I need to add a line that prints "Not a legal move!" if the user enters something that isn't R, P or S and have the game continue. Here is my code. #include #include //declare variables int main() { int randSeed; int numGames; int userScore=0; int compScore=0; char userChoice; int tieScore=0; char comp; //welcome and user input printf("Starting the CPSC 1011 Rock, Paper, Scissors Game!\n\n"); printf("Enter a random seed between 0 - 100:"); scanf("%d", &randSeed); srand(randSeed); printf("\nEnter the number of matches to play: "); scanf("%d", &numGames); //loop for number of games and letter check for(int i=0; i0){ printf(" You-%d ", userScore); } if(compScore>0){ printf(" Computer-%d ", compScore); } if(tieScore>0){ printf("Ties-%d\n", tieScore); } } //final add up of scores and totals printf("\nThe game of %d matches is complete. The final scores are:\n", numGames); printf("You: %d\n", userScore); printf("Computer: %d\n", compScore); printf("Ties: %d \n", tieScore); return(0); }
I need to add a line that prints "Not a legal move!" if the user enters something that isn't R, P or S and have the game continue. Here is my code.
#include <stdio.h>
#include <stdlib.h>
//declare variables
int main() {
int randSeed;
int numGames;
int userScore=0;
int compScore=0;
char userChoice;
int tieScore=0;
char comp;
//welcome and user input
printf("Starting the CPSC 1011 Rock, Paper, Scissors Game!\n\n");
printf("Enter a random seed between 0 - 100:");
scanf("%d", &randSeed);
srand(randSeed);
printf("\nEnter the number of matches to play: ");
scanf("%d", &numGames);
//loop for number of games and letter check
for(int i=0; i<numGames; i++) {
printf("\n\tMatch %d: Enter R for rock, P for paper, or S for scissors: ",(i+1));
while(1){
scanf("%c", &userChoice);
if((userChoice=='R')|| (userChoice=='P')|| (userChoice=='S')){
break; }
}
//computer selection
comp="RPS"[random()%3];
// scoring
if(userChoice=='R'){
if(comp=='R'){
printf("\tThe computer chose rock. You tied.");
tieScore++;
}
if(comp=='P'){
printf("\tThe computer chose paper. You lose.");
compScore++;
}
if(comp=='S'){
printf("\tThe computer chose scissors. You win!");
userScore++;
}
} else if(userChoice=='P'){
if(comp=='R'){
printf("\tThe computer chose rock. You win!");
userScore++;
}
if(comp=='P'){
printf("\tThe computer chose paper. You tied.");
tieScore++;
}
if(comp=='S'){
printf("\tThe computer chose scissors. You lose.");
compScore++;
}
}
if(userChoice=='S'){
if(comp=='R'){
printf("\tThe computer chose rock. You lose.");
compScore++;
}
if(comp=='P'){
printf("\tThe computer chose paper. You win!");
userScore++;
}
if(comp=='S'){
printf("\tThe computer chose scissors. You tied.");
tieScore++;
}
}
// add up of scores after each round
printf("\n\tScores: ");
if(userScore>0){
printf(" You-%d ", userScore);
}
if(compScore>0){
printf(" Computer-%d ", compScore);
}
if(tieScore>0){
printf("Ties-%d\n", tieScore);
}
}
//final add up of scores and totals
printf("\nThe game of %d matches is complete. The final scores are:\n", numGames);
printf("You: %d\n", userScore);
printf("Computer: %d\n", compScore);
printf("Ties: %d \n", tieScore);
return(0);
}

There is an extra case need to be added and below is the fixed code
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

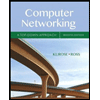
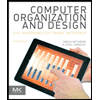
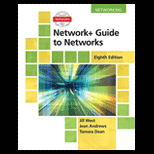
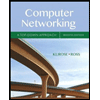
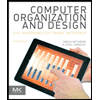
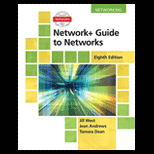
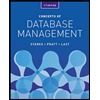
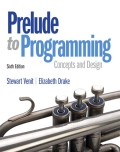
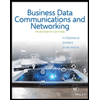