The Fraction Class – Immutable Classes In this section, we’ll build another Fraction class that is unchangeable once initialized and uses the keyword final for its numerator and denominator. Such a Fraction object will have all of its data declared final, and is our first example of building an immutable class. Once a Fraction object is built, its data items will never change for the lifetime of the object. Another way to view this is that the object is completely read-only. As a result, its data will also be declared public, which is the only example of public data you’ll find in this quarter. If you wish to change a fraction object’s numerator or denominator, the old object must be discarded and a new object created in its place. Again, this object’s data will be immutable, constant, non-variable, unchangeable, non-editable, or read-only. So, to add two fractions, our add function will return a new Fraction object that is the sum of the two previous (unchangeable) fractions we wish to add. Once you’ve built this class, uncomment out the appropriate tests in ClassDesignIIDriver.java. Start by building a new Fraction class, and define the following members: Class Invariants Numerators and denominators are unchangeable once set by the constructor. No denominator will be stored as a 0. (i.e., no DivideByZero Exceptions). A Fraction is always in reduced form (reduce in the constructor to ensure this). Data Define a numerator that is public and final. Why don’t we make this data private? Define a denominator that is public and final. What data types should these items be? Methods Define a constructor that takes a numerator and a denominator Do not define a no-argument constructor. Why? Define a constructor that takes a Fraction object and makes a copy of it. public Fraction(Fraction other){...} Define a toString() function as we’ve done for other classes. Define an add function that takes a fraction, adds it to this, then returns a new Fraction object that is the result of the addition of the two public Fraction add(Fraction that) {//add this and that together; remember to consider the denominator here! Define an equals(Object o) function that has the form: public boolean equals(Object other) { if( other != null && ! (other instanceof Fraction ) ) return false; //what does this code do? Fraction that = (Fraction) other; //and this code? //todo: code goes here }
The Fraction Class – Immutable Classes
In this section, we’ll build another Fraction class that is unchangeable once initialized and uses the keyword final for its numerator and denominator. Such a Fraction object will have all of its data declared final, and is our first example of building an immutable class. Once a Fraction object is built, its data items will never change for the lifetime of the object. Another way to view this is that the object is completely read-only. As a result, its data will also be declared public, which is the only example of public data you’ll find in this quarter. If you wish to change a fraction object’s numerator or denominator, the old object must be discarded and a new object created in its place. Again, this object’s data will be immutable, constant, non-variable, unchangeable, non-editable, or read-only. So, to add two fractions, our add function will return a new Fraction object that is the sum of the two previous (unchangeable) fractions we wish to add. Once you’ve built this class, uncomment out the appropriate tests in ClassDesignIIDriver.java. Start by building a new Fraction class, and define the following members:
Class Invariants
- Numerators and denominators are unchangeable once set by the constructor.
- No denominator will be stored as a 0. (i.e., no DivideByZero Exceptions).
- A Fraction is always in reduced form (reduce in the constructor to ensure this).
Data
- Define a numerator that is public and final.
- Why don’t we make this data private?
- Define a denominator that is public and final.
- What data types should these items be?
Methods
- Define a constructor that takes a numerator and a denominator
- Do not define a no-argument constructor. Why?
- Define a constructor that takes a Fraction object and makes a copy of it.
- public Fraction(Fraction other){...}
- Define a toString() function as we’ve done for other classes.
- Define an add function that takes a fraction, adds it to this, then returns a new Fraction object that is the result of the addition of the two
- public Fraction add(Fraction that) {//add this and that together; remember to consider the denominator here!
- Define an equals(Object o) function that has the form:
public boolean equals(Object other) {
if( other != null && ! (other instanceof Fraction ) ) return false; //what does this code do?
Fraction that = (Fraction) other; //and this code? //todo: code goes here }
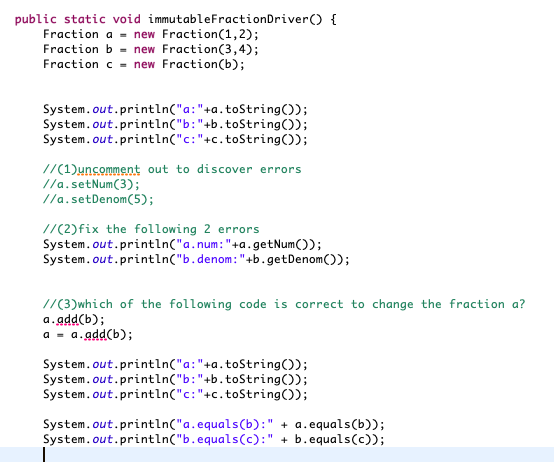

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 7 images
