ijava 23 3 public class Paintl { public static void main (String [] args) { Scanner scnr = new Scanner (System.in); double wallHeight = 0.0; double wallWidth = 0.0; double wallArea = 0.0; double gallonsPaintNeeded = 0.0; final double squareFeetPerGallons = 350.0: 12 13 14 15 16 17 18 // Implement a do-while loop to ensure input is valid // Prompt user to input wall's height System.out.println ("Enter wall height (feet): "); wallHeight = scnr.nextDouble (); 19 // Implement a do-while loop to ensure input is valid 20 // Prompt user to input wall's width System.out.println ("Enter wall width (feet) : "); wallWidth = scnr.nextDouble (); // Calculate and output wall area wallArea = wallHeight * wallWidth; System.out.println ("Wall area: " + wallArea + " square feet"); // Calculate and output the amount of paint (in gallons) needed to paint the wall 29 30 31 gallonsPaintNeeded = wallArea/squareFeetPerGallons; System.out.println ("Paint needed: " + gallonsPaintNeeded + " gallons");
ijava 23 3 public class Paintl { public static void main (String [] args) { Scanner scnr = new Scanner (System.in); double wallHeight = 0.0; double wallWidth = 0.0; double wallArea = 0.0; double gallonsPaintNeeded = 0.0; final double squareFeetPerGallons = 350.0: 12 13 14 15 16 17 18 // Implement a do-while loop to ensure input is valid // Prompt user to input wall's height System.out.println ("Enter wall height (feet): "); wallHeight = scnr.nextDouble (); 19 // Implement a do-while loop to ensure input is valid 20 // Prompt user to input wall's width System.out.println ("Enter wall width (feet) : "); wallWidth = scnr.nextDouble (); // Calculate and output wall area wallArea = wallHeight * wallWidth; System.out.println ("Wall area: " + wallArea + " square feet"); // Calculate and output the amount of paint (in gallons) needed to paint the wall 29 30 31 gallonsPaintNeeded = wallArea/squareFeetPerGallons; System.out.println ("Paint needed: " + gallonsPaintNeeded + " gallons");
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
I need help with this Java code, I have already found all the errors and I am getting the correct output. However; in regards to the next part, I have a hard time with loops. I need help creating a Do-while loop to validate all user input and handle exceptions. Please no advanced code, as I am new to Java.
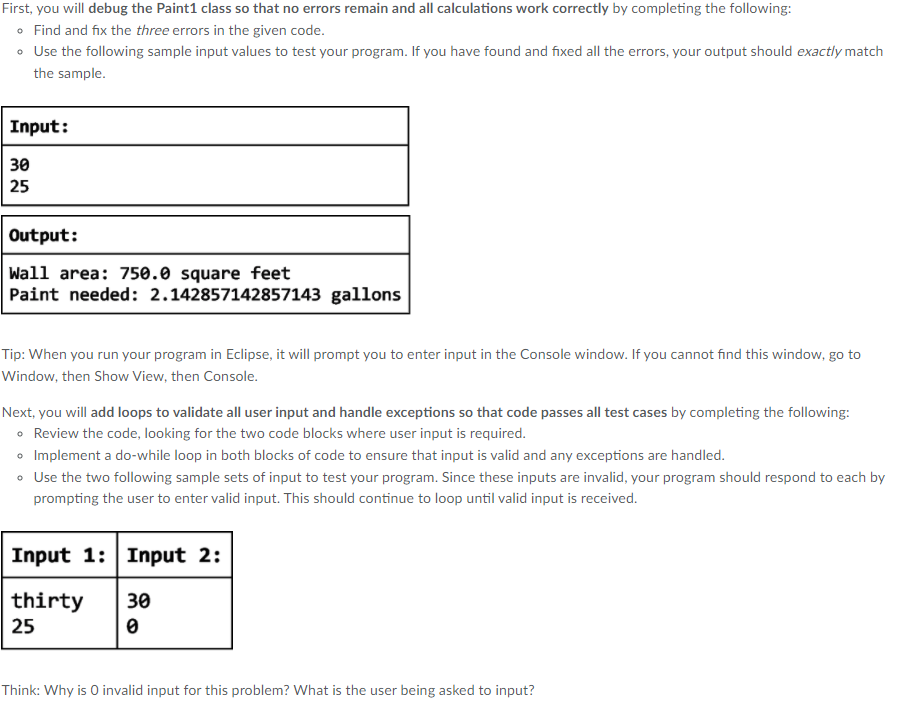
Transcribed Image Text:First, you will debug the Paint1 class so that no errors remain and all calculations work correctly by completing the following:
• Find and fix the three errors in the given code.
