I need help with my Optimizing the intermediate code to improve the efficiency of the generated machine code. I need to improve the effixiency of the generated machine code import java.util.*; public class SimpleCalculator { private final String input; private int position; private boolean hasDivByZero = false; private Map symbolTable; private List instructions; public SimpleCalculator(String input) { this.input = input; this.position = 0; this.symbolTable = new HashMap<>(); this.instructions = new ArrayList<>(); } public static void main(String[] args) { SimpleCalculator calculator = new SimpleCalculator("3 + 5 * (2 - 1)"); calculator.parseExpression(); if (calculator.hasDivByZero) { System.out.println("Error: division by zero"); } else { System.out.println("Result: " + calculator.instructions); } } public void parseExpression() { String t1 = parseTerm(); while (true) { String op = null; if (consume('+')) { op = "+"; } else if (consume('-')) { op = "-"; } else { break; } String t2 = parseTerm(); String result = newTemp(); emit(result + " = " + t1 + " " + op + " " + t2); t1 = result; } } public String parseTerm() { String t1 = parseFactor(); while (true) { String op = null; if (consume('*')) { op = "*"; } else if (consume('/')) { op = "/"; } else { break; } String t2 = parseFactor(); if (op.equals("/") && t2.equals("0")) { hasDivByZero = true; break; } String result = newTemp(); emit(result + " = " + t1 + " " + op + " " + t2); t1 = result; } return t1; } public String parseFactor() { if (consume('(')) { String result = parseExpression(); consume(')'); return result; } StringBuilder sb = new StringBuilder(); while (Character.isDigit(peek())) { sb.append(consume()); } String variable = sb.toString(); if (symbolTable.containsKey(variable)) { String type = symbolTable.get(variable); if (!type.equals("int")) { System.out.println("Error: " + variable + " is not an integer"); } } else { System.out.println("Error: " + variable + " is not defined"); } return variable; } private String newTemp() { String temp = "t" + (instructions.size() + 1); symbolTable.put(temp, "int"); return temp; } private void emit(String instruction) { instructions.add(instruction); } private char consume() { return input.charAt(position++); } private boolean consume(char c) { if (peek() == c) { position++; return true; } return false; } private char peek() { return position < input.length() ? input.charAt(position) : '\0'; } }
I need help with my Optimizing the intermediate code to improve the efficiency of the generated machine code.
I need to improve the effixiency of the generated machine code
import java.util.*;
public class SimpleCalculator {
private final String input;
private int position;
private boolean hasDivByZero = false;
private Map<String, String> symbolTable;
private List<String> instructions;
public SimpleCalculator(String input) {
this.input = input;
this.position = 0;
this.symbolTable = new HashMap<>();
this.instructions = new ArrayList<>();
}
public static void main(String[] args) {
SimpleCalculator calculator = new SimpleCalculator("3 + 5 * (2 - 1)");
calculator.parseExpression();
if (calculator.hasDivByZero) {
System.out.println("Error: division by zero");
} else {
System.out.println("Result: " + calculator.instructions);
}
}
public void parseExpression() {
String t1 = parseTerm();
while (true) {
String op = null;
if (consume('+')) {
op = "+";
} else if (consume('-')) {
op = "-";
} else {
break;
}
String t2 = parseTerm();
String result = newTemp();
emit(result + " = " + t1 + " " + op + " " + t2);
t1 = result;
}
}
public String parseTerm() {
String t1 = parseFactor();
while (true) {
String op = null;
if (consume('*')) {
op = "*";
} else if (consume('/')) {
op = "/";
} else {
break;
}
String t2 = parseFactor();
if (op.equals("/") && t2.equals("0")) {
hasDivByZero = true;
break;
}
String result = newTemp();
emit(result + " = " + t1 + " " + op + " " + t2);
t1 = result;
}
return t1;
}
public String parseFactor() {
if (consume('(')) {
String result = parseExpression();
consume(')');
return result;
}
StringBuilder sb = new StringBuilder();
while (Character.isDigit(peek())) {
sb.append(consume());
}
String variable = sb.toString();
if (symbolTable.containsKey(variable)) {
String type = symbolTable.get(variable);
if (!type.equals("int")) {
System.out.println("Error: " + variable + " is not an integer");
}
} else {
System.out.println("Error: " + variable + " is not defined");
}
return variable;
}
private String newTemp() {
String temp = "t" + (instructions.size() + 1);
symbolTable.put(temp, "int");
return temp;
}
private void emit(String instruction) {
instructions.add(instruction);
}
private char consume() {
return input.charAt(position++);
}
private boolean consume(char c) {
if (peek() == c) {
position++;
return true;
}
return false;
}
private char peek() {
return position < input.length() ? input.charAt(position) : '\0';
}
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps

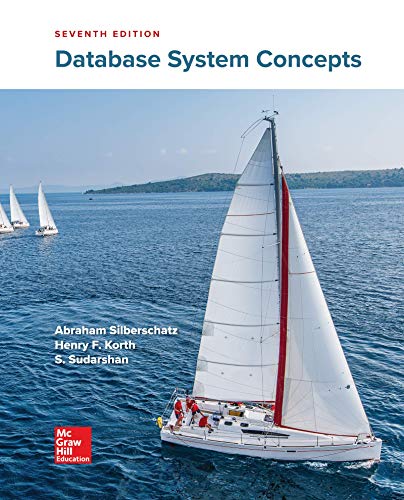
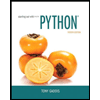
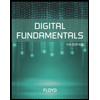
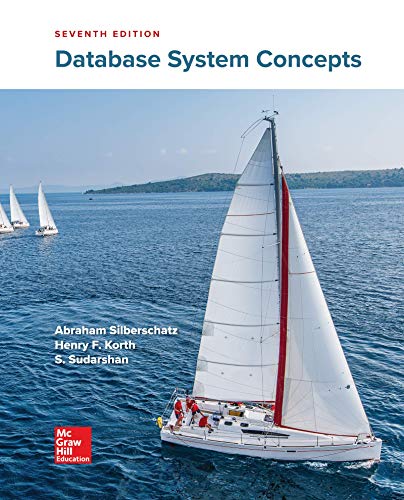
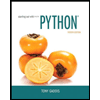
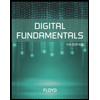
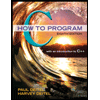
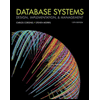
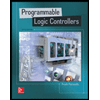