I have written some code however I am receiving error for task 1 and 2 as they are not completed for the toString. Please add toString. and my computemyaverage is receiving errors as well with decimal. Please help and fix. I have given the errors I am receiving below. This is the code that needs it added and changed package assignment; public class PassFailCourse extends Course { //See Assignment 3 or "High Level View of Your Tasks" in Folio to see what Course contains /** * This overrides Course's computerCourseAverage method. * The course is Pass / Fail, which means you will not receive a specific grade. * however, you need a 75% average to pass the course. * (Remember that the Student class has computeMyAverage as a method) * @return the number of students who's average of all the student's quizzes is 75% or better divided by the total students in the course */ @Override public double computeCourseAverage() { //counter for passing students double countP = 0; double pass = 75; //for loop to determine each student's average is what letters grade //increase the count for this for (int i = 0; i < getAllStudents().size(); i++) { //need to call computeMyAverage method of the Student class double avg = getAllStudents().get(i).computeMyAverage(); //if statements increase counter for passing if student's average is at or above 75% if (avg>= pass) { countP++; } } //RETURN double passingStudents = (countP/getAllStudents().size()); return passingStudents; } } Here is the course class because this class extends Course package assignment; import java.util.ArrayList; public class Course { // member variable to store all students in a course ArrayList students; // default constructor public Course() { students = new ArrayList(); } /** * @return the allStudents */ public ArrayList getAllStudents() { return students; } /** * @param allStudents the allStudents to set */ public void setAllStudents(ArrayList allStudents) { students = allStudents; } /** * * @param stu Student object * @return true if successfully added, false otherwise */ public boolean addStudent(Student stu) { // if not valid if (stu == null) return true; // loop over all students and check if already exists for (Student student : students) { if (student.equals(stu)) return false; } return students.add(stu); } /** * @desc removes a student if exists * @param stu Student object * @return true if already exists, false otherwise */ public boolean removeStudent(Student stu) { // remove the student if valid and exists return students.remove(stu); } /** * @desc updates a student if it exists * @param stu * @return true if students exists and update successfull, * false otherwise */ public boolean updateStudent(Student stu) { // if not valid if (stu == null) return false; // if list containts the student if (students.contains(stu)) { students.set(students.indexOf(stu), stu); return true; } return false; } /** * @return the average of all the student's quizzes */ public double computeCourseAverage() { double scoresAvgSum = 0.0; int totalScores = 0; for(int i = 0;i but was:<[]Students in this cou...> at tests.PassFailCourseTests.testToString1:160 (PassFailCourseTests.java) Test Failed! Make sure your toString is correctly formatted. expected:<[Programming Principles 3 (the secret ultimate course!!) is a pass/fail course. 75.00% of the class are on track to pass the course. ]Students in this cou...> but was:<[]Students in this cou...> at tests.PassFailCourseTests.testToString2:188 (PassFailCourseTests.java) Test Failed! Make sure your computeCourseAverage logic is correct. Only students above 75% average should be considered passing. expected:<50.0> but was:<0.5> at tests.PassFailCourseTests.testComputeCourseAverage5:135 (PassFailCourseTests.java) use driver attached to see if code runs please. need help.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
I have written some code however I am receiving error for task 1 and 2 as they are not completed for the toString. Please add toString. and my computemyaverage is receiving errors as well with decimal. Please help and fix. I have given the errors I am receiving below.
This is the code that needs it added and changed
package assignment;
public class PassFailCourse extends Course {
//See Assignment 3 or "High Level View of Your Tasks" in Folio to see what Course contains
/**
* This overrides Course's computerCourseAverage method.
* The course is Pass / Fail, which means you will not receive a specific grade.
* however, you need a 75% average to pass the course.