• Use the following sample input values to test your program. If you have found and fixed all the errors, your output should exactly match
the sample.
Input:
30
25
Output:
Wall area: 750.0 square feet
Paint needed: 2.142857142857143 gallons
Tip: When you run your program in Eclipse, it will prompt you to enter input in the Console window. If you cannot find this window, go to
Window, then Show View, then Console.
Next, you will add loops to validate all user input and handle exceptions so that code passes all test cases by completing the following:
• Review the code, looking for the two code blocks where user input is required.
o Implement a do-while loop in both blocks of code to ensure that input is valid and any exceptions are handled.
• Use the two following sample sets of input to test your program. Since these inputs are invalid, your program should respond to each by
prompting the user to enter valid input. This should continue to loop until valid input is received.
Input 1: Input 2:
thirty
25
30
Think: Why is O invalid input for this problem? What is the user being asked to input?
![D Paint1.java X
3 public class Paintl {
public static void main (String[] args) {
Scanner scnr = new Scanner (System.in);
double wallHeight = 0.0;
double wallWidth = 0.0;
double wallArea = 0.0;
double gallonsPaintNeeded = 0.0;
final double squareFeetPerGallons = 350.0;
// Implement a do-while loop to ensure input is valid
// Prompt user to input wall's height
System.out.println ("Enter wall height (feet): "):
wallHeight = scnr.nextDouble ();
// Implement a do-while loop to ensure input is valid
// Prompt user to input wall's width
System.out.println ("Enter walli width (feet): ");
wallWidth = scnr.nextDouble ();
// Calculate and output wall area
wallArea = wallHeight * wallWidth;
System.out.println ("Wall area: " + wallArea + " square feet");
// Calculate and output the amount of paint (in gallons) needed to paint the wall
gallonsPaintNeeded = wallArea/squareFeetPerGallons;
System.out.println ("Paint needed: " + gallonsPaintNeeded + " gallons");](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd9fa295a-90dd-4ec6-950d-22c80fce920e%2Fda7bfc60-39cf-4110-b23c-e59d5b9d8b35%2Femi1z7ji_processed.png&w=3840&q=75)
Transcribed Image Text:D Paint1.java X
3 public class Paintl {
public static void main (String[] args) {
Scanner scnr = new Scanner (System.in);
double wallHeight = 0.0;
double wallWidth = 0.0;
double wallArea = 0.0;
double gallonsPaintNeeded = 0.0;
final double squareFeetPerGallons = 350.0;
// Implement a do-while loop to ensure input is valid
// Prompt user to input wall's height
System.out.println ("Enter wall height (feet): "):
wallHeight = scnr.nextDouble ();
// Implement a do-while loop to ensure input is valid
// Prompt user to input wall's width
System.out.println ("Enter walli width (feet): ");
wallWidth = scnr.nextDouble ();
// Calculate and output wall area
wallArea = wallHeight * wallWidth;
System.out.println ("Wall area: " + wallArea + " square feet");
// Calculate and output the amount of paint (in gallons) needed to paint the wall
gallonsPaintNeeded = wallArea/squareFeetPerGallons;
System.out.println ("Paint needed: " + gallonsPaintNeeded + " gallons");
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Recommended textbooks for you
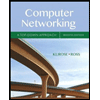
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
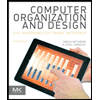
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
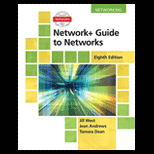
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
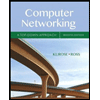
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
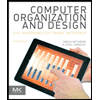
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
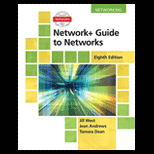
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
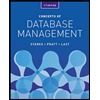
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
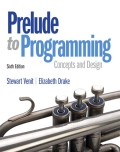
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
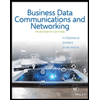
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY