Imagine a Car Parts and Accessories shop, which requires a software system to keep track of stock items and prices. The shop will sell different kinds of stock items. However, to start with, you have been tasked with designing and implementing a class called StockItem with the following properties. An instance (object) of the StockItem class represents a particular item which the shop sells, with a string representing fixed stock code, an integer representing variable quantity in stock and a double representing variable price of the stocked item. All these variables should be declared as private variables. The StockItemclass also contains a class variable (shared by all instances) of type string representing stock category which you can initialise as 'Car accessories'. A constructor that creates a Stock Item with the specified quantity, price and the stock code. All the appropriate ‘setters’ and ‘getters’ methods, including a getStockName() method which returns the string "Unknown Stock Name" and a getStockDescription() method which returns the string "Unknown Stock Description". An increaseStock() method that increases the stock level by the given amount. If the value is less than 1 or the stock exceeds 100, a suitable error message should be printed. A sellStock() method that attempts to reduce the stock level by the given amount. If it is less than 1, a suitable error message should be printed. If the amount is otherwise less than or equal to the stock level, then the reduction is successful and true is returned. Else there is no effect, but false is returned. A getVAT() method that returns the standard percentage VAT rate, e.g., you can use 17.5 Appropriate ‘setters’ method for price (without VAT) and ‘getters’ methods for price with and without VAT A method named __str__ () that returns a string giving the stock code, the stock name, the description, the quantity in stock, the price before VAT and the price after VAT. It must use the appropriate methods above to obtain the stock name, description, quantity and prices
Imagine a Car Parts and Accessories shop, which requires a software system to keep track of stock items and prices. The shop will sell different kinds of stock items. However, to start with, you have been tasked with designing and implementing a class called StockItem with the following properties.
-
An instance (object) of the StockItem class represents a particular item which the shop sells, with a string representing fixed stock code, an integer representing variable quantity in stock and a double representing variable price of the stocked item. All these variables should be declared as private variables. The StockItemclass also contains a class variable (shared by all instances) of type string representing stock category which you can initialise as 'Car accessories'.
-
A constructor that creates a Stock Item with the specified quantity, price and the stock code.
-
All the appropriate ‘setters’ and ‘getters’ methods, including a getStockName() method which returns the string "Unknown Stock Name" and a getStockDescription() method which returns the string "Unknown Stock Description".
-
An increaseStock() method that increases the stock level by the given amount. If the value is less than 1 or the stock exceeds 100, a suitable error message should be printed.
-
A sellStock() method that attempts to reduce the stock level by the given amount. If it is less than 1, a suitable error message should be printed. If the amount is otherwise less than or equal to the stock level, then the reduction is successful and true is returned. Else there is no effect, but false is returned.
-
A getVAT() method that returns the standard percentage VAT rate, e.g., you can use 17.5
-
Appropriate ‘setters’ method for price (without VAT) and ‘getters’ methods for price with and without VAT
-
A method named __str__ () that returns a string giving the stock code, the stock name, the description, the quantity in stock, the price before VAT and the price after VAT. It must use the appropriate methods above to obtain the stock name, description, quantity and prices.

Step by step
Solved in 2 steps with 3 images

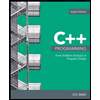
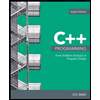