* (Remember that the Student class has computeMyAverage as a method)
* @return the number of students who's average of all the student's quizzes is 75% or better divided by the total students in the course
*/
@Override
public double computeCourseAverage() {
//counter for passing students
double countP = 0;
double pass = 75;
//for loop to determine each student's average is what letters grade
//increase the count for this
for (int i = 0; i < getAllStudents().size(); i++) {
//need to call computeMyAverage method of the Student class
double avg = getAllStudents().get(i).computeMyAverage();
//if statements increase counter for passing if student's average is at or above 75%
if (avg>= pass) {
countP++;
}
}
//RETURN
double passingStudents = (countP/getAllStudents().size());
return passingStudents;
}
}
Here is the course class because this class extends Course
package assignment;
import java.util.ArrayList;
public class Course {
// member variable to store all students in a course
ArrayList<Student> students;
// default constructor
public Course() {
students = new ArrayList<Student>();
}
/**
* @return the allStudents
*/
public ArrayList<Student> getAllStudents() {
return students;
}
/**
* @param allStudents the allStudents to set
*/
public void setAllStudents(ArrayList<Student> allStudents) {
students = allStudents;
}
/**
*
* @param stu Student object
* @return true if successfully added, false otherwise
*/
public boolean addStudent(Student stu) {
// if not valid
if (stu == null)
return true;
// loop over all students and check if already exists
for (Student student : students) {
if (student.equals(stu))
return false;
}
return students.add(stu);
}
/**
* @desc removes a student if exists
* @param stu Student object
* @return true if already exists, false otherwise
*/
public boolean removeStudent(Student stu) {
// remove the student if valid and exists
return students.remove(stu);
}
/**
* @desc updates a student if it exists
* @param stu
* @return true if students exists and update successfull,
* false otherwise
*/
public boolean updateStudent(Student stu) {
// if not valid
if (stu == null)
return false;
// if list containts the student
if (students.contains(stu)) {
students.set(students.indexOf(stu), stu);
return true;
}
return false;
}
/**
* @return the average of all the student's quizzes
*/
public double computeCourseAverage() {
double scoresAvgSum = 0.0;
int totalScores = 0;
for(int i = 0;i<students.size();i++){
if(students.get(i) != null) {
Score[] studentScores = students.get(i).getAllMyScores();
for(int j = 0; j < studentScores.length; j++) {
if(studentScores[j] != null) {
scoresAvgSum += studentScores[j].getScoreValue();
totalScores++;
}
}
}
}
return scoresAvgSum/totalScores;
}
public String getNumberOfStudents() {
// TODO Auto-generated method stub
return null;
}
}
Here are my errors
Make sure your toString is correctly formatted. expected:<[Underwater Basket Weaving is a pass/fail course. 50.00% of the class are on track to pass the course. ]Students in this cou...> but was:<[]Students in this cou...> at tests.PassFailCourseTests.testToString1:160 (PassFailCourseTests.java)
Test Failed! Make sure your toString is correctly formatted. expected:<[Programming Principles 3 (the secret ultimate course!!) is a pass/fail course. 75.00% of the class are on track to pass the course. ]Students in this cou...> but was:<[]Students in this cou...> at tests.PassFailCourseTests.testToString2:188 (PassFailCourseTests.java)
Test Failed! Make sure your computeCourseAverage logic is correct. Only students above 75% average should be considered passing. expected:<50.0> but was:<0.5> at tests.PassFailCourseTests.testComputeCourseAverage5:135 (PassFailCourseTests.java)
use driver attached to see if code runs please. need help.
![package assignment;
public class A4Pass FailDriver {
public static void main(String[] args) {
//NOTE: BELOW IS A STARTER SET OF TEST FOR YOUR ASSIGNMENT.
//IT IS NOT THE ONLY SET OF TESTS YOU SHOULD DO FOR YOUR ASSIGNMENT !
