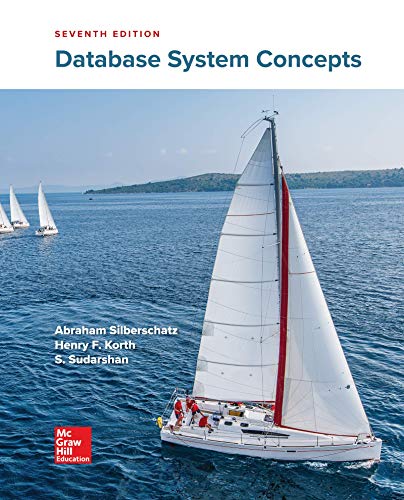
Concept explainers
How do I create this in Java?
Create the class Employee with int id, String name, double salary and int numberOfDependents as
private attributes. Add the Setters and Getters for each of those attributes, and override the toString
method to print an employee in the format [id,name,net salary], where:
Net salary = salary*0.91 + (numberOfDependent *0.01*salary)
In this program, two employee objects are equal when they have the same net salary. For that,
override the equals method (the one inherited from Object) so that emp1.equals(emp2) is true when
emp1 and emp2 are two employee objects that are equal.
In the main method, declare list to be an ArrayList of type Employee, and add at least 3 employees. Sort
and print the list in ascending order (with respect to the net salary). The code may use the Java sort
static method from the Collections class. If so, you may either implement the Java Comparable interface
or the Java Comparator interface.
Below can be used as the code of your main method:
emp2 = new Employee(598, "Jen Johnson", 47370, 5),
emp3 = new Employee(920, "Jan Jones", 47834.25, 1);
System.out.println(emp1.equals(emp3));
ArrayList<Employee> list = new ArrayList<>();
list.add(emp1);
list.add(emp2);
list.add(emp3);
//sort call goes here...
for (Employee e : list)
System.out.println(e);

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 4 images

- PLEASE DO IN JAVA (for programs like Eclipse) Create a UEmployee class that contains member variables for the university employee name and salary.The UEmployee class should contain member methods for returning the employee name and salary.Create Faculty and Staff classes that inherit the UEmployee class. The Faculty class should includemembers for storing and returning the department name. The Staff class should include members forstoring and returning the job title. User should be able to put in all the information like there name, department and salary. But for department type user should be given options and then told to pick. I have this code, but it does not work for me package UEmployee; import java.util.*; class UEmployee{//delaration of name and salaryString empName;double salary;//constructor of UEmployeeUEmployee(String empName,double salary){this.empName=empName;this.salary=salary;}//function to return the employee nameString getName(){return empName;} //function to…arrow_forwardThis is in Java. The assignement is to create a class. I am confused on how to write de code for an array that is up to 5. Also I am confused regarding how to meet the requirements of the constructor. This is what I have. public class InventoryOnShelf { //fields private int[] itemOnShelfList []; private int size; public InventoryOnShelf() { }arrow_forwardDo not remove this question theirs no reason to. It follows all the guidelines. If you need more information just ask. Code in Java and post the entire finished program with UML and algorithm.Create and implement a set of several classes (nine minimum) that define various types of vehicles in an auto dealership’s own inventory displaying Inheritance and Polymorphism. Please include data values that describe various attributes belonging to each vehicle like the year, make, and model. Also make a main driver class that instantiates and exercises several of the classes. Include pseudocode for each line. Include an algorithm and a simple UML diagram and write in your pseudocode specifically where the algorithm is. The output should include all the given information, make, model, year and an explenation of what the program does.arrow_forward
- Java allows for methods to be chained together. Consider the following message from the captain of a pirate ship: String msg1 = " Maroon the First Mate with a flagon of water and a pistol! "; We want to change the message to read the message msg1: String msg2= “Maroon the Quartermaster with a flagon of water.” Three changes need to be made to adjust the string as desired: Trim the leading and trailing whitespace. Replace the substring First Mate with Quartermaster. Remove "and a pistol!" Add a period at the end of the sentence. A “chaining1” method which will apply in sequence 4 operations to perform the above. We will use the trim, replace, and substring methods, in this order. Thus the chaining1 method will receive a string msg1 and return a string msg2. Make sure msg2 is printed. A “chaining2” method which will apply the 4 operations above in one single statement. Thus the chaining2 method will receive a string msg1 and return as string msg2. Make sure msg2 is printedarrow_forwardIn this question, we will bake a class. Create a class Cake , with the following instance attributes: • name (a string) • ingreds (a list of strings) • price (a float) The Cake class has also the following methods: • Write a constructor that takes as input a string indicating the cake name, and a list of strings indicating the cake ingredients. (No docstring needed.) The constructor uses these values to initialize the attributes accordingly. Note that each cake should be assigned a random price between $10.00 (inclusive) and $15.00 (exclusive). You may import random . Moreover, a cake must contain at least three or more ingredients. If this is not the case, the constructor should raise a ValueError . For this constructor, do not worry about making a copy of the input array. You can simply copy the reference received as input into the corresponding attribute. 1 • Write a method (including docstring) called __str__ which returns a string containing the name of the cake and its price…arrow_forwardAll vehicles used for transportation in the U.S. must have identification, which varies according to the type of vehicle. For example, all automobiles have a unique Vehicle Identification Number (VIN) assigned by the manufacturer, plus a license plate number assigend by the state in which the auto is registerd. Your task is to modify the Auto class below to override the equals method of the Vehicle class to test that the VIN and license plate number are identical.arrow_forward
- Solve using OOP in Java.arrow_forwardIn Java, please. Complete the Course class by implementing the findStudentHighestGpa() method, which returns the Student object with the highest GPA in the course. Assume that no two students have the same highest GPA. Given classes: Class LabProgram contains the main method for testing the program. Class Course represents a course, which contains an ArrayList of Student objects as a course roster. (Type your code in here.) Class Student represents a classroom student, which has three fields: first name, last name, and GPA. (Hint: getGPA() returns a student's GPA.) Note: For testing purposes, different student values will be used. Ex. For the following students: Henry Nguyen 3.5 Brenda Stern 2.0 Lynda Robison 3.2 Sonya King 3.9 the output is: Top student: Sonya King (GPA: 3.9) LabProgram.java import java.util.Scanner; public class LabProgram { public static void main(String args[]) { Scanner scnr = new Scanner(System.in); Course course = new Course(); int…arrow_forwardPlease use java only. In this assignment, you will implement a simple game in a class called SimpleGame. This game has 2 options for the user playing. Based on user input, the user can choose to either convert time, from seconds to hours, minutes, and seconds, or calculate the sum of all digits in an integer. At the beginning of the game, the user will be prompted to input either 1 or 2, to indicate which option of the game they want to play. 1 will indicate converting time, and 2 will indicate calculating the sum of digits in an integer. For converting time, the user will be prompted to input a number of seconds (as an int) and the program will call a method that will convert the seconds to time, in the format hours:minutes:seconds, and print the result. For example, if the user enters 6734, the program will print the time, 1:52:14. As another example, if the user enters 10,000, the program should print 2:46:39. For calculating the sum of digits in an integer, the user will be…arrow_forward
- IN JAVA. Any help is appreciated! Thank you! PART 1 : Automobiles Create a data class named Automobile that implements the Comparable interface. Give the class data fields for make, model, year, and price. Then add a constructor, all getters, a toString method that shows all attribute values, and implement Comparable by using the year as the criterion for comparing instances. Write a program named TestAutos that creates an ArrayList of five or six Automobiles. Use a for loop to display the elements in the ArrayList. Sort the Arraylist of autos by year with Collections.sort(). Finally, use a foreach loop to display the ArrayList sorted by year.arrow_forwardFirst, you need to design, code in Java, test and document a base class, Student. The Student class will have the following information, and all of these should be defined as Private: A first name (given name) A last name (family name/surname) Student number (ID) – an integer number (of type long) The Student class will have at least the following constructors and methods: (i) two constructors - one without any parameters (the default constructor), and one with parameters to give initial values to all the instance variables of Student. (ii) only necessary set and get methods for a valid class design. (iii) a reportGrade method, which you have nothing to report here, you can just print to the screen a message “There is no grade here.”. This method will be overridden in the respective child classes. (iv) an equals method which compares two student objects and returns true if they have the same student number (ID), otherwise it returns false. You may add other…arrow_forwardFirst, you need to design, code in Java, test and document a base class, Student. The Student class will have the following information: A. Title of the student (eg Mr, Miss, Ms, Mrs etc)B. A first name (given name)C. A last name (family name/surname)D. Student number (ID) – an integer number (of type long)E. A date of birth (in day/month/year format – three ints) - (Do NOT use the Date class from JAVA)The student class will have at least the following constructors and methods:(i) two constructors - one without any parameters (the default constructor), and one with parameters to give initial values to all the instance variables.(ii) a reasonable number of set and get methods.(iii) methods to compute the final overall mark and the final grade (which will be overridden in the respective child classes). These two methods will be void methods that set the appropriate instance variables. Remember one method can call another method. If you prefer, you can define a single method that sets…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
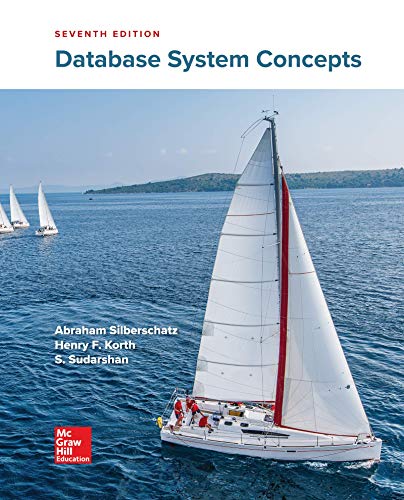
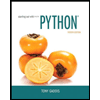
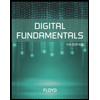
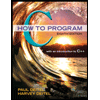
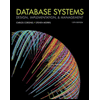
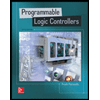