import java.util.Scanner; public class Juice_ordering { public static void main(String [] args) { Food[] foodArray = new Food[4]; foodArray[0] = new Juice("Apple","C01",5.00, "Which is very popular juice in our shop.","Apple",1); foodArray[1] = new Juice("Orange","I02",8.00, "Which is very popular juice in our shop.","Orange",2); foodArray[2] = new Snack("Cheez-It","SC001",15.00, "Which is very popular juice in shop.","tin","small"); foodArray[3] = new Snack("Cheez-It","SC002",18.00, "Nice snack.","tin","medium"); boolean loopagain = true; while(loopagain){ System.out.println("1.\t Display Food"); System.out.println("2.\t Order Food"); System.out.println("3.\t Add Food"); System.out.println("4.\t Delete Food"); System.out.println("0.\t To Exit"); double total=0; Scanner in = new Scanner(System.in); System.out.println("Please enter your choice:"); int choice=in.nextInt(); switch (choice) { case 1: System.out.println("1.\t Display Fruit Juice Order"); System.out.println("2.\t Display Snack Order"); System.out.println("Please enter your choice:"); Scanner on = new Scanner(System.in); int food_choice=on.nextInt(); switch(food_choice){ case 1:displayJuice(foodArray); break; case 2:displaySnack(foodArray); } break; case 2:Scanner ordercode = new Scanner(System.in); System.out.println("Enter the Food Code that wanted to order:"); String code = ordercode.nextLine(); double price=orderprocess(foodArray,code); if (price == -1) System.out.println("Invalid Order Code"); else System.out.println("Price =Rm " +price +"\n"); total+=price; break; case 3:Scanner addcode = new Scanner(System.in); System.out.println("Enter the Food Code that wanted to add order:"); String add = addcode.nextLine(); double addprice=addorderprocess(foodArray,add); if (addprice == -1) System.out.println("Invalid Order Code"); else System.out.println("Price =Rm " +addprice +"\n"); total+=addprice; break; case 4: Scanner dltcode = new Scanner(System.in); System.out.println("Enter the Food Code that wanted to delete order:"); String dlt = dltcode.nextLine(); double dltprice=dltorderprocess(foodArray,dlt); if (dltprice == -1) System.out.println("Invalid Order Code"); else System.out.println("You have delete an item"); total-=dltprice; break; case 0: System.out.println("Bye"); loopagain=false; break; default: System.out.println("Invalid choice"); } } } public static void displayJuice(Food[] foodArray){ for (int i = 0; i < foodArray.length; ++i) { if (foodArray[i] instanceof Juice){ System.out.println("\nJuice\n============"); System.out.println(foodArray[i]); } } } public static void displaySnack(Food[] foodArray){ for (int i = 0; i < foodArray.length; ++i) { if (foodArray[i] instanceof Snack){ System.out.println("\nSnack\n============"); System.out.println(foodArray[i]); } } } public static double orderprocess(Food[] foodArray,String code){ for (int i = 0; i < foodArray.length; ++i) { if(foodArray[i].getcode().compareTo(code)==0){ return foodArray[i].getprice(); } }return -1; } public static double addorderprocess(Food[] foodArray,String add){ for (int a = 0; a < foodArray.length; ++a) { if(foodArray[a].getcode().compareTo(add)==0){ return foodArray[a].getprice(); } }return -1; } public static double dltorderprocess(Food[] foodArray,String dlt){ for (int b = 0; b < foodArray.length; ++b) { if(foodArray[b].getcode().compareTo(dlt)==0){ return foodArray[b].getprice(); } }return -1; } } class Snack extends Food{ private String packing; private String size; public Snack(){ } public Snack(String name, String code, double price,String description ,String packing,String size ){ super(name, code,price,description); this.packing= packing; this.size= size; } public String toString(){ return super.toString() + "\nPacking: " + packing + "\nSize: " + size; } } class Juice extends Food{ private String flavor; private int cup ; public Juice(){ } public Juice(String name, String code, double price,String description, String flavor,int cup){ super(name,code,price,description); this.flavor = flavor; this.cup = cup; } public String toString(){ return super.toString() + "\nFlavor: " + flavor+ "\nCup: " + cup; } } abstract class Food{ private String name; private String code; private double price; private String description; public Food(){ } public Food(String name ,String code,double price,String description){ this.name = name ; this.code = code ; this.price = price ; this.description =description ; } public void setname(String name){ this.name = name; } public void setcode(String code){ this.code = code; } public void setprice(Double price){ this.price = price; } public void setdescription(String description){ this.description = description; } public String getname(){ return name; } public String getcode(){ return code; } public Double getprice(){ return price; } public String getdescription(){ return description; } public String toString(){ return "Name: " + name + "\nCode: " + code + "\nPrice: " + price + "\nDescription: " + description; } }
Please someone help me , based on the java code given , how can i add a simple payment system in the program .
