I am having trouble on removing the largest and smallest numbers and getting the sum and dividing it by
Following the instructions presented in the Lecture 4.5 and 4.6, complete the class Bag we discussed in the lectures. It should include all the data members, constructors, as well as all the member functions we have discussed. To be more specific, the class should have an interface shown as following:
class Bag
{
private:
int items[100];
int itemCount;
public:
Bag();
int getItemCount();
bool add(int newItem);
void display();
bool contains();
bool remove(int a);
int getSum();
};
Please complete all the member functions listed above including the default constructor.
Then add following member function to the class:
getMax()
This function returns the largest integer stored in the Bag without changing the position of each integer stored in the Bag.
Write a C++ program in a .cpp file (source file) that include above class Bag and perform following tasks:
Use function rand() to randomly generate 80 integers between 0 and 99 and put them into an object of the class Bag.
Use the member functions in the class Bag, such as getMax(), getMin(), and so on to
find the largest number and the smallest number in the Bag
display the indices of above those two numbers in the Bag
remove one largest number and one smallest number from the Bag.
find the sum of the remaining numbers and divide it by 78, and then display the result on the screen.
===============================================================================================
You will need to upload two files for this assignment:
The header file (.h file) that contains the required class Bag.
The source file (.cpp file) of your program specified as above.
Note: The class Bag should be created exactly the way we discussed in the class lecture 4.5 and 4.6. Otherwise, the assignment submitted will not be credited.
this is what I have so far
//class Bag
#ifndef Bag_h
#define Bag_h
#include<iostream>
using namespace std;
class Bag
{
private:
int items[100];
int itemCount;
public:
Bag();
int getItemCount();
void add(int newItem);
void display();
bool contains(int anItem);
bool remove(int anItem);
int getSum();
int getMax();
int getMin();
};
//Class Implementation
Bag::Bag()
{
itemCount = 0;
}
int Bag::getItemCount()
{
return itemCount;
}
void Bag::add(int newItem)
{
items[itemCount];
itemCount++;
}
void Bag::display()
{
cout << "The bag contains following integers:" << endl;
for (int i = 0; i < itemCount; i++) {
cout << items[i] << endl;
}
}
bool Bag::contains(int anItem)
{
for (int i = 0; i < itemCount; i++) {
if (items[i] == anItem)
return true;
}
return false;
}
bool Bag::remove(int anItem)
{
if (itemCount == 0) {
return false;
cout << "Item is not in the bag, removal failed. \n";
}
else {
int index = 0;
for (int i = 0; i < itemCount; i++) {
if (items[i] == anItem) {
index = i;
for (int k = index; k < itemCount - 1; k++) {
items[k] = items[k + 1];
}
itemCount--;
return true;
}
}
cout << "Item is not in the bag, removal failed. \n";
return false;
}
}
int Bag::getSum()
{
int s = 0;
for (int i = 0; i < itemCount; i++)
s += items[i];
return s;
}
int Bag::getMax()
{
int max = items[0];
for (int i = 0; i < itemCount; i++)
{
if (max < items[i])
{
max = items[i];
}
}
return max;
}
int Bag::getMin()
{
int min = items[0];
for (int i = 0; i < itemCount; i++)
{
if (min > items[i])
{
min = items[i];
}
}
return min;
}
#endif Bag_h
I am having trouble on removing the largest and smallest numbers and getting the sum and dividing it by 78

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 11 images

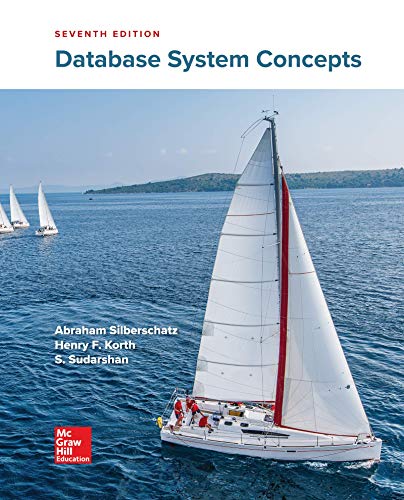
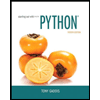
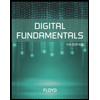
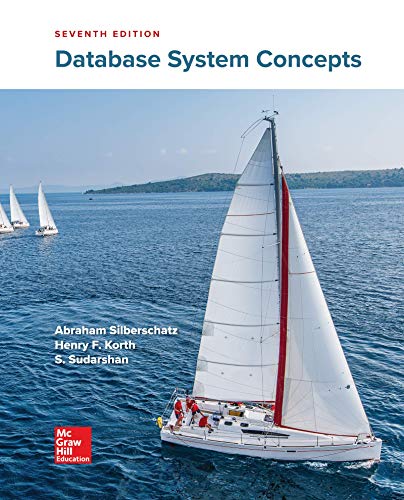
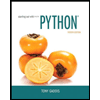
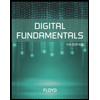
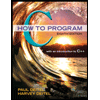
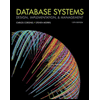
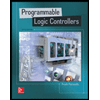