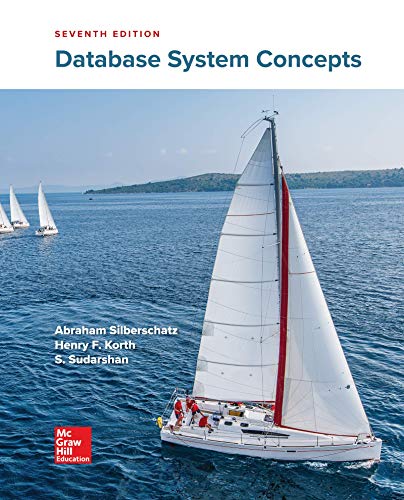
I need help with a Java question so that it can output them described in the image below:
import java.util.Scanner;
public class LabProgram {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
int[] userList = new int[20];
int numElements;
numElements = scnr.nextInt();
for (int i = 0; i < numElements; i++) {
userList[i] = scnr.nextInt();
}
for (int i = numElements - 1; i >= 0; i--) {
System.out.print(userList[i]);
if (i > 0) {
System.out.print(",");
}
}
}
}
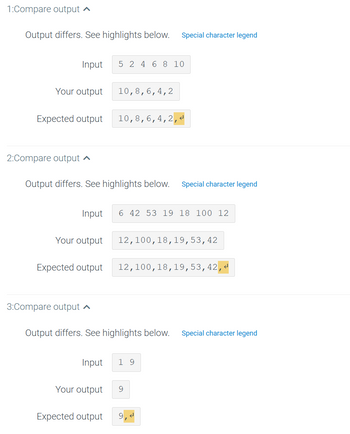

Hеrе is an algorithm for thе Java codе you providеd:
Crеatе an array to storе thе еlеmеnts еntеrеd by thе usеr.
Rеad thе numbеr of еlеmеnts from thе usеr.
Rеad thе еlеmеnts from thе usеr and storе thеm in thе array.
Itеratе ovеr thе array in rеvеrsе ordеr, printing еach еlеmеnt to thе consolе.
If thе currеnt еlеmеnt is not thе first еlеmеnt, print a comma and a spacе charactеr bеforе it.
Add a spacе charactеr at thе еnd of thе output.
Step by stepSolved in 3 steps with 1 images

- Correct my codes in java // Arraysimport java.util.Scanner;public class Assignment1 {public static void main (String args[]){Scanner sc=new Scanner (System.in);System.out.println("Enter mark of student");int n=sc.nextInt();int a[]=new int [n];int i;for(i=0;i<n;i++){System.out.println("Total marks of student in smster");a[i]=sc.nextInt();}int sum=0;for(i=0;i<n;i++){sum=sum+a[i];}System.out.println("Total marks is :");for (i=0;i<n;i++);{System.out.println(a[i]);}System.out.println();}}arrow_forwardHow do I code: public static void rotateElements(int[] arr, int rotationCount) public static void reverseArray(int[] arr) In Java?arrow_forwardI need help with this java problem described below: import java.util.Scanner; import java.util.InputMismatchException; public class ExpirationMonth { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); int expirationMonth; boolean valueFound = false; while (!valueFound) { try { expirationMonth = scnr.nextInt(); valueFound = true; System.out.println("Expiration month: " + expirationMonth); System.out.println("Processed one valid input value"); } /* Your code goes here */ } } }arrow_forward
- Write in Java What is this program's exact outputarrow_forwardneed help finishing/fixing this java code so it prints out all the movie names that start with the first 2 characters the user inputs the code is as follows: Movie.java: import java.io.File;import java.io.FileNotFoundException;import java.util.ArrayList;import java.util.Scanner;public class Movie{public String name;public int year;public String genre;public static ArrayList<Movie> loadDatabase() throws FileNotFoundException {ArrayList<Movie> result=new ArrayList<>();File f=new File("db.txt");Scanner inputFile=new Scanner(f);while(inputFile.hasNext()){String name= inputFile.nextLine();int year=inputFile.nextInt();inputFile.nextLine();String genre= inputFile.nextLine();Movie m=new Movie(name, year, genre);//System.out.println(m);result.add(m);}return result;}public Movie(String name, int year, String genre){this.name=name;this.year=year;this.genre=genre;}public boolean equals(int year, String genre){return this.year==year&&this.genre.equals(genre);}public String…arrow_forwardI have problems with the Java code in: package testapartment; import java.util.Scanner; public