Java Code: In this assignment, we are going to start working on the parser. The lexer’s job is to make “words”, the parser’s job is to make sure that those tokens are in an order that makes sense and create a data structure in memory that represents the program. The Tokens are in a 1-dimensional structure (array or list). The parser’s output will be a 2-dimensional tree. The parser works using recursive descent. We are encoding the rules into the structure of the program. There are a few simple rules that we will follow in writing our parser: Each function represents some “phrase” in our language, like “if statement” or “assignment statement”. Each function must either succeed or fail: On success, remove Tokens from the list and output a tree node On failure, leave the list unchanged and return null. When there are alternatives, the function must call each alternative’s function until it finds one that is not null. Create a Parser class (does not derive from anything). It must have a constructor that accepts your collection of Tokens. We will be treating the collection of tokens as a queue – taking off the front. It isn’t necessary to use a Java Queue, but you may. We will add three helper functions to parser. These should be private: matchAndRemove – accepts a token type. Looks at the next token in the collection: If the passed in token type matches the next token’s type, remove that token and return it. If the passed in token type DOES NOT match the next token’s type (or there are no more tokens) return null. expectEndsOfLine – uses matchAndRemove to match and discard one or more ENDOFLINE tokens. Throw a SyntaxErrorException if no ENDOFLINE was found. peek – accepts an integer and looks ahead that many tokens and returns that token. Returns null if there aren’t enough tokens to fulfill the request. Create a public parse method. There are no parameters, and it returns Node. This will be called from main once lexing is complete. For now, we are going to only parse a subset of Shank V2 – mathematical expressions. Parse should call expression() then expectEndOfLine() in a loop until either returns null. Don’t worry about storing the return node but you should print it out (using ToString()) for testing. Make sure to use various test cases and provide scree
Java Code:
In this assignment, we are going to start working on the parser. The lexer’s job is to make “words”, the parser’s job is to make sure that those tokens are in an order that makes sense and create a data structure in memory that represents the program. The Tokens are in a 1-dimensional structure (array or list). The parser’s output will be a 2-dimensional tree.
The parser works using recursive descent. We are encoding the rules into the structure of the program. There are a few simple rules that we will follow in writing our parser:
- Each function represents some “phrase” in our language, like “if statement” or “assignment statement”.
- Each function must either succeed or fail:
- On success, remove Tokens from the list and output a tree node
- On failure, leave the list unchanged and return null.
- When there are alternatives, the function must call each alternative’s function until it finds one that is not null.
Create a Parser class (does not derive from anything). It must have a constructor that accepts your collection of Tokens. We will be treating the collection of tokens as a queue – taking off the front. It isn’t necessary to use a Java Queue, but you may.
We will add three helper functions to parser. These should be private:
matchAndRemove – accepts a token type. Looks at the next token in the collection:
If the passed in token type matches the next token’s type, remove that token and return it.
If the passed in token type DOES NOT match the next token’s type (or there are no more tokens) return null.
expectEndsOfLine – uses matchAndRemove to match and discard one or more ENDOFLINE tokens. Throw a SyntaxErrorException if no ENDOFLINE was found.
peek – accepts an integer and looks ahead that many tokens and returns that token. Returns null if there aren’t enough tokens to fulfill the request.
Create a public parse method. There are no parameters, and it returns Node. This will be called from main once lexing is complete. For now, we are going to only parse a subset of Shank V2 – mathematical expressions. Parse should call expression() then expectEndOfLine() in a loop until either returns null. Don’t worry about storing the return node but you should print it out (using ToString()) for testing.
Make sure to use various test cases and provide screenshots of the test cases being tested for the code. There must be no error in the code at all.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

I ran the code and got an error. I even created a main.java file to run the test cases. Please fix the error and provide me the correct code for all parts. Make sure to give the screenshot of the output as well.
![Parser.java: Lexer.java
1- public class main {
2
3- public static void main(String[] args) {
4
// Test cases
5
6
7
Lexer lexer = new Lexer("2 + 3 * 4 * 5");
List<Token > tokens = lexer.lex();
Parser parser = new Parser (tokens);
Node parsedTree = parser.parse();
System.out.println(printTree(parsedTree));
8
9
10
11
12
13
14
15
16
A
}
public static String print Tree (Node node) {
if (node == null) {
return "";
Compilation failed due to following error(s).
Main.java:1: error: class main is public, should be declared in a file named main.java
public class main {
^
}
if (node.getLeft() == null && node.getRight()
return node.getValue();
input
Parser.java:10: error: cannot find symbol
private List<Token> tokens;
Token.java Node.java Main.java ⠀
symbol: class List
location: class Parser
Parser.java:13: error: cannot find symbol
public Parser(List<Token> tokens) {
A
symbol: class List
location: class Parser
^
Lexer. java:69: error: cannot find symbol
public List<Token> lex() {
symbol: class List
== null) {
stderr](https://content.bartleby.com/qna-images/question/e7ddc10c-4670-40fd-b02c-6a60c5fcc2f2/da25bf5f-cffe-4460-93bd-36026e0054fa/2b0x5c_thumbnail.png)
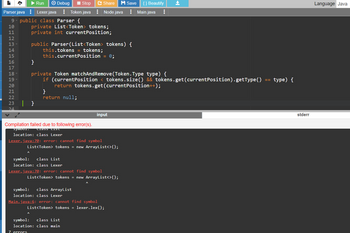
I ran the code and got an error. Please fix the error and provide me the correct code for all parts. Make sure to give the screenshot of the output as well.
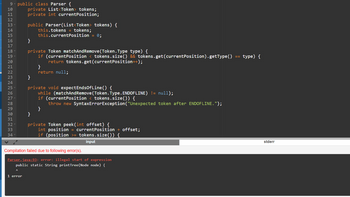
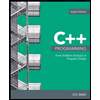
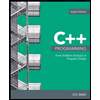