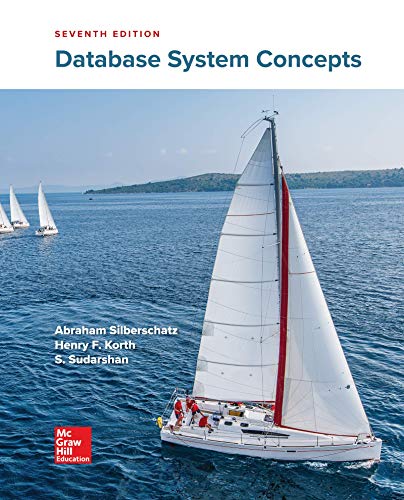
I have this Java code
it's supposed to take in a user input and reverse it and then print it reversed
so if i enter Hello There
it's supposed to print out ereth olleh or something like that. just the phrase backwards
and a while loop is supposed to make sure that it keeps running back and doing the same thing over again until the user enters Done, done or d at which point the input is not to be printed
so user input is hi the first time, and the user input is hello the second time and if its done the third time
ih
olleh
are supposed to be printed but unfortunately the code i have bellow frequently throws up erros and prints the done which it should not do. what am i doing wrong?
import java.util.Scanner;
public class LabProgram {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
String stringman;
stringman = "Hello There";
if (stringman == "done")
{
System.exit(0);
}
else{
while (stringman != "done")
{
StringBuilder input2 = new StringBuilder();
input2.append(stringman);
input2.reverse();
System.out.println(input2);
stringman = scnr.next();
}
}
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

Thank you so much
This works absolutely great it got rid of the errors and doesnt print any done
but what do i do if i need the phrase "Hello There"
to print as "ereht olleH"
and then when it goes through the loop for another input for it to print a yeH bellow in reverse. so then I have an a total output of
"ereht olleH"
"yeH"
how do i do that?
Thank you so much
This works absolutely great it got rid of the errors and doesnt print any done
but what do i do if i need the phrase "Hello There"
to print as "ereht olleH"
and then when it goes through the loop for another input for it to print a yeH bellow in reverse. so then I have an a total output of
"ereht olleH"
"yeH"
how do i do that?
- in java Jump to level 1 The first and second integers in the input are read into variables previousNum and currentNum, respectively. Write a loop that iterates until currentNum is not equal to 6 plus previousNum. In each iteration: Output currentNum, followed by " is 6 plus ", previousNum, and ". Sequence is increasing arithmetically." End with a newline. Assign previousNum with currentNum. Read the next integer from input and assign currentNum with the integer read. After the loop, output the last integer read followed by " breaks the sequence." and a newline. Click here for example Note: Assume that input has at least two integers. 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 import java.util.Scanner; public class Sequencing { publicstaticvoidmain(String[] args) { Scannerscnr=newScanner(System.in); intcurrentNum; intpreviousNum; previousNum=scnr.nextInt(); currentNum=scnr.nextInt(); System.out.println("Sequence starts at "+previousNum+"."); /* Your code…arrow_forwardWrite a program that will print asterisks in the shape of a diamond. The height of the diamond will depend on the value entered by the user. That value MUST be greater than 0, and it MUST be odd. To make sure that the value is correct, you will validate the value once the user has entered it using a validation loop. Then you will print out a diamond in the pattern demonstrated in the sample execution. Remember that the height will vary based on the user input.arrow_forwardIn Java Integer in is read from input. Write a while loop that iterates until in is less than or equal to 0. In each iteration: Update in with the quotient of in and 7. Output the updated in, followed by a newline. Click here for exampleEx: If the input is 2401, then the output is: 343 49 7 1 0 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 import java.util.Scanner; public class QuotientCalculator { publicstaticvoidmain(String[] args) { Scannerscnr=newScanner(System.in); intin; in=scnr.nextInt(); /your code here/ } }arrow_forward
- Write a java program to accept 5 valid positive numbers from 1 to 15 from the user into one variable num using a loop while iterating find the highest of the accepted valid numbers and store it in highest valid number in a variable named max. And display the highest number among the five valid number. Watch the video attached for the output Sample. Note: o All five numbers should be accepted before displaying the highest (use loop) o If the user enters an invalid number, display invalid number, and accept a valid number.arrow_forwardWrite a program that receives an integer input from the user and call it n. Use a for loop, an if condition, and a continue keyword, to find the summation of all numbers that are not a multiple of 3, from 1 to n, including 1 and n and print the result. For instance, the output of your program for 9, should be 27 and for 17 should be 108. You can get an input from user and convert it to an integer using a = int(input('Please enter an integer:')).arrow_forwardWrite a Java program that: Ask user to enter the last two digits of the Student ID number and store it in a variable of type integer; Use a while loop to print all the positive odd numbers smaller than the inputted number. After the while loop, make a for loop to print all the positive even numbers smaller than the inputted Note: Your answer should have the code as text, as well as a screenshot of the code with the output. The inputted number should be the last two digits of your Student ID number. ID is : 190058401arrow_forward
- Write a program with the most appropriate loop that repeats user entered number of times (say the variable counts holds that number), asking the user counts times to enter numbers. The loop should also calculate the sum and the average of the numbers entered. Be sure to include the following: A class header with an appropriately named class. A main method. Use Scanner class for keyboard input. Declare and initialize any necessary variables. Use appropriate loop statement. Display any necessary input prompt and the output.arrow_forwardWrite a Java program called Decision that includes a while loop to prompt the user to enter 5 marks using theJOptionPane statement and a System.out statement to output a message inside the loop highlighting a passmark >= 50 and <= 100. Any other mark will output a messaging stating it’s a fail.Example of the expected output is as follows:55 is a pass12 is a failarrow_forwardWrite a Python program that asks the user to input 2 numbers. add the 2 numbers together print a statement that shows the 2 numbers that were entered and their sum. For instance, if I input 2 and 4 the output should say, "The sum of 2 and 4 is 6". It is probably easiest to use the repl.it web site....you can write your code and run it there - BUT - you have to turn in the code to Canvas. Just copy and paste the code into the assignmentarrow_forward
- Write a java program that uses while loops to perform the following steps: a. Prompt the user to input two integers: firstNum and secondNum. (firstNum must be less than secondNum.) b. Output all the odd numbers between firstNum and secondNum inclusive. c. Output the sum of all the even numbers between firstNum and secondNum inclusive. d. Output all the numbers and their squares between 1 and 10. e. Output the sum of the squares of all the odd numbers between firstNum and secondNum inclusive. f. Output all the uppercase letters.arrow_forwardWrite a Java program using the three different Loops (for/do...while/while) to calculate the sum of numbers from 1-1000. The following should be the output of your program: === Calculating the Sum of Numbers 1-1000 === =>> Using for Loop The Sum is: 500500 =>> Using do...while Loop === The Sum is: 500500 =>> Using while Loop The Sum is: 500500 Job Done!===arrow_forwardYOU SHOULD NOT USE LOOPS (for, while,….) OR CONDITIONALS (if..else,….),For this question, you should use Math function for doing the “power” and “π”. When printingthe volume and the area values, DON’T ENTER THEM MANUALLY. Only print 2 numbers after the decimal points.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
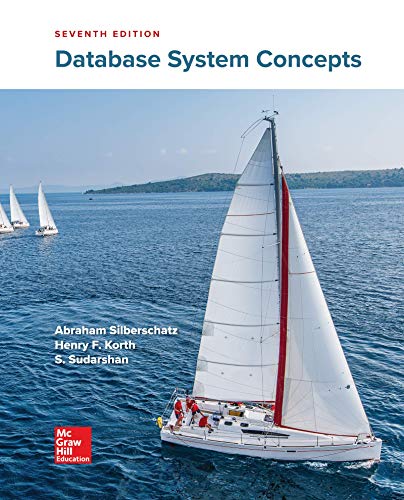
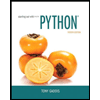
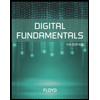
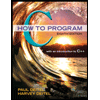
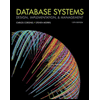
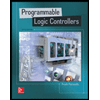