//client.c #include "csapp.h" int main(int argc, char **argv) { int connfd; rio_t rio; connfd = Open_clientfd(argv[1], argv[2]); Rio_readinitb(&rio, connfd); char buffer[MAXLINE]; printf("Your balance is %s\n", buffer); Close(connfd); exit(0); int choice; float amount; int acc2; char buffer[MAXLINE]; rio_t rio; Rio_readinitb(&rio, connfd); while (1) { printf("\nHello welcome to the Bank Management System\n"); printf("----------------------\n"); printf("1. Check Balance\n"); printf("2. Deposit\n"); printf("3. Withdraw\n"); printf("4. Transfer\n"); printf("5. Quit\n"); printf("Enter your choice: "); Rio_writen(connfd, &choice, sizeof(int)); if (choice == 1) { printf("Your balance is %s\n", buffer); } else if (choice == 2) { printf("Enter amount to deposit: "); Rio_writen(connfd, &amount, sizeof(float)); printf("%s\n", buffer); } else if (choice == 3) { printf("Enter amount to withdraw: "); Rio_writen(connfd, &amount, sizeof(float)); printf("%s\n", buffer); } else if (choice == 4) { printf("Enter amount to transfer: "); printf("Enter recipient account number: "); Rio_writen(connfd, &amount, sizeof(float)); Rio_writen(connfd, &acc2, sizeof(int)); printf("%s\n", buffer); printf("Recipient's new balance is %s\n", buffer); } else if (choice == 5) { Close(connfd); printf("Connection Closed!!\n"); break; } } exit(0); } //server.c #include "csapp.h" void *thread(void *vargp); int main(int argc, char **argv) { int listenfd, *connfdp; socklen_t clientlen; struct sockaddr_storage clientaddr; pthread_t tid; listenfd = Open_listenfd(argv[1]); while (1) { clientlen = sizeof(struct sockaddr_storage); connfdp = Malloc(sizeof(int)); *connfdp = Accept(listenfd, (SA *)&clientaddr, &clientlen); Pthread_create(&tid, NULL, thread, connfdp); } } void *thread(void *vargp) { int connfd = *((int *)vargp); Pthread_detach(pthread_self()); Free(vargp); FILE *fp = fopen("bank.txt", "r"); char buf[MAXLINE]; int account_number, balance; char name[MAXLINE]; rio_t rio; while (fgets(buf, MAXLINE, fp) != NULL) { sscanf(buf, "%d %s %d", &account_number, name, &balance); } fclose(fp); sprintf(buf, "%d", balance); Rio_writen(connfd, buf, strlen(buf)); while (1) { if (Rio_readlineb(&rio, buf, MAXLINE) <= 0) { break; } int choice = buf[0] - '0'; if (choice == 1) { sprintf(buf, "%d\n", balance); Rio_writen(connfd, buf, strlen(buf)); } else if (choice == 2) { if (Rio_readlineb(&rio, buf, MAXLINE) <= 0) { break; } int deposit_amount = atoi(buf); balance += deposit_amount; sprintf(buf, "Deposit successful. New balance: %d\n", balance); Rio_writen(connfd, buf, strlen(buf)); } else if (choice == 3) { if (Rio_readlineb(&rio, buf, MAXLINE) <= 0) { break; } int withdrawal_amount = atoi(buf); if (balance >= withdrawal_amount) { balance -= withdrawal_amount; sprintf(buf, "Withdrawal successful. New balance: %d\n", balance); Rio_writen(connfd, buf, strlen(buf)); } } else if (choice == 4) { if (Rio_readlineb(&rio, buf, MAXLINE) <= 0) { break; } int transfer_amount = atoi(buf); if (Rio_readlineb(&rio, buf, MAXLINE) <= 0) { break; } int destination_account = atoi(buf); if (balance >= transfer_amount) { balance -= transfer_amount; sprintf(buf, "Transfer successful. New balance: %d\n", balance); Rio_writen(connfd, buf, strlen(buf)); } } else if (choice == 5) { Close(connfd); break; } } return NULL; } //bank.txt 100 John Smith 500.00 200 Jane Doe 1000.00 300 Luke Skylar 1500.00 Can you help me make a connection with the client and server and be able to read the text file?
//client.c
#include "csapp.h"
int main(int argc, char **argv)
{
int connfd;
rio_t rio;
connfd = Open_clientfd(argv[1], argv[2]);
Rio_readinitb(&rio, connfd);
char buffer[MAXLINE];
printf("Your balance is %s\n", buffer);
Close(connfd);
exit(0);
int choice;
float amount;
int acc2;
char buffer[MAXLINE];
rio_t rio;
Rio_readinitb(&rio, connfd);
while (1)
{
printf("\nHello welcome to the Bank Management System\n");
printf("----------------------\n");
printf("1. Check Balance\n");
printf("2. Deposit\n");
printf("3. Withdraw\n");
printf("4. Transfer\n");
printf("5. Quit\n");
printf("Enter your choice: ");
Rio_writen(connfd, &choice, sizeof(int));
if (choice == 1)
{
printf("Your balance is %s\n", buffer);
}
else if (choice == 2)
{
printf("Enter amount to deposit: ");
Rio_writen(connfd, &amount, sizeof(float));
printf("%s\n", buffer);
}
else if (choice == 3)
{
printf("Enter amount to withdraw: ");
Rio_writen(connfd, &amount, sizeof(float));
printf("%s\n", buffer);
}
else if (choice == 4)
{
printf("Enter amount to transfer: ");
printf("Enter recipient account number: ");
Rio_writen(connfd, &amount, sizeof(float));
Rio_writen(connfd, &acc2, sizeof(int));
printf("%s\n", buffer);
printf("Recipient's new balance is %s\n", buffer);
}
else if (choice == 5)
{
Close(connfd);
printf("Connection Closed!!\n");
break;
}
}
exit(0);
}
//server.c
#include "csapp.h"
void *thread(void *vargp);
int main(int argc, char **argv)
{
int listenfd, *connfdp;
socklen_t clientlen;
struct sockaddr_storage clientaddr;
pthread_t tid;
listenfd = Open_listenfd(argv[1]);
while (1)
{
clientlen = sizeof(struct sockaddr_storage);
connfdp = Malloc(sizeof(int));
*connfdp = Accept(listenfd, (SA *)&clientaddr, &clientlen);
Pthread_create(&tid, NULL, thread, connfdp);
}
}
void *thread(void *vargp)
{
int connfd = *((int *)vargp);
Pthread_detach(pthread_self());
Free(vargp);
FILE *fp = fopen("bank.txt", "r");
char buf[MAXLINE];
int account_number, balance;
char name[MAXLINE];
rio_t rio;
while (fgets(buf, MAXLINE, fp) != NULL)
{
sscanf(buf, "%d %s %d", &account_number, name, &balance);
}
fclose(fp);
sprintf(buf, "%d", balance);
Rio_writen(connfd, buf, strlen(buf));
while (1)
{
if (Rio_readlineb(&rio, buf, MAXLINE) <= 0)
{
break;
}
int choice = buf[0] - '0';
if (choice == 1)
{
sprintf(buf, "%d\n", balance);
Rio_writen(connfd, buf, strlen(buf));
}
else if (choice == 2)
{
if (Rio_readlineb(&rio, buf, MAXLINE) <= 0)
{
break;
}
int deposit_amount = atoi(buf);
balance += deposit_amount;
sprintf(buf, "Deposit successful. New balance: %d\n", balance);
Rio_writen(connfd, buf, strlen(buf));
}
else if (choice == 3)
{
if (Rio_readlineb(&rio, buf, MAXLINE) <= 0)
{
break;
}
int withdrawal_amount = atoi(buf);
if (balance >= withdrawal_amount)
{
balance -= withdrawal_amount;
sprintf(buf, "Withdrawal successful. New balance: %d\n", balance);
Rio_writen(connfd, buf, strlen(buf));
}
}
else if (choice == 4)
{
if (Rio_readlineb(&rio, buf, MAXLINE) <= 0)
{
break;
}
int transfer_amount = atoi(buf);
if (Rio_readlineb(&rio, buf, MAXLINE) <= 0)
{
break;
}
int destination_account = atoi(buf);
if (balance >= transfer_amount)
{
balance -= transfer_amount;
sprintf(buf, "Transfer successful. New balance: %d\n", balance);
Rio_writen(connfd, buf, strlen(buf));
}
}
else if (choice == 5)
{
Close(connfd);
break;
}
}
return NULL;
}
//bank.txt
100 John Smith 500.00
200 Jane Doe 1000.00
300 Luke Skylar 1500.00
Can you help me make a connection with the client and server and be able to read the text file?

Step by step
Solved in 5 steps

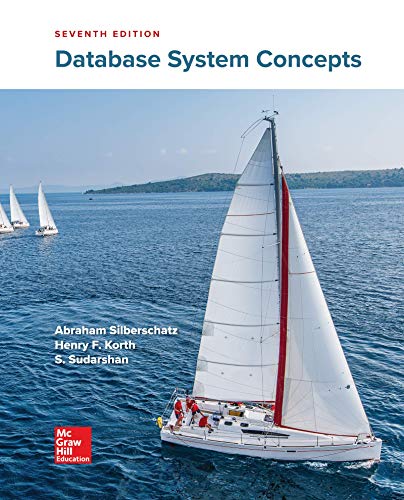
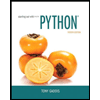
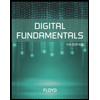
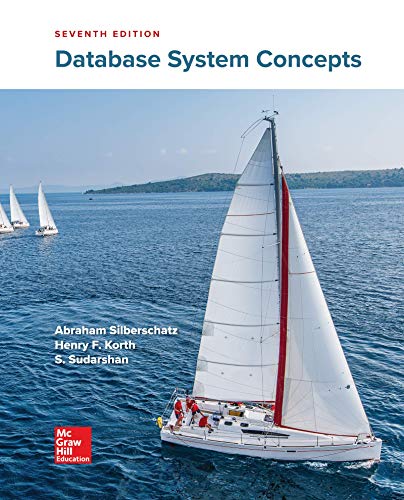
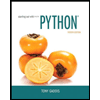
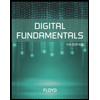
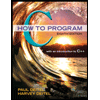
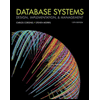
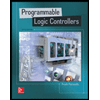