I am trying to write a program for a bowling score in C++ Here is what I have so far: #include int main() { const int numFrames = 10; //The number of frames is 10 const int numThrows = 21; //The number of throws is 21 int throws[numThrows]; //Input for when the number of throws is <21 int frameScores[numFrames]; //Frame scores for the frames /*Computes a score for a different amount of throws in case the number of throws is less than 21 */ for (int i = 0; i < numThrows; ++i) { //std::cout << "Please enter score for throw: " << std::endl; std::cin >> throws[i]; } /* Calculates the bowling scores for the first 9 frames */ int throwIndex = 0; for (int frame = 0; frame < 9; ++frame) { int frameScore = throws[throwIndex]; if (frameScore == 10) { //For a strike frameScore += throws[throwIndex + 1] + throws[throwIndex + 2]; ++throwIndex; } else { frameScore += throws[throwIndex + 1]; if (frameScore == 10) { //For a spare frameScore += throws[throwIndex + 2]; } throwIndex += 2; } frameScores[frame] = frameScore; } /*Calculates the bowling scores for the 10th frame */ int frameScore = throws[throwIndex] + throws[throwIndex + 1]; if (frameScore >= 10) { //For a spare or strike frameScore += throws[throwIndex + 2]; } frameScores[9] = frameScore; //Outputs the scores for all frames in game for (int frame = 0; frame < numFrames; ++frame) { std::cout << frameScores[frame]; if (frame < numFrames -1) { std::cout << " "; } } std::cout << std::endl; return 0; }
I am trying to write a program for a bowling score in C++
Here is what I have so far:
#include <iostream>
int main() {
const int numFrames = 10; //The number of frames is 10
const int numThrows = 21; //The number of throws is 21
int throws[numThrows]; //Input for when the number of throws is <21
int frameScores[numFrames]; //Frame scores for the frames
/*Computes a score for a different amount of throws in case the
number of throws is less than 21 */
for (int i = 0; i < numThrows; ++i) {
//std::cout << "Please enter score for throw: " << std::endl;
std::cin >> throws[i];
}
/* Calculates the bowling scores for the first 9 frames */
int throwIndex = 0;
for (int frame = 0; frame < 9; ++frame) {
int frameScore = throws[throwIndex];
if (frameScore == 10) { //For a strike
frameScore += throws[throwIndex + 1] + throws[throwIndex + 2];
++throwIndex;
}
else {
frameScore += throws[throwIndex + 1];
if (frameScore == 10) { //For a spare
frameScore += throws[throwIndex + 2];
}
throwIndex += 2;
}
frameScores[frame] = frameScore;
}
/*Calculates the bowling scores for the 10th frame */
int frameScore = throws[throwIndex] + throws[throwIndex + 1];
if (frameScore >= 10) { //For a spare or strike
frameScore += throws[throwIndex + 2];
}
frameScores[9] = frameScore;
//Outputs the scores for all frames in game
for (int frame = 0; frame < numFrames; ++frame) {
std::cout << frameScores[frame];
if (frame < numFrames -1) {
std::cout << " ";
}
}
std::cout << std::endl;
return 0;
}



Step by step
Solved in 4 steps with 2 images

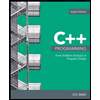
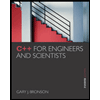
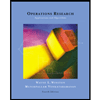
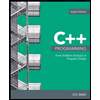
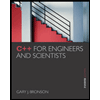
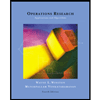