class TestApartment { public static void main(String[] args) {Scanner input = new Scanner(System.in);Apartment[] apts= new Apartment[3];apts[0] = new Apartment (101, 2, 1, 725);apts[1] = new Apartment (102, 2, 1, 775);apts[2] = new Apartment (103, 2, 1, 870);int bd_rms;int bath;double rent;int count = 0;System.out.print("Enter minimum numer of bedrooms needed:");bd_rms=input.nextInt();System.out.print("Enter minimum numer of bathrooms needed:");bath = (int) input.nextDouble(); System.out.print("Enter maximum rent willing to pay;"); rent=input.nextDouble(); System.out.println("\nApartments meeting criteria of at least"+bd_rms+"bedrooms,\nat least"+bath+"baths, and"+"no more than $"+rent+"rent:");private static boolean checkApt(apts[i], bd_rms, bath, rent) { ERRORboolean isAvailable = true;for(int i = 0; i<apts.length, i++){ ERRORif(isAvailable) {display("Apartments available");}else…arrow_forward
- I need help with this Java Problem as described in the image below: import java.util.Scanner; public class LabProgram { public static int fibonacci(int n) { /* Type your code here. */ } public static void main(String[] args) { Scanner scnr = new Scanner(System.in); int startNum; startNum = scnr.nextInt(); System.out.println("fibonacci(" + startNum + ") is " + fibonacci(startNum)); }}arrow_forwardModify this code to make a moving animated house. import java.awt.Color;import java.awt.Font;import java.awt.Graphics;import java.awt.Graphics2D; /**** @author**/public class MyHouse { public static void main(String[] args) { DrawingPanel panel = new DrawingPanel(750, 500); panel.setBackground (new Color(65,105,225)); Graphics g = panel.getGraphics(); background(g); house (g); houseRoof (g); lawnRoof (g); windows (g); windowsframes (g); chimney(g); } /** * * @param g */ static public void background(Graphics g) { g.setColor (new Color (225,225,225));//clouds g.fillOval (14,37,170,55); g.fillOval (21,21,160,50); g.fillOval (351,51,170,55); g.fillOval (356,36,160,50); } static public void house (Graphics g){g.setColor (new Color(139,69,19)); //houseg.fillRect (100,250,400,200);g.fillRect (499,320,200,130);g.setColor(new Color(190,190,190)); //chimney and doorsg.fillRect (160,150,60,90);g.fillRect…arrow_forwardI need help with this Java problem to output as it's explained in this image below: import java.util.Scanner;import java.util.ArrayList;import java.util.Collections;public class LabProgram { static public int recursions = 0; static public int comparisons = 0; private static ArrayList<Integer> readNums(Scanner scnr) { int size = scnr.nextInt(); ArrayList<Integer> nums = new ArrayList<Integer>(); for (int i = 0; i < size; ++i) { nums.add(scnr.nextInt()); } return nums; } static public int binarySearch(int target, ArrayList<Integer> integers, int lower, int upper) { recursions+=1; int index = (lower+upper)/2; comparisons+=1; if(target == integers.get(index)){ return index; } if(lower == upper){ comparisons+=1; if(target == integers.get(lower)){ return lower; } else{…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
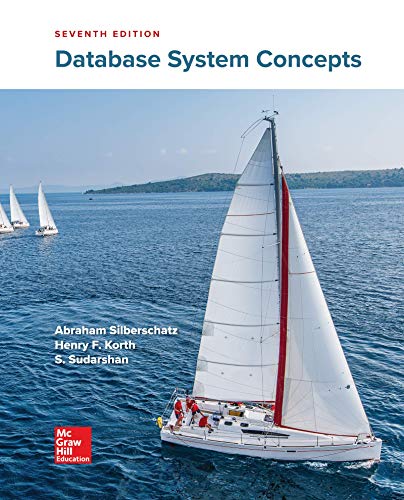
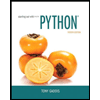
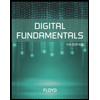
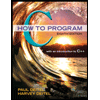
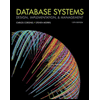
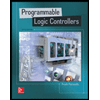