I Love Shapes
1. I Love Shapes
by Codechum Admin
Shapes are the best thing in the world! We see them everywhere, from triangular pizza pies to her perfectly shaped almond eyes ?
For this program, you are tasked to implement the Shape class. This class should be an abstract class and should have the following properties:
- private String name
- private String color
- private boolean isFlat
It should have a constructor with the following signature, public Shape(String name, String color, boolean isFlat), getter methods for all the properties, and a setter method for the color.
Then implement two more abstract classes that inherit from this Shape class: TwoDShape and ThreeDShape. The TwoDShape will have the following additional details:
-
Additional property:
- private int numberOfSides
-
Additional methods:
- getter for the numberOfSides
- public abstract double getArea()
- public abstract double getPerimeter()
- Constructor with the following signature: public TwoDShape(String name, String color, int numberOfSides). The constructor will call its parent class' constructor and pass the value true as the third argument (i.e. as the value for the isFlat parameter)
The ThreeDShape on the other hand will have the following additional details:
-
Additional methods:
- public abstract getSurfaceArea()
- public abstract getVolume()
- Constructor with the following signature: public ThreeDShape(String name, String color). The constructor will call its parent class' constructor and pass the value false as the third argument (i.e. as the value for the isFlat parameter)
Then, create the Square class which inherits from the TwoDShape class. This will have the following details:
-
Additional property:
- private double lengthOfSide
-
Additional methods:
- getter for the lengthOfSide
- implementation of the inherited getArea() method (area of a square is side * side)
- implementation of the inherited getPerimeter() method (perimeter of a square is 4 * side)
- Constructor with the following signature: public Square(String color, double lengthOfSide). The constructor will call its parent class' constructor and pass the value "Square" as the first argument (i.e. as the value for the name parameter) and the value 4 as the third argument (i.e. as the value for the numberOfSides parameter)
Also create the RectangularPrism class which inherits from the ThreeDShape class. This will have the following details:
-
Additional properties:
- private double length;
- private double width;
- private double height;
-
Additional methods:
- getter for length, width, and height
- implementation of the inherited getSurfaceArea() method (surface area of a rectangular prism is 2*(w*l + h*l + h*w))
- implementation of the inherited getVolume() method (volume of a rectangular prism is w*h*l)
- Constructor with the following signature: public RectangularPrism(String color, double length, double width, double height). The constructor will call its parent class' constructor and pass the value "Rectangular Prism" as the first argument (i.e. as the value for the name parameter).
For this program, an initial code in the main class is already provided for you.
Finally, before you submit your code, uncomment the Tester code lines.

Step by step
Solved in 6 steps with 3 images

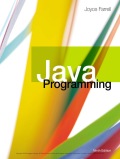
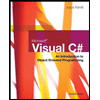
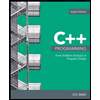
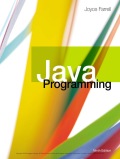
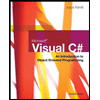
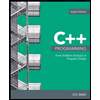