creenshot needed, just explain the program and answer the red question to copy program /* Multi-threaded example */ #include #include #include #define NUM_THREADS 10 /*This data is shared by the thread(s) */ pthread_t tid[NUM_THREADS]; /*This is the thread function */ void *runner(void *param); int main(int argc, char *argv[]) { int i; pthread_attr_t attr;
No screenshot needed, just explain the
to copy program
/* Multi-threaded example */
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define NUM_THREADS 10
/*This data is shared by the thread(s) */ pthread_t tid[NUM_THREADS];
/*This is the thread function */ void *runner(void *param);
int main(int argc, char *argv[]) {
int i;
pthread_attr_t attr;
printf("I am the parent thread\n");
/* get the default attributes */ pthread_attr_init(&attr);
/* set the scheduling
/* set the scheduling policy - FIFO, RR, or OTHER */
pthread_attr_setschedpolicy(&attr, SCHED_OTHER);
/* create the threads */
for (i = 0; i < NUM_THREADS; i++) pthread_create(&tid[i], &attr, runner, (void *) i);
/* now join on each thread */
for (i = 0; i < NUM_THREADS; i++) pthread_join(tid[i], NULL);
printf("I am the parent thread again\n");
return 0;
}
/* Each thread will begin control in this function */
void *runner(void *param) {
int id; id = (int) param;
printf("I am thread #%d, My ID #%lu\n", id, tid[id]);
pthread_exit(0);
}
![3. The following example demonstrates a multi-threaded program:
7* Multi-threaded example /
Ainclude <stdio.h>
include <atdlib.h>
tinclude <pthread. h>
define NUM_THREADS 10
Thls data is shared by the threadis) /
pthread_t tid NUM THREADS):
"This is the thread function
void *runner (void *param) :
int maintint arge, char "argv[l)
Int i
pthread attr_t attr:
printf ("I an the parent thread\n"):
* get the default attribates
pthread attr_init(satte):
* set the scheduling algoritha to PROCESS (PCS) or SYSTEM (SCS)
pthread_attr_setscope (tattr, PTHREAD_SCOPE_SYSTEM) :
* set the scheduling poliey - FIro, RR, or OTHER /
pthread_attr_setschedpolicy (6attr, SCHED OTHER) :
create the threada
for (i- 07 i < NUM_THREADS: 1++)
pthread_create (stid(i1, sattr, runner, (void ) 1):
* now jain on each thread
for (i- 0: 1 < NUM_THREADS: 1++)
pthread join(tid(a), NULL) :
printf("I an the parent thread again\n"):
return 0:
1* Kach thread wiil begin control in thin funetion /
void "Eunner (void "param)1
int idi
id (intl param
printf("I an thread Fid, My ID Fluin". id, tid[id]):
pthread exit (0):
Run the above program several times and observe the outputs:
I am the parent thread
I am thread #4, My ID #3043994480
I am thread #5, My ID #3035601776
I am thread #6, My ID #3027209072
I am thread #3, My ID #3052387184
I am thread #2, My ID #3060779888
I am thread #7, My ID #3018816368
I am thread #8, My ID #3010423664
I am thread #9, My ID #3002030960
I am thread #1, My ID #3069172592
I am thread #0, My ID #3077565296
I am the parent thread again
Do the output lines come in the same order every time? Why?](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F58383a79-7136-42d5-96cb-b769297f304b%2F43994356-fd00-42f5-8863-fcc1f447dece%2Ff3lsqi6_processed.jpeg&w=3840&q=75)

Step by step
Solved in 2 steps

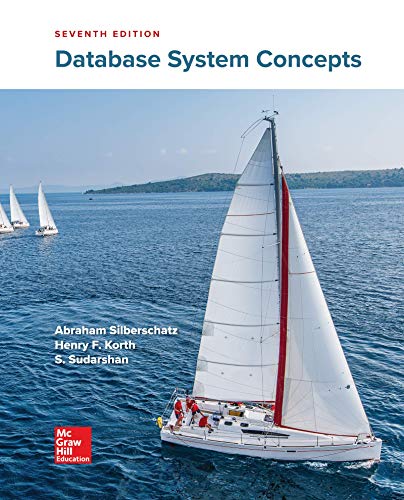
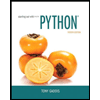
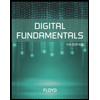
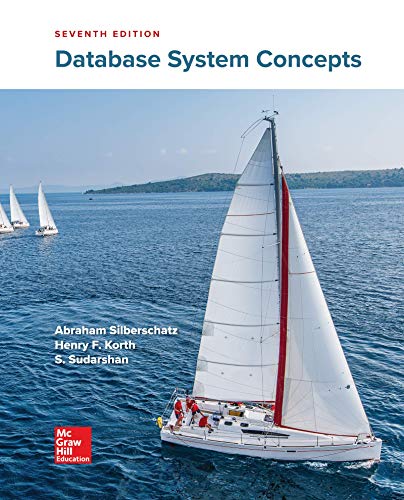
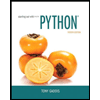
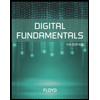
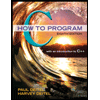
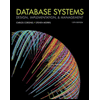
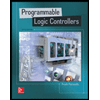