//Student Class
Student stul = new Student ("Bugsy", 521);
stul. AddScore (new
Score ("q1", 90.1));
stul.AddScore (new
Score ("q2", 87.21));
stul. AddScore (new Score ("q3", 94));
Student stu2 = new Student ("Daisy", 321);
stu2.AddScore (new Score ("q1", 80.36));
stu2. AddScore (new Score ("q2", 92.70));
stu2. AddScore (new Score ("q3", 86.3));
Student stu3 = new Student ("Minny", 876);
stu3.AddScore (new Score ("q1", 75.75));
stu3.AddScore (new Score ("q2", 83.45));
stu3.AddScore (new Score ("q3", 78.98));
Student stu4 = new Student ("Mikey", 543);
stu4.AddScore (new Score ("ql", 60.99));
stu4.AddScore (new Score ("q2", 78.67));
stu4.AddScore (new Score ("q3", 50.38));
//PassFailCourse object stored in Course object reference variable
Course cou = new Pass FailCourse ("Underwater Basket Weaving");
System.out.println("Add Student to course successfully ->"+ cou.addStudent (stul));
System.out.println("Add Student to course successfully ->"+ cou.addStudent (stu2));
System.out.println("Add Student to course successfully ->"+ cou.addStudent (stu3));
System.out.println("Add Student to course successfully ->"+ cou.addStudent (stu4));
//PassFailCourse overrides computeCourseAverage so it returns the percentage of students that are passing (at or above 75%)
System.out.println("Percentage of students with grade above 75% ->"+ cou.compute CourseAverage () );
System.out.println("Number of Students in the Course ->"+ cou.getAllStudents ().size());
System.out.println("toString prints the following\n"+cou.toString());](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F45fadc90-cd80-481e-8b65-2add153b12e5%2F948f7b8d-8d71-40cc-9180-5a7be4d14169%2F34zv89s_processed.png&w=3840&q=75)
![Tasks - PassFailCourse
Class
• Task 1: The PassFailCourse class contains all the functionality of the Course class as it is a child class of Course class. You are given the Course class in the provided.jar
file named A3.jar.
Task 2: A PassFailCourse object can be created by giving as an argument a course name as a String. Complete the method public Pass FailCourse(String courseName) to
complete this task. See the method comments for more detail.
• Task 3: The user can get the percentage of students in the course on track to pass this PassFailCourse. The method will return a String containing the percent of students
who's average of all the student's quizzes is at or better then 75% currently. Complete the method public double compute Course Average() to complete this task. The public
double compute Course Average() overrides the method of of the superclass. See the method comments for more detail.
• Task 4: The user will be able to ask for the textual representation of this PassFailCourse instance. The String includes the course name, type of course, percentage of the
class that are above 75% average, and the superclass' toString. The method will return a String in the form specified in the example below. Complete the method public
String toString() to complete this task. See the method comments for more detail.
• Underwater Basket Weaving is a pass/fail course. 75.00% of the class are on track to pass the course. Students in this course:
[Bugsy 521, Daisy 321, Minny 876, Mikey 543]
Test your code using the A4PassFailDriver class, then submit your code on Gradescope. Fix any issues and resubmit to Gradescope as many time before the deadline as needed to
maximize your score for this task.
A4Pass FailDriver Example Output
Add Student to course successfully ->true
Add Student to course successfully ->true
Add Student to course successfully ->true
Add Student to course successfully ->true
Percentage of students with grade above 75% ->75.0
Number of Students in the Course ->4
toString prints the following
Underwater Basket Weaving is a pass/fail course. 75.00% of the class are on track to pass the course. Students in this course:
[Bugsy 521, Daisy 321, Minny 876, Mikey 543]](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F45fadc90-cd80-481e-8b65-2add153b12e5%2F948f7b8d-8d71-40cc-9180-5a7be4d14169%2F1lhdra_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

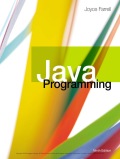
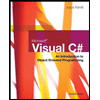
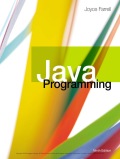
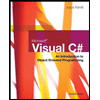