a. (100pts) Write a program to define a class Car according to the following UML. Please note that this is only a definition class, so there is no main method in the class. Car -name: String -mileage: int - isManual: boolean - carAmount: int //Increments by 1 when a new Car is created + Car (name: String, mileage: int, isManual: boolean) + getName(): String + setName (name: String): void + getMileage (): int + setMileage (mileage: int): void +isManual (): boolean + setIsManual (is: boolean): void +getCarAmount(): int b. (100pts) Based on the Car class, write a program named CarTest that completes the following tasks. Create an array with 3 Car objects. Use a for loop to initialize the array using user's inputs for name, mileage and whether the car has a manual transmission for each car. (Hint: use input.nextLine() to receive user's input for car name because car names could consist of more than one word.) Use a for loop to display the cars' names and their mileages in a table format. Use a for loop to calculate the average mileage of all cars. Display the average mileage of the cars. Display how many cars there exists using the getCarAmount () method. A sample execution of the CarTest program is given below: Please enter the car's name: Honda Civic Please enter the car's mileage: 61000 Please enter whether the car is manual (true/false): false Please enter the car's name: Ford Bronco Please enter the car's mileage: 11000 Please enter whether the car is manual (true/false): true
a. (100pts) Write a program to define a class Car according to the following UML. Please note that this is only a definition class, so there is no main method in the class. Car -name: String -mileage: int - isManual: boolean - carAmount: int //Increments by 1 when a new Car is created + Car (name: String, mileage: int, isManual: boolean) + getName(): String + setName (name: String): void + getMileage (): int + setMileage (mileage: int): void +isManual (): boolean + setIsManual (is: boolean): void +getCarAmount(): int b. (100pts) Based on the Car class, write a program named CarTest that completes the following tasks. Create an array with 3 Car objects. Use a for loop to initialize the array using user's inputs for name, mileage and whether the car has a manual transmission for each car. (Hint: use input.nextLine() to receive user's input for car name because car names could consist of more than one word.) Use a for loop to display the cars' names and their mileages in a table format. Use a for loop to calculate the average mileage of all cars. Display the average mileage of the cars. Display how many cars there exists using the getCarAmount () method. A sample execution of the CarTest program is given below: Please enter the car's name: Honda Civic Please enter the car's mileage: 61000 Please enter whether the car is manual (true/false): false Please enter the car's name: Ford Bronco Please enter the car's mileage: 11000 Please enter whether the car is manual (true/false): true
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter13: Overloading And Templates
Section: Chapter Questions
Problem 38SA
Related questions
Question
100%
I am struggling with creating arrays program on eclipse. Struggling with getting the result as asked in assignment. Need help with problem a and b. Please help as soon as you can.
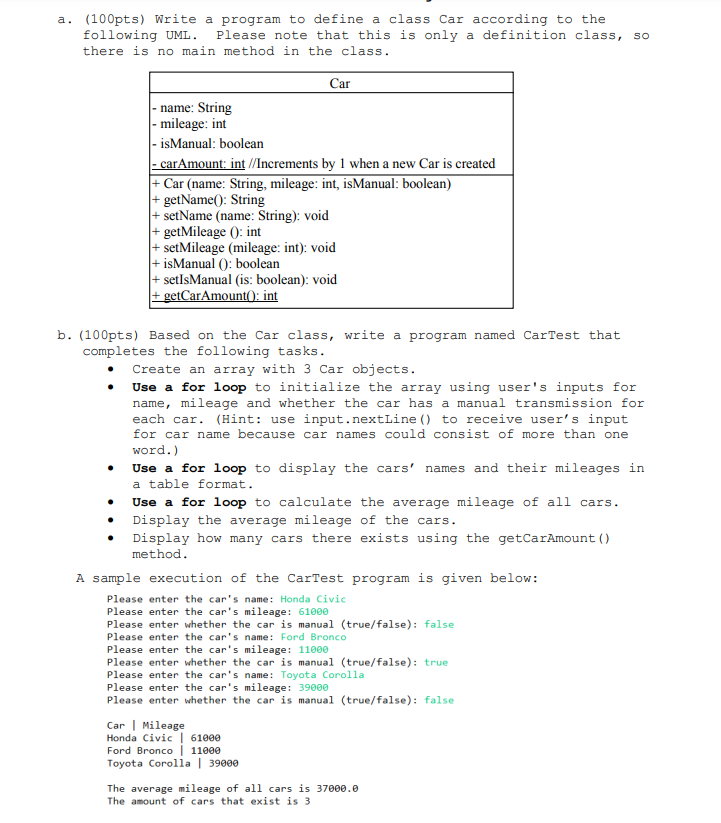
Transcribed Image Text:a program to define a class Car according to the
a. (100pts) Write
following UML.
Please note that this is only a definition class, so
there is no main method in the class.
Car
-name: String
mileage: int
- isManual: boolean
- carAmount: int //Increments by 1 when a new Car is created
+ Car (name: String, mileage: int, isManual: boolean)
+ getName(): String
+ setName (name: String): void
+ getMileage (): int
+ setMileage (mileage: int): void
+ isManual (): boolean
+ setIsManual (is: boolean): void
+getCarAmount(): int
b. (100pts) Based on the Car class, write a program named CarTest that
completes the following tasks.
Create an array with 3 Car objects.
Use a for loop to initialize the array using user's inputs for
name, mileage and whether the car has a manual transmission for
each car. (Hint: use input.nextLine () to receive user's input
for car name because car names could consist of more than one
word.)
Use a for loop to display the cars' names and their mileages in
a table format.
Use a for loop to calculate the average mileage of all cars.
Display the average mileage of the cars.
Display how many cars there exists using the getCarAmount ()
method.
A sample execution of the CarTest program is given below:
Please enter the car's name: Honda Civic
Please enter the car's mileage: 61000
Please enter whether the car is manual (true/false): false
Please enter the car's name: Ford Bronco
Please enter the car's mileage: 11000
Please enter whether the car is manual (true/false): true
Please enter the car's name: Toyota Corolla
Please enter the car's mileage: 39000
Please enter whether the car is manual (true/false): false
Car | Mileage
Honda Civic | 61000
Ford Bronco
11000
Toyota Corolla | 39000
The average mileage of all cars is 37000.0
The amount of cars that exist is 3
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
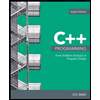
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
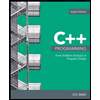
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning