Information Systems
Computer Science
ISBN:
9781337097536
Author:
Ralph Stair, George Reynolds
Publisher:
Cengage Learning
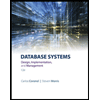
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781305627482
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
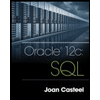
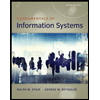
Fundamentals of Information Systems
Computer Science
ISBN:
9781337097536
Author:
Ralph Stair, George Reynolds
Publisher:
Cengage Learning
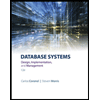
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781305627482
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
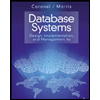
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781285196145
Author:
Steven, Steven Morris, Carlos Coronel, Carlos, Coronel, Carlos; Morris, Carlos Coronel and Steven Morris, Carlos Coronel; Steven Morris, Steven Morris; Carlos Coronel
Publisher:
Cengage Learning
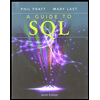
A Guide to SQL
Computer Science
ISBN:
9781111527273
Author:
Philip J. Pratt
Publisher:
Course Technology Ptr
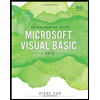
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning