I have a code (class Server). I need help to fix error in another class. These are 2 seperated files. import socket import pandas as pd import sqlite3 class Server: def __init__(self, portnumber): self.port = portnumber self.s = socket.socket() self.host = socket.gethostname() self.s.bind((self.host, self.port)) self.s.listen(5) print('Server listening....') def __enter__(self): return self def __exit__(self, exc_type, exc_val, exc_tb): self.close() def serve(self): while True: conn, addr = self.s.accept() print('Got connection from', addr) query = conn.recv(1024).decode() print('Server received query:', query) # Process the SQL query and retrieve data data = self.process_query(query) # Send the data back to the client conn.sendall(data.to_msgpack()) print('Sent data:', data) conn.close() def process_query(self, query): # Connect to the SQLDatabase and execute the query connection = sqlite3.connect('data.db') data = pd.read_sql_query(query, connection) connection.close() return data def close(self): self.s.close() # Run the server with Server(5000) as server: server.serve() _______________________________ # Y Nhi Tran # Exercise Client import socket import pickle class Client: def __init__(self, portnumber): self.port = portnumber self.s = socket.socket() self.host = socket.gethostname() def connect(self): self.s.connect((self.host, self.port)) def send_query(self, query): self.s.send(query.encode()) def receive_dataframe(self): # Receive the data size size_data = b"" while len(size_data) < 4: chunk = self.s.recv(4 - len(size_data)) if not chunk: break size_data += chunk data_size = int.from_bytes(size_data, "big") # Receive the data data = b"" while len(data) < data_size: chunk = self.s.recv(min(data_size - len(data), 4096)) if not chunk: break data += chunk dataframe = pickle.loads(data) return dataframe def close(self): self.s.close() def __str__(self): return f"Client(port={self.port}, host={self.host})" def __repr__(self): return f"Client(port={self.port}, host={self.host})" def __enter__(self): self.connect() return self def __exit__(self, exc_type, exc_val, exc_tb): self.close() client = Client(5000) client.connect() query = "SELECT * FROM table_name" client.send_query(query) dataframe = client.receive_dataframe() print(dataframe) client.close() It says: dataframe = pickle.loads(data) EOFError: Ran out of input Please help me fix it. Change anything you need (in both files).
I have a code (class Server). I need help to fix error in another class. These are 2 seperated files. import socket import pandas as pd import sqlite3 class Server: def __init__(self, portnumber): self.port = portnumber self.s = socket.socket() self.host = socket.gethostname() self.s.bind((self.host, self.port)) self.s.listen(5) print('Server listening....') def __enter__(self): return self def __exit__(self, exc_type, exc_val, exc_tb): self.close() def serve(self): while True: conn, addr = self.s.accept() print('Got connection from', addr) query = conn.recv(1024).decode() print('Server received query:', query) # Process the SQL query and retrieve data data = self.process_query(query) # Send the data back to the client conn.sendall(data.to_msgpack()) print('Sent data:', data) conn.close() def process_query(self, query): # Connect to the SQLDatabase and execute the query connection = sqlite3.connect('data.db') data = pd.read_sql_query(query, connection) connection.close() return data def close(self): self.s.close() # Run the server with Server(5000) as server: server.serve() _______________________________ # Y Nhi Tran # Exercise Client import socket import pickle class Client: def __init__(self, portnumber): self.port = portnumber self.s = socket.socket() self.host = socket.gethostname() def connect(self): self.s.connect((self.host, self.port)) def send_query(self, query): self.s.send(query.encode()) def receive_dataframe(self): # Receive the data size size_data = b"" while len(size_data) < 4: chunk = self.s.recv(4 - len(size_data)) if not chunk: break size_data += chunk data_size = int.from_bytes(size_data, "big") # Receive the data data = b"" while len(data) < data_size: chunk = self.s.recv(min(data_size - len(data), 4096)) if not chunk: break data += chunk dataframe = pickle.loads(data) return dataframe def close(self): self.s.close() def __str__(self): return f"Client(port={self.port}, host={self.host})" def __repr__(self): return f"Client(port={self.port}, host={self.host})" def __enter__(self): self.connect() return self def __exit__(self, exc_type, exc_val, exc_tb): self.close() client = Client(5000) client.connect() query = "SELECT * FROM table_name" client.send_query(query) dataframe = client.receive_dataframe() print(dataframe) client.close() It says: dataframe = pickle.loads(data) EOFError: Ran out of input Please help me fix it. Change anything you need (in both files).
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
I have a code (class Server). I need help to fix error in another class. These are 2 seperated files.
import socket
import pandas as pd
import sqlite3
class Server:
def __init__(self, portnumber):
self.port = portnumber
self.s = socket.socket()
self.host = socket.gethostname()
self.s.bind((self.host, self.port))
self.s.listen(5)
print('Server listening....')
def __enter__(self):
return self
def __exit__(self, exc_type, exc_val, exc_tb):
self.close()
def serve(self):
while True:
conn, addr = self.s.accept()
print('Got connection from', addr)
query = conn.recv(1024).decode()
print('Server received query:', query)
# Process the SQL query and retrieve data
data = self.process_query(query)
# Send the data back to the client
conn.sendall(data.to_msgpack())
print('Sent data:', data)
conn.close()
def process_query(self, query):
# Connect to the SQLDatabase and execute the query
connection = sqlite3.connect('data.db')
data = pd.read_sql_query(query, connection)
connection.close()
return data
def close(self):
self.s.close()
# Run the server
with Server(5000) as server:
server.serve()
import pandas as pd
import sqlite3
class Server:
def __init__(self, portnumber):
self.port = portnumber
self.s = socket.socket()
self.host = socket.gethostname()
self.s.bind((self.host, self.port))
self.s.listen(5)
print('Server listening....')
def __enter__(self):
return self
def __exit__(self, exc_type, exc_val, exc_tb):
self.close()
def serve(self):
while True:
conn, addr = self.s.accept()
print('Got connection from', addr)
query = conn.recv(1024).decode()
print('Server received query:', query)
# Process the SQL query and retrieve data
data = self.process_query(query)
# Send the data back to the client
conn.sendall(data.to_msgpack())
print('Sent data:', data)
conn.close()
def process_query(self, query):
# Connect to the SQLDatabase and execute the query
connection = sqlite3.connect('data.db')
data = pd.read_sql_query(query, connection)
connection.close()
return data
def close(self):
self.s.close()
# Run the server
with Server(5000) as server:
server.serve()
_______________________________
# Y Nhi Tran
# Exercise Client
import socket
import pickle
class Client:
def __init__(self, portnumber):
self.port = portnumber
self.s = socket.socket()
self.host = socket.gethostname()
def connect(self):
self.s.connect((self.host, self.port))
def send_query(self, query):
self.s.send(query.encode())
def receive_dataframe(self):
# Receive the data size
size_data = b""
while len(size_data) < 4:
chunk = self.s.recv(4 - len(size_data))
if not chunk:
break
size_data += chunk
data_size = int.from_bytes(size_data, "big")
# Receive the data
data = b""
while len(data) < data_size:
chunk = self.s.recv(min(data_size - len(data), 4096))
if not chunk:
break
data += chunk
dataframe = pickle.loads(data)
return dataframe
def close(self):
self.s.close()
def __str__(self):
return f"Client(port={self.port}, host={self.host})"
def __repr__(self):
return f"Client(port={self.port}, host={self.host})"
def __enter__(self):
self.connect()
return self
def __exit__(self, exc_type, exc_val, exc_tb):
self.close()
client = Client(5000)
client.connect()
query = "SELECT * FROM table_name"
client.send_query(query)
dataframe = client.receive_dataframe()
print(dataframe)
client.close()
# Exercise Client
import socket
import pickle
class Client:
def __init__(self, portnumber):
self.port = portnumber
self.s = socket.socket()
self.host = socket.gethostname()
def connect(self):
self.s.connect((self.host, self.port))
def send_query(self, query):
self.s.send(query.encode())
def receive_dataframe(self):
# Receive the data size
size_data = b""
while len(size_data) < 4:
chunk = self.s.recv(4 - len(size_data))
if not chunk:
break
size_data += chunk
data_size = int.from_bytes(size_data, "big")
# Receive the data
data = b""
while len(data) < data_size:
chunk = self.s.recv(min(data_size - len(data), 4096))
if not chunk:
break
data += chunk
dataframe = pickle.loads(data)
return dataframe
def close(self):
self.s.close()
def __str__(self):
return f"Client(port={self.port}, host={self.host})"
def __repr__(self):
return f"Client(port={self.port}, host={self.host})"
def __enter__(self):
self.connect()
return self
def __exit__(self, exc_type, exc_val, exc_tb):
self.close()
client = Client(5000)
client.connect()
query = "SELECT * FROM table_name"
client.send_query(query)
dataframe = client.receive_dataframe()
print(dataframe)
client.close()
It says: dataframe = pickle.loads(data)
EOFError: Ran out of input
EOFError: Ran out of input
Please help me fix it. Change anything you need (in both files).
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
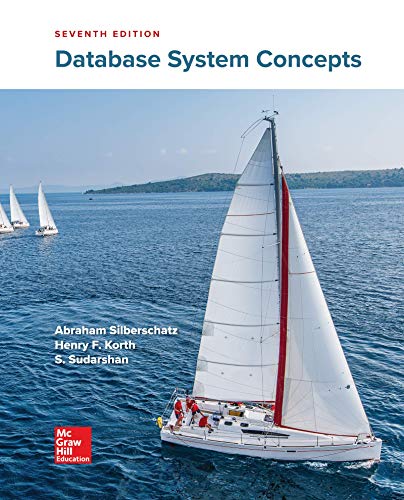
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
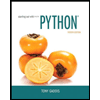
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
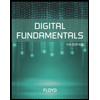
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
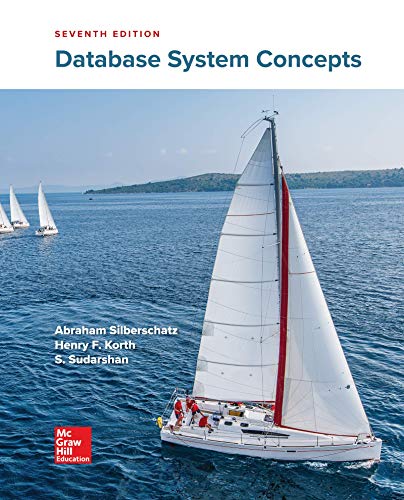
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
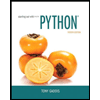
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
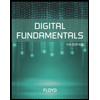
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
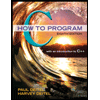
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
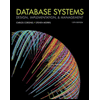
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
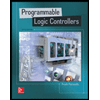
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education