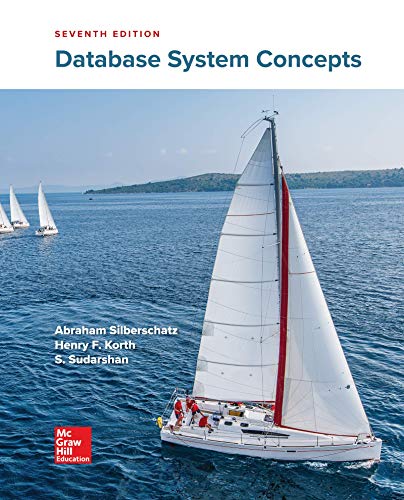
import java.util.Scanner;
public class ModifyArray {
public swapArrayEnds(10, 20, 30, 40) {
for (i = 0; i < (arrVals.length / 2); ++i) {
tempValue = arrVals[i]; // Do swap //*please fix code//
arrVals[i] = arrVals[arrVals.length - 1 - i];
arrVals[arrVals.length - 1 - i] = tempValue;
Write a method swapArrayEnds() that swaps the first and last elements of its array parameter. Ex: sortArray = {10, 20, 30, 40} becomes {40, 20, 30, 10}.
public static void main (String [] args) {
Scanner scnr = new Scanner(System.in);
int numElem = 4;
int[] sortArray = new int[numElem];
int i;
int userNum;
for (i = 0; i < sortArray.length; ++i) {
sortArray[i] = scnr.nextInt();
}
swapArrayEnds(sortArray);
for (i = 0; i < sortArray.length; ++i) {
System.out.print(sortArray[i]);
System.out.print(" ");
}
System.out.println("");
}
}
![CTU Class Homepage.
H
zy Section 4.4 - CS 226T: Java Progra X
✰ learn.zybooks.com/zybook/CS226-2204A-03-2204A/chapter/4/section/4
=zyBooks My library > CS 226T: Java Programming home > 4.4: Array parameters
Run
Thank you. Your reservation is co X
ART
Common student error
Your code used a for loop. Instead, swapping the first and last elements requires a temporary variable, not a loop.
Failed to compile
ModifyArray.java:5: error: invalid method declaration; return type required
public swapArrayEnds (10, 20, 30, 40) {
Print - Your Confirmed Reservatic X b Answered: 4.2.3: Method definiti X
ModifyArray.java:5: error: illegal start of type
public swapArrayEnds (10, 20, 30, 40) {
3 errors
ModifyArray.java:12: error: illegal start of expression
public static void main (String [] args) {
How was this section?
Note: Although the reported line number is in the uneditable part of the code, the error actually exists in your code. Tools often
don't recognize the problem until reaching a later line.
Provide feedback
zyBooks catalog
Activity summary for assignment: Unit 4 Interactive Assignment
Due: 10/31/2022, 3:59 PM -08
Feedback?
? Help/FAQ
70 %
70% submitted to
Stacey Queen
X
:
9:11 AM
8/30/2022](https://content.bartleby.com/qna-images/question/788fc729-a951-45c4-9909-47eea7bbf82f/068e5bb6-79ec-4ded-92a1-143614a71080/pxc0v1_thumbnail.png)

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

- Java How do I use printf to format this to money with .00 ? public static void main(String[] args) { PaymentBatchProcessor <Payment> processor = new PaymentBatchProcessor<>(); // create payements: checks(amount) and IOUs(amount) Payment payment1 = new Check(25000.63); Payment payment2 = new IOU(3600.56); Payment payment3 = new Check(250000.64); Payment payment4 = new IOU(36000.56); Payment payment5= new Check(2500.63); Payment payment6= new IOU(36007.56); //Add payments to payment batch Processor processor.add(payment1); processor.add(payment2); processor.add(payment3); processor.add(payment4); processor.add(payment5); processor.add(payment6); //Print out getSize, getMax, getTotal //use printf = "'%5.2f'%n" for money format double System.out.printf("Payments: " + "'%5.2f'%n",processor.getSize()); System.out.printf("Max payment: " + "'%5.2f'%n",processor.getMax()); System.out.printf("Total payment: " +"'%5.2f'%n",processor.getTotal());arrow_forwardWhat’s the output?arrow_forwardUSING C++, IN VISUAL STUDIOS, I NEED HELP WITH THIS. template class Dictionary (friend class UnitTests Lab5,// Giving access to test code struct Pair (Key key. Value value; Pair(const Key& key, const Value& value) { // TODO: Implement this method key = key; value = value; } // For testing bool operator = = (const Pair& comp) const { return ( comp.key = = key && comp.value == value);]]; // Data members // NOTE: All values set to -1 for unit test purposes std::list mTable = reinterpret cast">(-1); // A dynamic array of lists (these are the buckets) size_t mNumBuckets=1; // Number of elements in mlable unsigned int("mHashFunc) (const Key&) = reinterpret cast(-1); // Pointer to the hash function public: Dictionary(size_t_numBuckets, unsigned int ("_hashFunc) (const Key&)) // TODO: Implement this method ) --Dictionary (// TODO: Implement this method] Dictionary(const Dictionary& copy) { // TODO: Implement this method) Dictionary& operator= (const…arrow_forward
- Implement in C Programming 6.11.2: Modify an array parameter. Write a function SwapArrayEnds() that swaps the first and last elements of the function's array parameter. Ex: sortArray = {10, 20, 30, 40} becomes {40, 20, 30, 10}. #include <stdio.h> /* Your solution goes here */ int main(void) { const int SORT_ARR_SIZE = 4; int sortArray[SORT_ARR_SIZE]; int i; int userNum; for (i = 0; i < SORT_ARR_SIZE; ++i) { scanf("%d", &sortArray[i]); } SwapArrayEnds(sortArray, SORT_ARR_SIZE); for (i = 0; i < SORT_ARR_SIZE; ++i) { printf("%d ", sortArray[i]); } printf("\n"); return 0;}arrow_forwardLab2.java contains the following code: public final class Lab2 { /** * This is empty by design, Lab2 cannot be instantiated */ private Lab2() { // empty by design } /** * Returns the sum of a consecutive set of numbers from <code> start </code> to <code> end </code>. * * @pre <code> start </code> and <code> end </code> are small enough to let this * method return an int. This means the return value at most requires 4 bytes and * no overflow would happen. * * @param start is an integer number * @param end is an integer number * @return the sum of start + (start + 1) + .... + end */ public static int sum(int start, int end) { //Insert your code here and change the return statement to fit your implementation. return 0; } /** * This method creates a string using the given char * by repeating the character <code> n </code> times. * * @param first is…arrow_forwardJAVA Language: Transpose Rotate. Question: Modify Transpose.encode() so that it uses a rotation instead of a reversal. That is, a word like “hello” should be encoded as “ohell” with a rotation of one character. (Hint: use a loop to append the letters into a new string) import java.util.*; public class TestTranspose { public static void main(String[] args) { String plain = "this is the secret message"; // Here's the message Transpose transpose = new Transpose(); String secret = transpose.encrypt(plain); System.out.println("\n ********* Transpose Cipher Encryption *********"); System.out.println("PlainText: " + plain); // Display the results System.out.println("Encrypted: " + secret); System.out.println("Decrypted: " + transpose.decrypt(secret));// Decrypt } } abstract class Cipher { public String encrypt(String s) { StringBuffer result = new…arrow_forward
- What does the following code do? In [1]: def mystery (a, b) : if b == 1: return a else: return a + mystery (a, b - 1) In [2]: mystery (2, 10) Out [2]: ?????arrow_forwardThis is What i have so far. #include <iostream> // for cin and cout#include <iomanip> // for setw() and setfill()using namespace std; // so that we don't need to preface every cin and cout with std:: void printFirstTwoBuildingSection(int n, int startSpacing){int start = n / 2, end = 0;if (n <= 2){start = 0;}for (int i = 0; i < n; i++){for (int j = 0; j < startSpacing; j++){cout << " ";}cout << "|";if (i < (n / 2)){for (int k = 0; k < i; k++){cout << " ";}cout << "\\";for (int k = 0; k < start; k++){cout << " ";}cout << "/";start -= (n / 2);for (int k = 0; k < i; k++){cout << " ";}cout << "|" << endl;}else{for (int k = n - i - 1; k > 0; k--){cout << " ";}cout << "/";for (int k = 0; k < end; k++){cout << " ";}cout << "\\";end += (n / 2);for (int k = n - i - 1; k > 0; k--){cout << " ";}cout << "|" << endl;}}for (int j = 0; j < startSpacing;…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
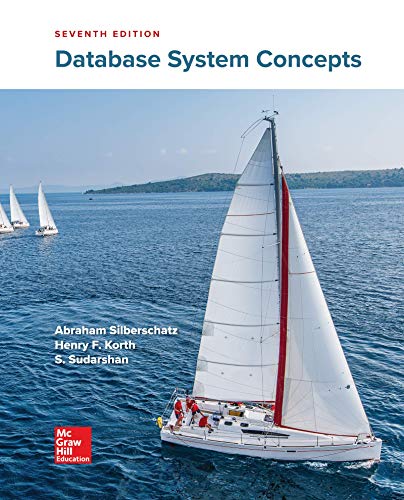
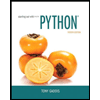
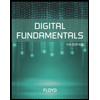
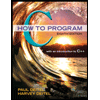
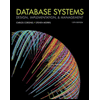
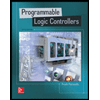