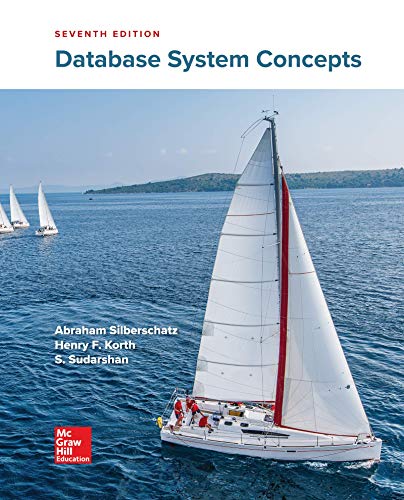
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
You'll write a C++ program to demonstrate thread synchronization. Your main function should first create an empty file called *sync.txt*. Then it will create two separate threads: *Thread-A* and *Thread-B*. Both threads will open *sync.txt* and write to it simultaneously.
*Thread-A* will write the numbers 0 through 9, a total of fifty-thousand times in nested `for` loops, then exit. In other words, print the numbers 0 through 9 over and over again, with each run on a separate line, for 50,000 lines. Here's an example of one such line:
```text
0 1 2 3 4 5 6 7 8 9
```
Somewhat similarly, *Thread-B* will write the letters A through Z fifty-thousand times in nested `for` loops, then exit. In other words, print the letters A through Z over and over again, with each run on a separate line, for 50,000 lines. Here's an example of one such line:
```text
A B C D E F G H I J K L M N O P Q R S T U V W X Y Z
```
Do not write each line as one long string. You must write each character to the file, one by one (i.e., using multiple calls ). After writing a character to the file, write a space too, so the characters appear visually separated.
This means each thread would use a doubly-nested `for` loop. The outer loop would run 50,000 times (once per line), and the inner loop would run either 26 times (once per character) or 10 times (once per digit).
Hopefully, you understand this might create a race condition. Because *Thread-A* and *Thread-B* will both attempt to write individual characters to the same file at the same time, we might expect to see an undesirable mixing of the two types of lines, rather than each line remaining intact.
## Solving With a Critical Section
We'll solve the previous dilemma with a *critical section* in our code. You will use a mutex lock to control synchronization between the two threads, such that each line of numbers or letters will render uninterrupted, even with multiple competing writes to the file.
1. We ***don't*** want to mix letters and numbers on a single line. Each line should only contain letters *or* numbers, but not both.
2. We ***do*** want line types to change sometimes. In other words, you might see a line of letters followed by a line of numbers. Or you may later see a line of numbers followed by a line of letters. You wouldn't want to see 100 lines of letters followed by 100 lines of numbers.
Try to make your *critical section* as small as possible while still solving the race condition for the above criteria.
### Overview of beginWriting()
You'll quickly see the following prototype in your starter code:
```cpp
void beginWriting(bool use_mutexes);
```
At minimum, this function should perform the following steps:
1. Take the incoming argument `use_mutexes` and assign its value to the global variable `G_USE_MUTEXES`.
2. Create both threads.
3. Join both threads.
### Overview of Thread Runner Functions
You will also see two prototypes for your thread runner functions:
```cpp
void* threadAEntry(void* param);
void* threadBEntry(void* param);
``
1. Each individual line contains only letters *OR* numbers, but not both.
2. There should not be 100 lines of one type, followed by 100 lines of another type. Some lines should be of a different type than the last.
Here's an shortened example of what a successfully written file might look like (although yours may be slightly different):
```text
0 1 2 3 4 5 6 7 8 9
A B C D E F G H I J K L M N O P Q R S T U V W X Y Z
A B C D E F G H I J K L M N O P Q R S T U V W X Y Z
0 1 2 3 4 5 6 7 8 9
0 1 2 3 4 5 6 7 8 9
A B C D E F G H I J K L M N O P Q R S T U V W X Y Z
A B C D E F G H I J K L M N O P Q R S T U V W X Y Z
A B C D E F G H I J K L M N O P Q R S T U V W X Y Z
0 1 2 3 4 5 6 7 8 9
0 1 2 3 4 5 6 7 8 9
```
## Confirming Failure
As stated above, no line should have a mix of letters *and* numbers. Here is an example that shows your *critical section* isn't working, or perhaps needs to cover a larger area:
```text
A 0 1 B C 2 3 4 D E 5 F 6 7 G 8 H 9 I J K L M N O P Q R S T U V W X Y Z
```
Also, you should not see a block of 50,000 lines of any particular type in a row. We expect to see the lines switch up a bit. Here's the general idea (some lines omitted and parenthetical added for explanation), which may indicate your *critical section* covers *too much* code.
```text
A B C D E F G H I J K L M N O P Q R S T U V W X Y Z
A B C D E F G H I J K L M N O P Q R S T U V W X Y Z
A B C D E F G H I J K L M N O P Q R S T U V W X Y Z
A B C D E F G H I J K L M N O P Q R S T U V W X Y Z
A B C D E F G H I J K L M N O P Q R S T U V W X Y Z
(letter lines repeat a total of 50,000 times)
0 1 2 3 4 5 6 7 8 9
0 1 2 3 4 5 6 7 8 9
0 1 2 3 4 5 6 7 8 9
0 1 2 3 4 5 6 7 8 9
0 1 2 3 4 5 6 7 8 9
(number lines repeat a total of 50,000 times)
```
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- When you compile and run packaged software from an IDE, the execution process can be as easy as clicking a run icon, as the IDE will maintain the classpath for you and will also let you know if anything is out of sorts. When you try to compile and interpret the code yourself from the command line, you will need to know exactly how to path your files. Let us start from c:\Code directory for this assignment. Consider a java file who's .class will result in the com.CITC1318.course package as follows: package com.CITC1318.course; public class GreetingsClass { public static void main(String[] args) { System.out.println("$ Greetings, CITC1318!"); } } This exercise will have you compiling and running the application with new classes created in a separate package: 1. Compile the program: c:\Code>javac -d . GreetingsClass.java 2. Run the program to ensure it is error-free: c:\Code>java -cp . com.CITC1318.course.GreetingsClass 3. Create three classes named Chapter1, Chapter2, and Chapter3…arrow_forwardMake use of a random number generator to generate a list of 500 three-digit numbers. Create a sequential list FILE of the 500 numbers. Artificially implement storage blocks on the sequential list with every block containing a maximum of 10 numbers only. Open an index INDX over the sequential list FILE which records the highest key in each storage block and the address of the storage block. Implement Indexed Sequential search to look for keys K in FILE. Compare the number of comparisons made by the search with that of the sequential search for the same set of keys.Extend the implementation to include an index over the index INDX.arrow_forwardin java Variables bananaStream and bananaDataFS are FileOutputStream and PrintWriter, respectively. String bananaDataName is assigned a file's name read from input. Perform the following tasks: Assign bananaStream with a new FileOutputStream that opens bananaDataName for writing. Assign bananaDataFS with a new PrintWriter created using bananaStream. Ex: If the input is note.txt Ina, then note.txt contains: Ina ordered bananas 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 public class BananaDataProcessor { publicstaticvoidmain(String[] args) throwsIOException { Scannerscnr=newScanner(System.in); StringbananaDataName; StringbananaPurchase; FileOutputStreambananaStream=null; PrintWriterbananaDataFS=null; bananaDataName=scnr.next(); /* Your code goes here */ bananaPurchase=scnr.next(); bananaDataFS.println(bananaPurchase+" ordered bananas"); bananaDataFS.close(); } }arrow_forward
- in C++ answer the following question in the image :arrow_forwardneed code for this in python or java thanksarrow_forwardIn UNIX, how would you remove the directory test and all files and subdirectories (recursively) in it? The directory test is located in (is a subdirectory of) /home/myid/smith/projects. O rmdir /home/myid/smith/projects/test O rmdir -r /home/myid/smith/projects/test rm -r /home/myid/smith/projects/test O rm -r projects/testarrow_forward
- Need help to implement networking in python Server.py Load data from file This option will ask the user for a fully qualified path and will load a JSON file containing the information for users and pending messages. The format of the JSON file will be defined based on your implementation and it will the same used to save the data into file (menu option #4). Functionalities the server must provide: 1) User Sign Up: adds a user identified by a phone number to the system. a) Protocol: USR|username|password|display_name b) Response: 0|OK for success. 1| for an error. c) The server should validate that the username does not exist 2) User Sign In: verify user credentials a) Protocol: LOG|username|password b) Response: i) 0|OK → the credentials are correct. ii) 1|Invalid Credentials iii) 2|Already Logged In → in case that the user is logged in from another client. c) This will help the server knowing which user is connected. d) The server should keep a list of connected clients, which should…arrow_forwardImplement a simple version of the linux grep command in C++. grep - Looks through a file, line by line, trying to find a user-specified search term in the line. If a line has the word within it, the line is printed out, otherwise it is not. Use the system calls open(), getline(), close(). Requirements (examples run from. terminal) Your program grep is always passed a search term and zero or more files to grep through (thus, more than one is possible). It should go through each line and see if the search term is in it; if so, the line should be printed, and if not, the line should be skipped. [terminal]$ ./grep ! main.cpp main2.cppcout << "Hello, World!";cout << "Programming is great fun!"; The matching is case sensitive. Thus, if searching for world, lines with World will not match. Lines can be arbitrarily long (that is, you may see many many characters before you encounter a newline character, \n). grep should work as expected even with very long lines. For this,…arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
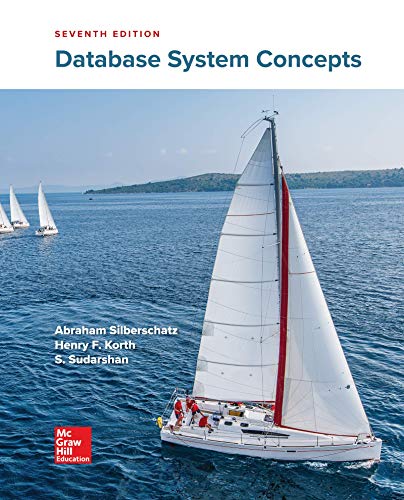
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
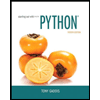
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
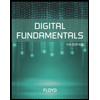
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
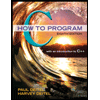
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
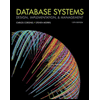
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
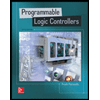
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education