I am given these 2 skeleton codes in java. I have ran hundreds of different codes, but I am completely lost. Please help! 1) package BankAccount; public class BankAccount { public String name; public int accNo; private int balance = 0; // This is your constructor! // Make sure to put in a String, int, and a double when creating a BankAccount object! /** * Constructs a new BankAccount object with the specified name, account number, and initial balance. * * @param name The name of the account holder. * @param accNo The account number. * @param balance The initial balance of the account. */ public BankAccount(String name, int accNo, int balance) { this.name = name; this.accNo = accNo; this.balance = balance; } /** * Sets the name of the account holder. * * @param name The new name of the account holder. */ public void setName(String name) { this.name = name; } /** * Gets the name of the account holder. * * @return The name of the account holder. */ public String getName() { r eturn this.name; } // Create getter and setter for accNo // Create getter and setter for balance // TODO Create the necessary methods here } 2) package BankAccount; public class BankAccountTester { public static void main(String[] args) { // Creating a test1 object where: // name is "John Smith" / / accNo is 153 // balance is 4500 BankAccount test1 = new BankAccount("John Smith", 153, 4500); //System.out.println(b.name); /*This statement will raise an error * as the "first" variable in BankAccount class is defined as * private and it cannot be accessed in a public class, * you can only view the details using getter methods you define*/ // test the the methods down here! // BEFORE: name of test1 is "John Smith" System.out.println(test1.getName()); / / AFTER: name of test1 is "Alice Bob" test1.setName("Alice Bob"); System.out.println(test1.getName()); // TODO These are my guidelines: Download BankAccount.java and BankAccountTester.java and place them in your package/folder. These skeleton files are “barebones” and need to be modified. Feel free to add into it or even remove code from it! You may need to add some private member variables. Make modifications to BankAccount.java file to solve the following questions: 1. Create “getter methods” and return the full name and account number of a customer - Sometimes, you don’t have to create getters and setters for EVERY variable! - We've implemented the setters for you! 2. Create methods to help customers: (a) deposit into their account balance (b) withdraw from their account balance (c) get (look at) their account balance Make sure you give them a good name based on their variable names! 3. Create two methods called setLoan and getLoan to: (a) give one customer a $5000 loan and the second customer a $10,000 loan both at an interest rate of 5% per year. The formula for interest given principal amount, rate of interest, and time: which also means “P + P*r*t” (b) return the total interest to be paid with a principal for a period of 3 years. 4. Create a method to check whether the account number is an Armstrong number or not. The method returns true if it is an Armstrong number, otherwise it will return false. - An Armstrong number is always 3 digits for which the cube of each digit can be summed up to equal the original number - 371 is an Armstrong number because 3*3*3 + 7*7*7 + 1*1*1 = 371 . - Inputting 153 should output true - Inputting 123 should output false Don’t forget Javadocs (docstring) for your methods! Usually, you don’t need them for your main method or your getters and setters. You also don’t need them in your equals or toString method either.
I am given these 2 skeleton codes in java. I have ran hundreds of different codes, but I am completely lost. Please help!
1)
package BankAccount;
public class BankAccount {
public String name;
public int accNo;
private int balance = 0;
// This is your constructor!
// Make sure to put in a String, int, and a double when creating a BankAccount object!
/**
* Constructs a new BankAccount object with the specified name, account number, and initial balance.
*
* @param name The name of the account holder.
* @param accNo The account number.
* @param balance The initial balance of the account.
*/
public BankAccount(String name, int accNo, int balance) {
this.name = name;
this.accNo = accNo;
this.balance = balance;
}
/**
* Sets the name of the account holder.
*
* @param name The new name of the account holder.
*/
public void setName(String name) {
this.name = name;
}
/**
* Gets the name of the account holder.
*
* @return The name of the account holder.
*/
public String getName() { r
eturn this.name;
}
// Create getter and setter for accNo
// Create getter and setter for balance
// TODO Create the necessary methods here
}
2)
package BankAccount;
public class BankAccountTester {
public static void main(String[] args) {
// Creating a test1 object where:
// name is "John Smith" /
/ accNo is 153
// balance is 4500
BankAccount test1 = new BankAccount("John Smith", 153, 4500);
//System.out.println(b.name);
/*This statement will raise an error
* as the "first" variable in BankAccount class is defined as
* private and it cannot be accessed in a public class,
* you can only view the details using getter methods you define*/
// test the the methods down here!
// BEFORE: name of test1 is "John Smith"
System.out.println(test1.getName()); /
/ AFTER: name of test1 is "Alice Bob"
test1.setName("Alice Bob");
System.out.println(test1.getName());
// TODO
These are my guidelines:
Download BankAccount.java and BankAccountTester.java and place them in your package/folder. These skeleton files are “barebones” and need to be modified. Feel free to add into it or even remove code from it! You may need to add some private member variables.
Make modifications to BankAccount.java file to solve the following questions:
1. Create “getter methods” and return the full name and account number of a customer - Sometimes, you don’t have to create getters and setters for EVERY variable! - We've implemented the setters for you!
2. Create methods to help customers:
(a) deposit into their account balance
(b) withdraw from their account balance
(c) get (look at) their account balance
Make sure you give them a good name based on their variable names!
3. Create two methods called setLoan and getLoan to: (a) give one customer a $5000 loan and the second customer a $10,000 loan both at an interest rate of 5% per year.
The formula for interest given principal amount, rate of interest, and time: which also means “P + P*r*t” (b) return the total interest to be paid with a principal for a period of 3 years.
4. Create a method to check whether the account number is an Armstrong number or not. The method returns true if it is an Armstrong number, otherwise it will return false.
- An Armstrong number is always 3 digits for which the cube of each digit can be summed up to equal the original number
- 371 is an Armstrong number because 3*3*3 + 7*7*7 + 1*1*1 = 371
. - Inputting 153 should output true
- Inputting 123 should output false
Don’t forget Javadocs (docstring) for your methods! Usually, you don’t need them for your main method or your getters and setters. You also don’t need them in your equals or toString method either.

Step by step
Solved in 5 steps with 4 images

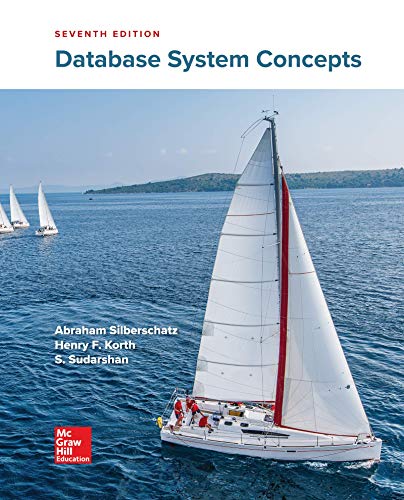
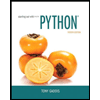
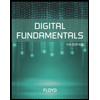
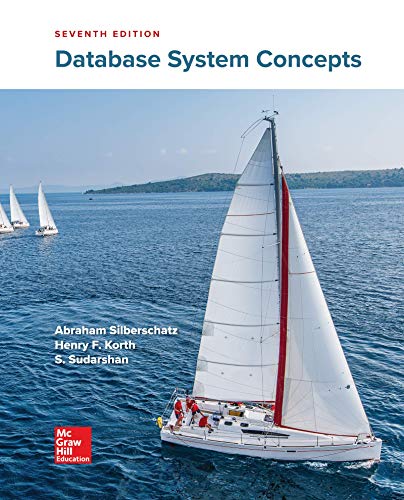
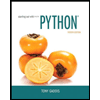
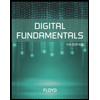
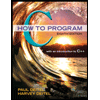
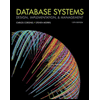
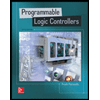