import java.util.Scanner; public static void main(String [] args) Food[] foodArray = new Food[4]; foodArray[0] = new Juice("Apple","C01",5.00, "Which is very popular juice in our shop.","Apple",1);
boolean loopagain = true; while(loopagain){ System.out.println("1.\t Display Food"); double total=0; Scanner in = new Scanner(System.in); int choice=in.nextInt(); switch (choice) { Scanner on = new Scanner(System.in); switch(food_choice){ case 2:Scanner ordercode = new Scanner(System.in);
break; double addprice=addorderprocess(foodArray,add); break; double dltprice=dltorderprocess(foodArray,dlt); case 0: System.out.println("Bye"); } public static void displayJuice(Food[] foodArray){ if (foodArray[i] instanceof Juice){ System.out.println("\nJuice\n============"); public static void displaySnack(Food[] foodArray){ for (int i = 0; i < foodArray.length; ++i) { if (foodArray[i] instanceof Snack){ System.out.println("\nSnack\n============"); public static double orderprocess(Food[] foodArray,String code){ public static double addorderprocess(Food[] foodArray,String add){ public static double dltorderprocess(Food[] foodArray,String dlt){
class Snack extends Food{ public Snack(){ public Snack(String name, String code, double price,String description ,String packing,String size ){ public String toString(){
class Juice extends Food{ public Juice(){ public Juice(String name, String code, double price,String description, String flavor,int cup){
abstract class Food{ public Food(){ public Food(String name ,String code,double price,String description){ this.name = name ;
public void setcode(String code){ public void setprice(Double price){ public void setdescription(String description){ public String getname(){ public String getcode(){ public Double getprice(){ public String getdescription(){ public String toString(){
|

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

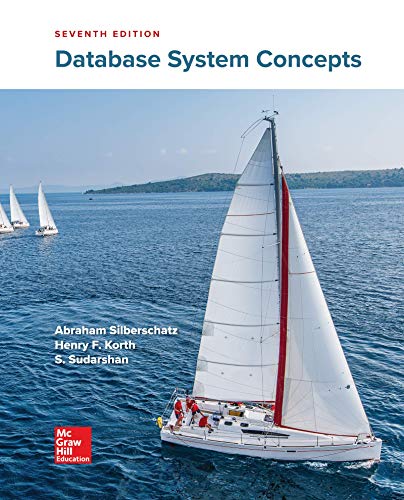
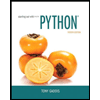
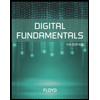
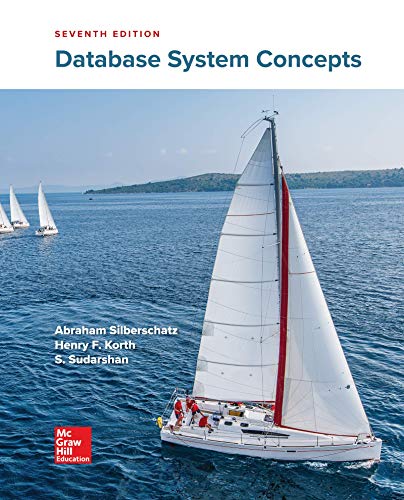
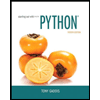
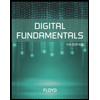
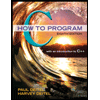
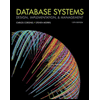
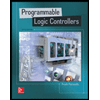