I keep getting Java null point exception for makeMovielist
Here is the code I have done so far. I keep getting Java null point exception for makeMovielist
code:
import java.util.ArrayList;
import java.util.Arrays;
public class MovieList {
/**
* This method will take the name of a movie and delete it
* in the movies if exists and
* delete its corresponding year in years
*
* @param name movie name to delete
* @param movies List of all movies' name
* @param years List of corresponding years
*/
public static void deleteMovieByName(String name, ArrayList<String> movies,
ArrayList<Integer> years) {
if(name==null || movies == null || years == null)
return;
for(int i=0; i<movies.size(); i++){
if(movies.get(i).equals(name)){
movies.remove(i);
years.remove(i);
}
}
}
/**
* This method will delete all movies with specific year
* in the movie list and year list
*
* @param year the given year
* @param movies List of all movies' name
* @param years List of corresponding years
*/
public static void deleteMoviesByYear(int year, ArrayList<String> movies,
ArrayList<Integer> years) {
if(movies == null || years == null)
return;
for(int i=0; i<years.size(); i++){
if(years.get(i)==year){
movies.remove(i);
years.remove(i);
}
}
}
/**
* This method will return a string that contains each
* movie name and its year.
* Each line will format as follow:
* <movie name> (<year>)\n
*
* @param movies List of all movies' name
* @param years List of corresponding years
* @return string contains all movie names and years
*/
public static String makeMovieList(ArrayList<String> movies,
ArrayList<Integer> years) {
String result = "";
for(int i=0; i<movies.size(); i++){
result += movies.get(i)+" ("+years.get(i)+")\n";
}
return result;
}
/**
* test for deleteMovieByName()
* @param movies
* @param years
*/
public static void testDeleteByName(ArrayList<String> movies,
ArrayList<Integer> years) {
boolean error = false;
deleteMovieByName("Fight Club", movies, years);
ArrayList<String> expectedMovie1 = new ArrayList<>(
Arrays.asList("The Shawshank Redemption","The Godfather",
"The Godfather: Part II", "The Dark Knight",
"12 Angry Men", "Schindler's List",
"The Lord of the Rings: The Return of the King",
"Pulp Fiction", "The Good, the Bad and the Ugly"
));
ArrayList<Integer> expectedYears1 = new ArrayList<>(
Arrays.asList(1994, 1972, 1974, 2008, 1957,
1993, 2003, 1994, 1966));
if(!movies.equals(expectedMovie1) || !years.equals(expectedYears1)){
error = true;
System.out.println("Expected movie list: " + expectedMovie1);
System.out.println("Actual movie list: " + movies);
System.out.println("Expected movie list: " + expectedYears1);
System.out.println("Actual movie list: " + years);
}
//add more test cases
if(error){
System.out.println("testDeleteByName fail");
}else{
System.out.println("testDeleteByName pass");
}
}
/**
* test for deleteMoviesByYear()
* @param movies
* @param years
*/
public static void testDeleteByYear(ArrayList<String> movies,
ArrayList<Integer> years) {
}
/**
* test for makeMovieList()
* @param movies
* @param years
*/
public static void testMakeMovieList(ArrayList<String> movies,
ArrayList<Integer> years) {
}
public static void main(String[] args) {
ArrayList<String> movies = new ArrayList<>(
Arrays.asList("The Shawshank Redemption","The Godfather",
"The Godfather: Part II", "The Dark Knight",
"12 Angry Men", "Schindler's List",
"The Lord of the Rings: The Return of the King",
"Pulp Fiction", "The Good, the Bad and the Ugly",
"Fight Club"
)
);
ArrayList<Integer> years = new ArrayList<>(
Arrays.asList(1994, 1972, 1974, 2008, 1957,
1993, 2003, 1994, 1966, 1999));
testDeleteByName(movies, years);
//testDeleteByYear(movies, years);
//testMakeMovieList(movies, years);
}
}
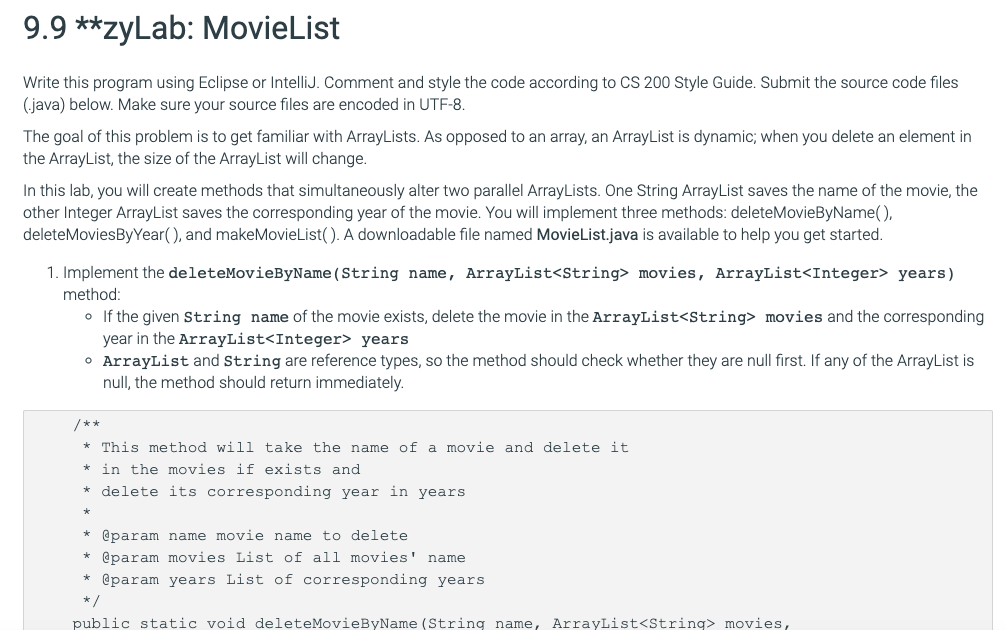
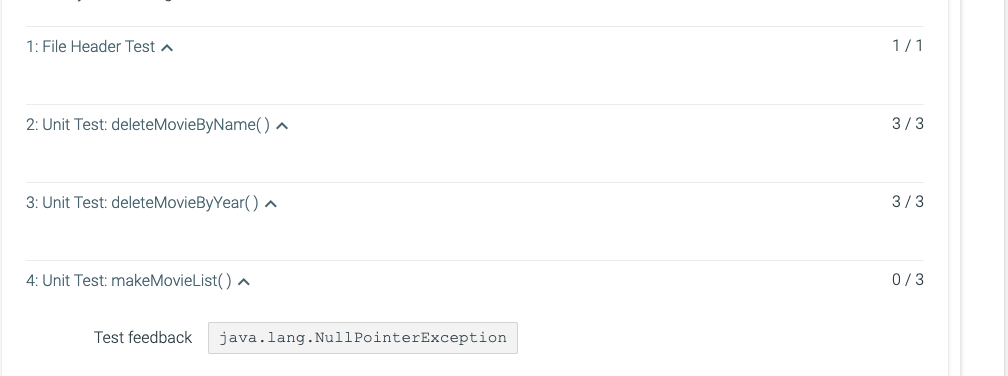

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

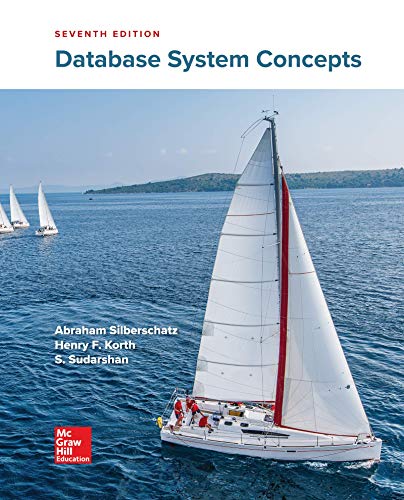
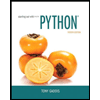
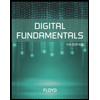
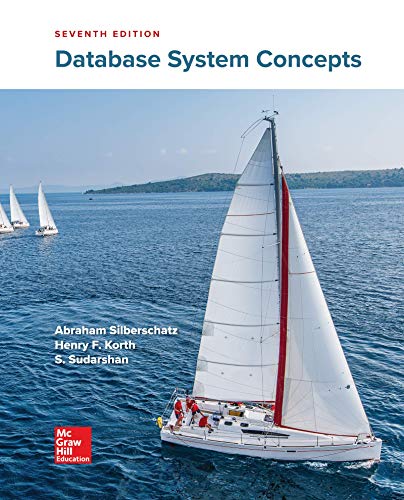
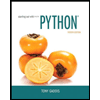
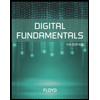
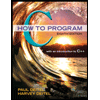
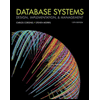
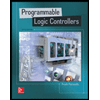