I need to create an UML digram from the following code: import java.util.Scanner; //Code Provided For Project Two. class Main { public static void main(String[] args) { Scanner input = new Scanner(System.in); System.out.print("Enter the first complex number: "); double a = input.nextDouble(); double b = input.nextDouble(); Complex c1 = new Complex(a, b); System.out.print("Enter the second complex number: "); double c = input.nextDouble(); double d = input.nextDouble(); Complex c2 = new Complex(c, d); System.out.println("(" + c1 + ")" + " + " + "(" + c2 + ")" + " = " + c1.add(c2)); System.out.println("(" + c1 + ")" + " - " + "(" + c2 + ")" + " = " + c1.subtract(c2)); System.out.println("(" + c1 + ")" + " * " + "(" + c2 + ")" + " = " + c1.multiply(c2)); System.out.println("(" + c1 + ")" + " / " + "(" + c2 + ")" + " = " + c1.divide(c2)); System.out.println("|" + c1 + "| = " + c1.abs()); Complex c3 = null; try { c3 = (Complex)c1.clone(); } catch (CloneNotSupportedException e) { e.printStackTrace(); } System.out.println(c1 == c3); System.out.println(c3.getRealPart()); System.out.println(c3.getImaginaryPart()); Complex c4 = new Complex(4, -0.5); Complex[] list = {c1, c2, c3, c4}; java.util.Arrays.sort(list); System.out.println(java.util.Arrays.toString(list)); } } //class complex class complex{ //Design a class named Complex class Complex implements Cloneable { private double a; private double b; public Complex(double a, double b) { this.a = a; this.b = b; } public Complex(double a) { this.a = a; b = 0; } public Complex() { this.a = 0; this.b = 0; } public double getRealPart() { return this.a; } public double getImaginaryPart() { return this.b; } public String toString() { if (b < 0) { return this.a + " " + this.b + "i"; } else if (b > 0) { return this.a + " + " + this.b + "i"; } else { return String.valueOf(this.a); } } public Object clone() throws CloneNotSupportedException { return super.clone(); } //Methods adition //subtract public Complex subtract(Complex c) { double real = this.a - c.getRealPart(); double imaginary = this.b - c.getImaginaryPart(); return new Complex(real, imaginary); } //add public Complex add(Complex c) { double real = this.a + c.getRealPart(); double imaginary = this.b + c.getImaginaryPart(); return new Complex(real, imaginary); } //Division public Complex divide(Complex c) { double real = this.a / c.getRealPart(); double imaginary = this.b / c.getImaginaryPart(); return new Complex(real, imaginary); } //multiplication public Complex multiply(Complex c) { double real = this.a * c.getRealPart(); double imaginary = this.b * c.getImaginaryPart(); return new Complex(real, imaginary); } public Complex abs() { double real = this.a < 0 ? -this.a : this.a; double imaginary = this.b < 0 ? -this.b : this.b; return new Complex(real, imaginary); } } }
I need to create an UML digram from the following code:
import java.util.Scanner;
//Code Provided For Project Two.
class Main {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.print("Enter the first complex number: ");
double a = input.nextDouble();
double b = input.nextDouble();
Complex c1 = new Complex(a, b);
System.out.print("Enter the second complex number: ");
double c = input.nextDouble();
double d = input.nextDouble();
Complex c2 = new Complex(c, d);
System.out.println("(" + c1 + ")" + " + " + "(" + c2 + ")" + " = " + c1.add(c2));
System.out.println("(" + c1 + ")" + " - " + "(" + c2 + ")" + " = " + c1.subtract(c2));
System.out.println("(" + c1 + ")" + " * " + "(" + c2 + ")" + " = " + c1.multiply(c2));
System.out.println("(" + c1 + ")" + " / " + "(" + c2 + ")" + " = " + c1.divide(c2));
System.out.println("|" + c1 + "| = " + c1.abs());
Complex c3 = null;
try {
c3 = (Complex)c1.clone();
}
catch (CloneNotSupportedException e) {
e.printStackTrace();
}
System.out.println(c1 == c3);
System.out.println(c3.getRealPart());
System.out.println(c3.getImaginaryPart());
Complex c4 = new Complex(4, -0.5);
Complex[] list = {c1, c2, c3, c4};
java.util.Arrays.sort(list);
System.out.println(java.util.Arrays.toString(list));
}
}
//class complex
class complex{
//Design a class named Complex
class Complex implements Cloneable {
private double a;
private double b;
public Complex(double a, double b) {
this.a = a;
this.b = b;
}
public Complex(double a) {
this.a = a;
b = 0;
}
public Complex() {
this.a = 0;
this.b = 0;
}
public double getRealPart() {
return this.a;
}
public double getImaginaryPart() {
return this.b;
}
public String toString() {
if (b < 0) {
return this.a + " " + this.b + "i";
} else if (b > 0) {
return this.a + " + " + this.b + "i";
} else {
return String.valueOf(this.a);
}
}
public Object clone() throws CloneNotSupportedException {
return super.clone();
}
//Methods adition
//subtract
public Complex subtract(Complex c) {
double real = this.a - c.getRealPart();
double imaginary = this.b - c.getImaginaryPart();
return new Complex(real, imaginary);
}
//add
public Complex add(Complex c) {
double real = this.a + c.getRealPart();
double imaginary = this.b + c.getImaginaryPart();
return new Complex(real, imaginary);
}
//Division
public Complex divide(Complex c) {
double real = this.a / c.getRealPart();
double imaginary = this.b / c.getImaginaryPart();
return new Complex(real, imaginary);
}
//multiplication
public Complex multiply(Complex c) {
double real = this.a * c.getRealPart();
double imaginary = this.b * c.getImaginaryPart();
return new Complex(real, imaginary);
}
public Complex abs() {
double real = this.a < 0 ? -this.a : this.a;
double imaginary = this.b < 0 ? -this.b : this.b;
return new Complex(real, imaginary);
}
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

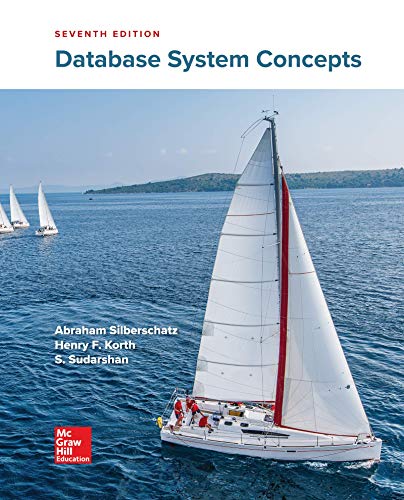
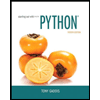
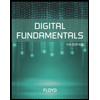
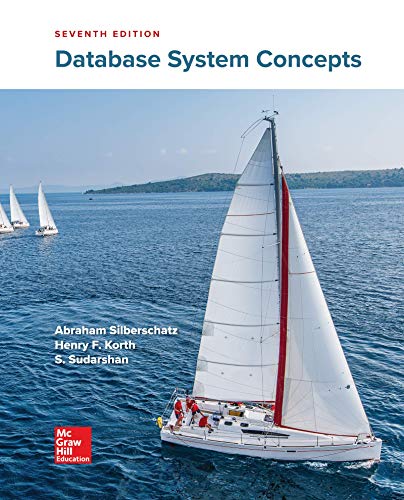
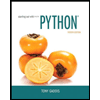
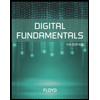
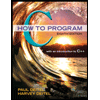
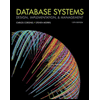
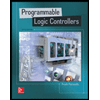