I have this code for word app API and I need to Create a client-side application that provides a user interface to manage multiple collections of words using the API developed earlier. 1. Create an HTML file under words-app/public/index.html. You can access and test this page using http://localhost:3000/index.html.
I have this code for word app API and I need to Create a client-side application that provides a user interface to manage multiple collections of words using the API developed earlier. 1. Create an HTML file under words-app/public/index.html. You can access and test this page using http://localhost:3000/index.html.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
I have this code for word app API
and I need to Create a client-side application that provides a user interface to manage multiple collections of words using the API developed earlier.
1. Create an HTML file under words-app/public/index.html. You can access and test this page using http://localhost:3000/index.html.
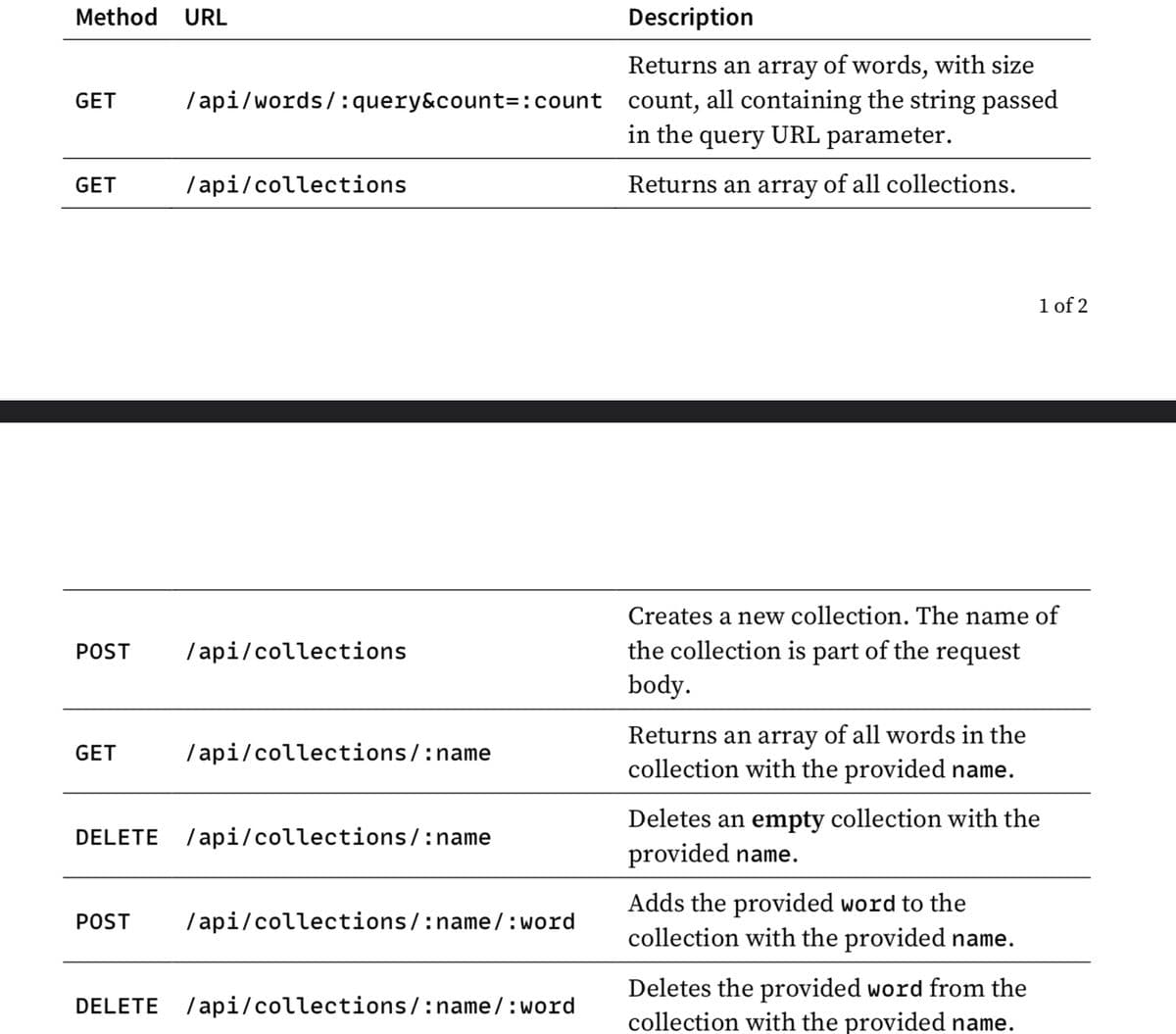
Transcribed Image Text:Method URL
GET
GET
POST
GET
Description
Returns an array of words, with size
/api/words/:query&count=:count count, all containing the string passed
in the query URL parameter.
Returns an array of all collections.
POST
DELETE
/api/collections
/api/collections
DELETE /api/collections/:name
/api/collections/:name
/api/collections/:name/:word
/api/collections/:name/:word
Creates a new collection. The name of
the collection is part of the request
body.
Returns an array of all words in the
collection with the provided name.
1 of 2
Deletes an empty collection with the
provided name.
Adds the provided word to the
collection with the provided name.
Deletes the provided word from the
collection with the provided name.
![1 // Import necessary modules and setup the Express app
2 const express = require("express");
3 const
fs = require("fs");
HN M & N
const app = express();
5 const wordsDataPath = "./words-app/data/words.json";
6 const collectionsDataPath = "./words-app/data/collections.json";
7 const PORT = process.env.PORT || 3000;
8
9
4
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26 // Endpoint to read all collections
27
28
29
30 res.send(collections);
31 });
32
33 // Endpoint to create a new collection
34 app.post("/api/collections", (req, res) => {
35 let collections = fs.readFileSync(collectionsDataPath);
36
collections = JSON.parse(collections);
37
let newCollectionName = req.body.name;
38 collections [newCollectionName] = [];
39 fs.writeFileSync (collectionsDataPath, JSON.stringify(collections));
40
res.sendStatus (200);
41
42
43 // Endpoint to get all words in a collection
44
app.get("/api/collections/:name", (req, res) => {
45 let collections = fs.readFileSync(collectionsDataPath);
46
collections = JSON.parse(collections);
47
let collectionName = req.params.name;
48 let collection = collections [collectionName];
49
res.send(collection);
50
51
52 // Endpoint to delete an empty collection
app.delete("/api/collections/:name", (req, res) => {
let collections = fs.readFileSync (collectionsDataPath);
collections = JSON.parse(collections);
let collectionName = req.params.name;
if (collections [collectionName].length
delete collections [collectionName];
fs.writeFileSync (collectionsDataPath, JSON.stringify(collections));
res.sendStatus (200);
53
54
55
56
57
58
59
60
61
u62 3 64 65 66印 686970123475 76 7
63
67
// Endpoint to search for a certain number of words using a query text
app.get("/api/words/:query", (req, res) => {
77
let query = req.params.query;
let count = req.query.count || 10;
count = count > 100 ? 100 count; // Limit to 100 words per request
let words = fs.readFileSync (wordsDataPath);
words = JSON.parse(words);
let matchingWords words.filter((word) => word.includes (query));
let selectedWords =
[];
for (let i = 0; i < count && matchingWords.length > 0; i++) {
let index = Math.floor(Math.random() * matchingWords.length);
selectedWords.push(matchingWords[index]);
}
res.send(selectedWords);
});
app.get("/api/collections", (req, res) => {
let collections = fs.readFileSync (collectionsDataPath);
collections = JSON.parse(collections);
});
matchingWords.splice(index, 1);
});
} else {
});
res.sendStatus (400);
collections [collectionName].push(word);
});
// Endpoint to add a word to a collection
app.post("/api/collections/:name/:word", (req, res) => {
let collections = fs.readFileSync(collectionsDataPath);
collections = JSON.parse(collections);
let collectionName = req.params.name;
let word = req.params.word;
fs.writeFileSync(collectionsDataPath, JSON.stringify(collections));
res.sendStatus (200);
// Endpoint to delete a word from a collection
let collectionName = req.params.name;
78 app.delete("/api/collections/:name/:word", (req, res) => {
79
let collections = fs.readFileSync(collectionsDataPath);
80 collections = JSON.parse(collections);
81
82 let word = req.params.word;
83
84
85
86
87
88
89
90
91
92
93
94
}
95 });
96
97 // Start the server
98 app.listen (PORT, () => {
99
100 });
101
===
);
0) {
let index = collections [collectionName].indexOf(word);
if (index > -1) {
collections [collectionName].splice(index, 1);
fs.writeFileSync (
collections DataPath,
JSON.stringify(collections)
res.sendStatus (200);
} else {
res.sendStatus (400);
console.log(`server listening on port ${PORT}`);](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fbbc8a687-c0f0-429c-86f1-046930399c86%2F2e33a1a6-78e9-47e9-a11e-8deef594909e%2Fnpyycol_processed.png&w=3840&q=75)
Transcribed Image Text:1 // Import necessary modules and setup the Express app
2 const express = require("express");
3 const
fs = require("fs");
HN M & N
const app = express();
5 const wordsDataPath = "./words-app/data/words.json";
6 const collectionsDataPath = "./words-app/data/collections.json";
7 const PORT = process.env.PORT || 3000;
8
9
4
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26 // Endpoint to read all collections
27
28
29
30 res.send(collections);
31 });
32
33 // Endpoint to create a new collection
34 app.post("/api/collections", (req, res) => {
35 let collections = fs.readFileSync(collectionsDataPath);
36
collections = JSON.parse(collections);
37
let newCollectionName = req.body.name;
38 collections [newCollectionName] = [];
39 fs.writeFileSync (collectionsDataPath, JSON.stringify(collections));
40
res.sendStatus (200);
41
42
43 // Endpoint to get all words in a collection
44
app.get("/api/collections/:name", (req, res) => {
45 let collections = fs.readFileSync(collectionsDataPath);
46
collections = JSON.parse(collections);
47
let collectionName = req.params.name;
48 let collection = collections [collectionName];
49
res.send(collection);
50
51
52 // Endpoint to delete an empty collection
app.delete("/api/collections/:name", (req, res) => {
let collections = fs.readFileSync (collectionsDataPath);
collections = JSON.parse(collections);
let collectionName = req.params.name;
if (collections [collectionName].length
delete collections [collectionName];
fs.writeFileSync (collectionsDataPath, JSON.stringify(collections));
res.sendStatus (200);
53
54
55
56
57
58
59
60
61
u62 3 64 65 66印 686970123475 76 7
63
67
// Endpoint to search for a certain number of words using a query text
app.get("/api/words/:query", (req, res) => {
77
let query = req.params.query;
let count = req.query.count || 10;
count = count > 100 ? 100 count; // Limit to 100 words per request
let words = fs.readFileSync (wordsDataPath);
words = JSON.parse(words);
let matchingWords words.filter((word) => word.includes (query));
let selectedWords =
[];
for (let i = 0; i < count && matchingWords.length > 0; i++) {
let index = Math.floor(Math.random() * matchingWords.length);
selectedWords.push(matchingWords[index]);
}
res.send(selectedWords);
});
app.get("/api/collections", (req, res) => {
let collections = fs.readFileSync (collectionsDataPath);
collections = JSON.parse(collections);
});
matchingWords.splice(index, 1);
});
} else {
});
res.sendStatus (400);
collections [collectionName].push(word);
});
// Endpoint to add a word to a collection
app.post("/api/collections/:name/:word", (req, res) => {
let collections = fs.readFileSync(collectionsDataPath);
collections = JSON.parse(collections);
let collectionName = req.params.name;
let word = req.params.word;
fs.writeFileSync(collectionsDataPath, JSON.stringify(collections));
res.sendStatus (200);
// Endpoint to delete a word from a collection
let collectionName = req.params.name;
78 app.delete("/api/collections/:name/:word", (req, res) => {
79
let collections = fs.readFileSync(collectionsDataPath);
80 collections = JSON.parse(collections);
81
82 let word = req.params.word;
83
84
85
86
87
88
89
90
91
92
93
94
}
95 });
96
97 // Start the server
98 app.listen (PORT, () => {
99
100 });
101
===
);
0) {
let index = collections [collectionName].indexOf(word);
if (index > -1) {
collections [collectionName].splice(index, 1);
fs.writeFileSync (
collections DataPath,
JSON.stringify(collections)
res.sendStatus (200);
} else {
res.sendStatus (400);
console.log(`server listening on port ${PORT}`);
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
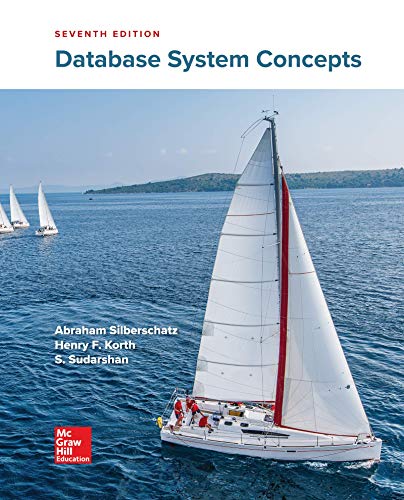
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
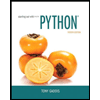
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
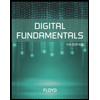
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
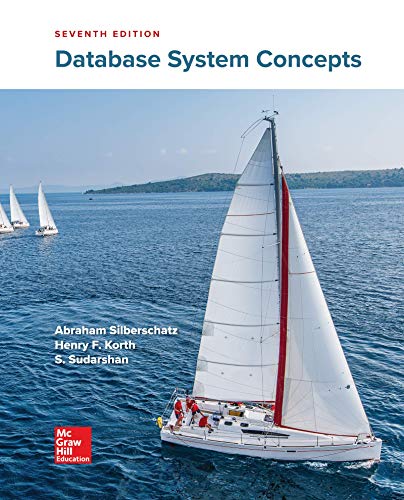
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
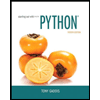
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
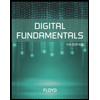
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
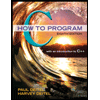
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
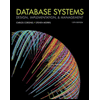
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
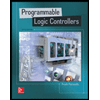
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education