ify your program so every data entry statement uses a TryParse() method to ensure that each piece of data is the correct type. using System; using static System.Console; using System.Globalization; class GreenvilleRevenue { static void Main() { const int ENTRANCE_FEE = 25;
ify your program so every data entry statement uses a TryParse() method to ensure that each piece of data is the correct type. using System; using static System.Console; using System.Globalization; class GreenvilleRevenue { static void Main() { const int ENTRANCE_FEE = 25;
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter11: Inheritance And Composition
Section: Chapter Questions
Problem 6PE
Related questions
Question
modify your program so every data entry statement uses a TryParse() method to ensure that each piece of data is the correct type.
using System;
using static System.Console;
using System.Globalization;
class GreenvilleRevenue
{
static void Main()
{
const int ENTRANCE_FEE = 25;
const int MIN_CONTESTANTS = 0;
const int MAX_CONTESTANTS = 30;
int numThisYear;
int numLastYear;
int revenue;
string[] names = new string[MAX_CONTESTANTS];
char[] talents = new char[MAX_CONTESTANTS];
char[] talentCodes = { 'S', 'D', 'M', 'O' };
string[] talentCodesStrings = { "Singing", "Dancing", "Musical instrument", "Other" };
int[] counts = { 0, 0, 0, 0 }; ;
numLastYear = GetContestantNumber("last", MIN_CONTESTANTS, MAX_CONTESTANTS);
numThisYear = GetContestantNumber("this", MIN_CONTESTANTS, MAX_CONTESTANTS);
revenue = numThisYear * ENTRANCE_FEE;
WriteLine("Last year's competition had {0} contestants, and this year's has {1} contestants",
numLastYear, numThisYear);
WriteLine("Revenue expected this year is {0}", revenue.ToString("C", CultureInfo.GetCultureInfo("en-US")));
DisplayRelationship(numThisYear, numLastYear);
GetContestantData(numThisYear, names, talents, talentCodes, talentCodesStrings, counts);
GetLists(numThisYear, talentCodes, talentCodesStrings, names, talents, counts);
}
public static int GetContestantNumber(string when, int min, int max)
{
string entryString;
int num;
do
{
Console.Write("Enter number of contestants {0} year >> ", when);
entryString = ReadLine();
bool success = Int32.TryParse(entryString, out num);
if (success)
{
if (num < min || num > max)
WriteLine("Number must be between {0} and {1}", min, max);
}
else
{
WriteLine("Invalid format - entry must be a single character");
WriteLine("That is not a valid code");
}
} while (num < min || num > max);
return num;
}
public static void DisplayRelationship(int numThisYear, int numLastYear)
{
if (numThisYear > 2 * numLastYear)
WriteLine("The competition is more than twice as big this year!");
else
if (numThisYear > numLastYear)
WriteLine("The competition is bigger than ever!");
else
if (numThisYear < numLastYear)
WriteLine("A tighter race this year! Come out and cast your vote!");
}
public static void GetContestantData(int numThisYear, string[] names, char[] talents, char[] talentCodes, string[] talentCodesStrings, int[] counts)
{
int x = 0;
bool isValid;
while (x < numThisYear)
{
Console.Write("Enter contestant name >> ");
names[x] = ReadLine();
WriteLine("Talent codes are:");
for (int y = 0; y < talentCodes.Length; ++y)
WriteLine(" {0} {1}", talentCodes[y], talentCodesStrings[y]);
while(true)
{
Console.Write(" Enter talent code >> ");
bool success=Char.TryParse(ReadLine(), out talents[x]);
if (!success)
WriteLine("Invalid format - entry must be a single character");
else
break;
}
isValid = false;
while (!isValid)
{
for (int z = 0; z < talentCodes.Length; ++z)
{
if (talents[x] == talentCodes[z])
{
isValid = true;
++counts[z];
}
}
if (!isValid)
{
WriteLine("That is not a valid code", talents[x]);
while (true)
{
Console.Write(" Enter talent code >> ");
bool success = Char.TryParse(ReadLine(), out talents[x]);
if (!success)
WriteLine("Invalid format - entry must be a single character");
else
break;
}
}
}
++x;
}
}
public static void GetLists(int numThisYear, char[] talentCodes, string[] talentCodesStrings, string[] names, char[] talents, int[] counts)
{
int x;
char QUIT = 'Z';
char option;
bool isValid;
int pos = 0;
bool found;
WriteLine("\nThe types of talent are:");
for (x = 0; x < counts.Length; ++x)
WriteLine("{0, -20} {1, 5}", talentCodesStrings[x], counts[x]);
while (true)
{
Console.Write("\nEnter a talent type or {0} to quit >> ", QUIT);
bool success = Char.TryParse(ReadLine(), out option);
if (!success)
WriteLine("Invalid format - entry must be a single character");
else
break;
}
while (option != QUIT)
{
isValid = false;
for (int z = 0; z < talentCodes.Length; ++z)
{
if (option == talentCodes[z])
{
isValid = true;
pos = z;
}
}
if (!isValid)
WriteLine("That is not a valid code", option);
else
{
WriteLine("\nContestants with talent {0} are:", talentCodesStrings[pos]);
found = false;
for (x = 0; x < numThisYear; ++x)
{
if (talents[x] == option)
{
WriteLine(names[x]);
found = true;
}
}
if (!found)
WriteLine("No contestants had talent {0}", talentCodesStrings[pos]);
}
while (true)
{
Console.Write("\nEnter a talent type or {0} to quit >> ", QUIT);
bool success = Char.TryParse(ReadLine(), out option);
if (!success)
WriteLine("Invalid format - entry must be a single character ");
else
break;
}
}
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
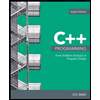
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
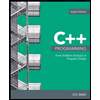
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning