* iii. This program calculates the driving skill and points of two players in a driving game. * */ import java.util.Scanner; public class DriverGame { String player1, player2; //player names double points1=0, points2 = 0;//points achieved double driving1=0, driving2=0; //driving skill score public DriverGame() { inputPlayerNames(); //call method to input the names driving1= calculateDrivingSkill(player1); //store the first player's driving skill value driving2= calculateDrivingSkill(player2); //store the second player's driving skill value calculateAndDisplayPoints(); //calculate and display the points achieved by each player } public void inputPlayerNames() { Scanner scan = new Scanner(System.in); /** ADD CODE HERE * 1. Ask user to input first player name * 2. Ask user to input second name keeping user trapped until both player names are different. */ } public double calculateDrivingSkill(String name) { Scanner scan = new Scanner(System.in); int jump=0, accidents=0; double skillScore=10; /** each player is initally given a starting score of 10 */ System.out.print("How many stunt jumps has " + name + " successfully performed?"); /** ADD CODE HERE * 1. Ask user to input the nuumber of jumps this player has performed * 2. Ask user to input the number of accidents this player has had * 3. Update the player's skill score by * 3.1 For every jump, the skill score is increased by 5% of the current skill score (see exam paper for an example of this) * 3.2 If there have been no accidents, the skill score is increased by 100 * 3.3 If there have been accidents, the player's skill score is decreased by 50 multipled by the number of accidents (this * may result in a negative score). * 4. The skill score for this player is returned */ return 0; /** YOU CAN DELETE THIS LINE WHEN YOU'VE WRITTEN YOUR CODE. IT IS ONLY HERE TO ENSURE PROGRAM COMPILES */ } public String bestPlayer() { /** ADD CODE HERE 1. Compare the points achieved by both players, 1.1 the name of the player with the largest amounts of points is returned. 1.2 If players achieve the same points, an empty string ("") is returned. */ return null; /** YOU CAN DELETE THIS LINE WHEN YOU'VE WRITTEN YOUR CODE. IT IS ONLY HERE TO ENSURE PROGRAM COMPILES */ } public void calculateAndDisplayPoints() { /** ADD CODE HERE 1. Calculate the points achieved by each player - their points achieved is double their driving skill score. Make sure you use variables points1 and point2 declared at the top of the program to store the points. 2. Find out which player is the best player as they also get a bonus set of 50 points (you MUST use bestPlayer() to find the best player). 3. Display the names of each player and their points. */ } public static void main(String[] args) { new DriverGame(); } }
* iii. This program calculates the driving skill and points of two players in a driving game.
*
*/
import java.util.Scanner;
public class DriverGame
{
String player1, player2; //player names
double points1=0, points2 = 0;//points achieved
double driving1=0, driving2=0; //driving skill score
public DriverGame()
{
inputPlayerNames(); //call method to input the names
driving1= calculateDrivingSkill(player1); //store the first player's driving skill value
driving2= calculateDrivingSkill(player2); //store the second player's driving skill value
calculateAndDisplayPoints(); //calculate and display the points achieved by each player
}
public void inputPlayerNames()
{
Scanner scan = new Scanner(System.in);
/** ADD CODE HERE
* 1. Ask user to input first player name
* 2. Ask user to input second name keeping user trapped until both player names are different.
*/
}
public double calculateDrivingSkill(String name)
{
Scanner scan = new Scanner(System.in);
int jump=0, accidents=0;
double skillScore=10; /** each player is initally given a starting score of 10 */
System.out.print("How many stunt jumps has " + name + " successfully performed?");
/** ADD CODE HERE
* 1. Ask user to input the nuumber of jumps this player has performed
* 2. Ask user to input the number of accidents this player has had
* 3. Update the player's skill score by
* 3.1 For every jump, the skill score is increased by 5% of the current skill score (see exam paper for an example of this)
* 3.2 If there have been no accidents, the skill score is increased by 100
* 3.3 If there have been accidents, the player's skill score is decreased by 50 multipled by the number of accidents (this
* may result in a negative score).
* 4. The skill score for this player is returned
*/
return 0; /** YOU CAN DELETE THIS LINE WHEN YOU'VE WRITTEN YOUR CODE. IT IS ONLY HERE TO ENSURE PROGRAM COMPILES */
}
public String bestPlayer()
{
/** ADD CODE HERE
1. Compare the points achieved by both players,
1.1 the name of the player with the largest amounts of points is returned.
1.2 If players achieve the same points, an empty string ("") is returned.
*/
return null; /** YOU CAN DELETE THIS LINE WHEN YOU'VE WRITTEN YOUR CODE. IT IS ONLY HERE TO ENSURE PROGRAM COMPILES */
}
public void calculateAndDisplayPoints()
{
/** ADD CODE HERE
1. Calculate the points achieved by each player - their points achieved is double their driving skill score. Make sure
you use variables points1 and point2 declared at the top of the program to store the points.
2. Find out which player is the best player as they also get a bonus set of 50 points (you MUST use bestPlayer() to find the best player).
3. Display the names of each player and their points.
*/
}
public static void main(String[] args)
{
new DriverGame();
}
}

Step by step
Solved in 6 steps with 11 images

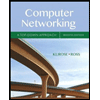
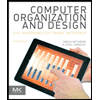
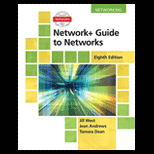
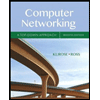
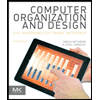
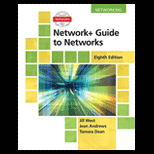
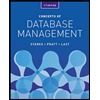
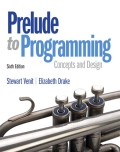
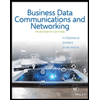