I have my code below and I am stuck at the last part which is the display of the thread that finished first and last. There are times when it give the correct output and sometimes it is not. How can it be fixed? The sample output is given below and the output I made. import java.util.Random; import java.util.Scanner; class prog1 implements Runnable { private String cName = new String(""); private int x; public int c1 = 0, c2 = 0, c3 = 0; // static array to store which thread closed first public static int [] order = new int[3]; // index of array private static int index = 0; public prog1 (String cName, int x){ this.cName = cName; this.x = x; } @Override public void run(){ Random r = new Random(); int randNum; for (int i = 1; i <= this.x; i++){ randNum = r.nextInt(x) + 1; if(i == this.x) { // put the number // get number from name's last character of thread order[index++] = cName.charAt(cName.length() -1) - '0'; System.out.println(" Voter " + i + " " + cName + " = " + randNum + "[Voting is closed]"); } else System.out.println(" Voter " + i + " " + cName + " = " + randNum); if(randNum == 1) c1++; if(randNum == 2) c2++; if(randNum == 3) c3++; } } } public class Quiz2 { public static void main(String args[]){ Scanner sc = new Scanner(System.in); int c1 = 0, c2 = 0, c3 = 0; for (int x = 0; x < args.length; x++){ System.out.println(args[x]); } System.out.print("Enter number of voters: "); int input = sc.nextInt(); prog1 r1 = new prog1("Thread #1", input); Thread Candidate1 = new Thread(r1); Candidate1.start(); prog1 r2 = new prog1("Thread #2", input); Thread Candidate2 = new Thread(r2); Candidate2.start(); prog1 r3 = new prog1("Thread #3", input); Thread Candidate3 = new Thread(r3); Candidate3.start(); try { Candidate1.join(); Candidate2.join(); Candidate3.join(); } catch (InterruptedException e) { e.printStackTrace(); } c1 = r1.c1 + r2.c1 + r3.c1; c2 = r1.c2 + r2.c2 + r3.c2; c3 = r3.c3 + r2.c3 + r3.c3; System.out.println("\nCandidate #1 has " + c1 + " vote(s)."); System.out.println("Candidate #2 has " + c2 + " vote(s)."); System.out.println("Candidate #3 has " + c3 + " vote(s)."); // if each candidate get same number of votes if(c1 == c2 && c2 == c3){ System.out.println("No Winner"); } else if(c1 > c2) { if(c1 > c3) { System.out.println("\nCandidate #1 wins!!!\n"); } else { System.out.println("\nCandidate #3 wins!!!\n"); } } else { if(c2 > c3) { System.out.println("\nCandidate #2 wins!!!\n"); } else { System.out.println("\nCandidate #3 wins!!!\n"); } } // print the order which thread closed System.out.println("Thread #"+r1.order[0]+" Finished 1st"); System.out.println("Thread #"+r1.order[2] +" Finished last"); sc.close(); } }
I have my code below and I am stuck at the last part which is the display of the thread that finished first and last. There are times when it give the correct output and sometimes it is not. How can it be fixed? The sample output is given below and the output I made.
import java.util.Random;
import java.util.Scanner;
class prog1 implements Runnable {
private String cName = new String("");
private int x;
public int c1 = 0, c2 = 0, c3 = 0;
// static array to store which thread closed first
public static int [] order = new int[3];
// index of array
private static int index = 0;
public prog1 (String cName, int x){
this.cName = cName;
this.x = x;
}
@Override
public void run(){
Random r = new Random();
int randNum;
for (int i = 1; i <= this.x; i++){
randNum = r.nextInt(x) + 1;
if(i == this.x) {
// put the number
// get number from name's last character of thread
order[index++] = cName.charAt(cName.length() -1) - '0';
System.out.println(" Voter " + i + " " + cName + " = " + randNum + "[Voting is closed]");
} else
System.out.println(" Voter " + i + " " + cName + " = " + randNum);
if(randNum == 1)
c1++;
if(randNum == 2)
c2++;
if(randNum == 3)
c3++;
}
}
}
public class Quiz2 {
public static void main(String args[]){
Scanner sc = new Scanner(System.in);
int c1 = 0, c2 = 0, c3 = 0;
for (int x = 0; x < args.length; x++){
System.out.println(args[x]);
}
System.out.print("Enter number of voters: ");
int input = sc.nextInt();
prog1 r1 = new prog1("Thread #1", input);
Thread Candidate1 = new Thread(r1);
Candidate1.start();
prog1 r2 = new prog1("Thread #2", input);
Thread Candidate2 = new Thread(r2);
Candidate2.start();
prog1 r3 = new prog1("Thread #3", input);
Thread Candidate3 = new Thread(r3);
Candidate3.start();
try {
Candidate1.join();
Candidate2.join();
Candidate3.join();
} catch (InterruptedException e) {
e.printStackTrace();
}
c1 = r1.c1 + r2.c1 + r3.c1;
c2 = r1.c2 + r2.c2 + r3.c2;
c3 = r3.c3 + r2.c3 + r3.c3;
System.out.println("\nCandidate #1 has " + c1 + " vote(s).");
System.out.println("Candidate #2 has " + c2 + " vote(s).");
System.out.println("Candidate #3 has " + c3 + " vote(s).");
// if each candidate get same number of votes
if(c1 == c2 && c2 == c3){
System.out.println("No Winner");
}
else if(c1 > c2) {
if(c1 > c3) {
System.out.println("\nCandidate #1 wins!!!\n");
} else {
System.out.println("\nCandidate #3 wins!!!\n");
}
} else {
if(c2 > c3) {
System.out.println("\nCandidate #2 wins!!!\n");
} else {
System.out.println("\nCandidate #3 wins!!!\n");
}
}
// print the order which thread closed
System.out.println("Thread #"+r1.order[0]+" Finished 1st");
System.out.println("Thread #"+r1.order[2] +" Finished last");
sc.close();
}
}
![General Output
--Configuration: <Default>-
Enter number of voters: 4
Voter 1 Thread #1 = 1
Voter 2 Thread #1 = 1
Voter 3 Thread #1 = 4
Voter 1 Thread #3 = 3
Voter 2 Thread #3
Voter 3 Thread #3 = 3
3
Voter 1 Thread #2 = 2
Voter 2 Thread #2 1
Voter 3 Thread #2 = 3
Voter 4 Thread #3
2[Voting is closed]
2[ Voting is closed]
3[ Voting is closed]
%3D
Voter 4 Thread #1
Voter 4 Thread #2 =
Candidate #1 has 3 vote(s).
Candidate #2 has 3 vote(s).
Candidate #3 has 8 vote(s).
Candidate #3 wins!!!
Thread #1 Finished 1st
Thread #2 Finished last
Process completed.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fca2180eb-e455-463f-9a9e-dd04cac7e66f%2Fb3783634-172d-4255-b8e6-1de9920c8c78%2Fy6ix5ag_processed.jpeg&w=3840&q=75)
![Create a program that allOws users to enter the number of voters, then
generates 3 Threads.
Thread #1 will be named Candidate #1, Thread #2 as Candidate #2 and Thread #3 as
Candidate #3.
Each of the thread runs an iterative statement that generates "x" random numbers
between 1 to 3.
If the system generates a value of 1, that means a vote will be counted for
Candidate #1; if 2 vote will be added for Candidate #2 otherwise, it will be
credited to Candidate#3.
Note:
1. "x" (will be based on the "Number of voters")
2. If either of candidates have the same number of votes, then output text must say
"No Winner"
Sample output:
Enter # of voters: 3
Voter 1 Thread #1 = 1
Voter 2 Thread #1 = 3
Voter 1 Thread #3
2
%3D
Voter 1 Thread #2 = 1
Voter 2 Thread #2
= 3
Voter 3 Thread #1 = 1 [Voting closed]
Voter 2 Thread #3 = 2
1 [Voting closed]
Voter 3 Thread #3 = 2 [Voting closed]
Voter 3 Thread #2
Candidate #1 has 4 vote(s)
Candidate #2 has 3 vote(s)
Candidate #3 has 2 vote(s)
Candidate #1 Wins!!!
Thread #1 Finished 1st
Thread #3 Finished last](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fca2180eb-e455-463f-9a9e-dd04cac7e66f%2Fb3783634-172d-4255-b8e6-1de9920c8c78%2Fm2lqh5_processed.jpeg&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

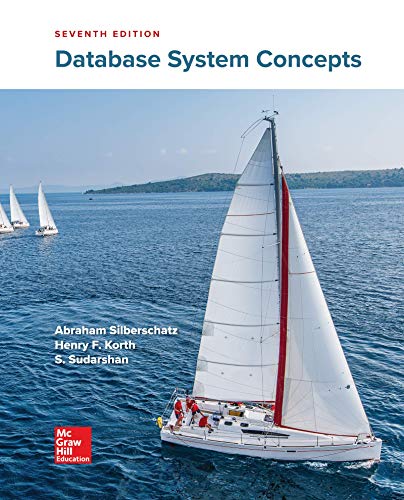
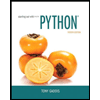
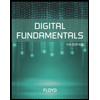
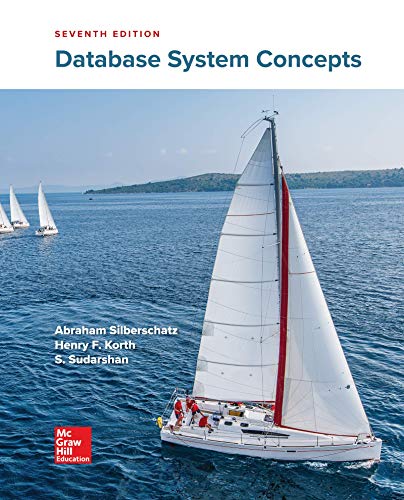
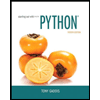
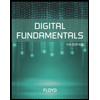
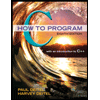
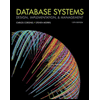
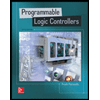