Implement a superclass Appointment and subclasses Onetime, Daily, and Monthly. An appointment has a description (for example, “see the dentist”) and a date (use int's to store the date). Write a method occursOn(int year, int month, int day) that checks whether the appointment occurs on that date. For example, for a monthly appointment, you must check whether the day of the month matches. Additionally, you will need to implement a class called Calendar. This will contain an ArrayList of Appointment objects (The list is an instance variable of the Calendar class). You will need to provide the following methods for your Calendar class. A no argument constructor public Calendar(){...} This constructor will initialize your ArrayList instance variable to an empty list. /** * A method to add an appointment to the calendar * @param apt – the appointment object to add to the calendar. */ public void add(Appointment apt) {…} /** * A method to remove an appointment from the calendar. * This method uses the occursOn() method from the public * interface for the Appointment class. Therefore, if parameters * are entered that occur after a start date for a given Daily * appointment * the Daily appointment will be removed as well. (Because occursOn() * willreturn true in this case). This is a limitation we will * accept for now. * @param year - the year of the appointment to remove * @param month - the month of the appointment to remove * @param day - the day of the appointment to remove */ public void remove(int year, int month, int day) { //this method needs to iterate over your list of appointments //and remove elements who's occursOn() method return true //when passed the parameters above. } /** * Method to return a string representation of this Calendar object. * Overrides the Object method toString * @return a String representation of the Calendar object. */ public String toString() { String ret = ""; //this method needs to iterate over your list of appointments //and construct the return string //make sure to put each appointment on its own line //by using “\n” return ret; } My question is: I am unable to add any items into the ArrayList. The error I'm recieving says that the type is not applicable for the arguements. Arrays are very confusing to me, so I'm sure there is something I am missing, but I've tried changing the type of the input to string before adding it to the list and nothing seems to be working. What am I doing wrong?
Implement a superclass Appointment and subclasses Onetime, Daily, and Monthly. An appointment has a description (for example, “see the dentist”) and a date (use int's to store the date). Write a method occursOn(int year, int month, int day) that checks whether the appointment occurs on that date. For example, for a monthly appointment, you must check whether the day of the month matches.
Additionally, you will need to implement a class called Calendar. This will contain an ArrayList of Appointment objects (The list is an instance variable of the Calendar class). You will need to provide the following methods for your Calendar class.
A no argument constructor
public Calendar(){...}
This constructor will initialize your ArrayList instance variable to an empty list.
/**
* A method to add an appointment to the calendar
* @param apt – the appointment object to add to the calendar.
*/
public void add(Appointment apt) {…}
/**
* A method to remove an appointment from the calendar.
* This method uses the occursOn() method from the public
* interface for the Appointment class. Therefore, if parameters
* are entered that occur after a start date for a given Daily
* appointment
* the Daily appointment will be removed as well. (Because occursOn() * willreturn true in this case). This is a limitation we will
* accept for now.
* @param year - the year of the appointment to remove
* @param month - the month of the appointment to remove
* @param day - the day of the appointment to remove
*/
public void remove(int year, int month, int day) {
//this method needs to iterate over your list of appointments
//and remove elements who's occursOn() method return true
//when passed the parameters above.
}
/**
* Method to return a string representation of this Calendar object.
* Overrides the Object method toString
* @return a String representation of the Calendar object.
*/
public String toString() {
String ret = "";
//this method needs to iterate over your list of appointments
//and construct the return string
//make sure to put each appointment on its own line
//by using “\n”
return ret;
}
My question is: I am unable to add any items into the ArrayList. The error I'm recieving says that the type is not applicable for the arguements. Arrays are very confusing to me, so I'm sure there is something I am missing, but I've tried changing the type of the input to string before adding it to the list and nothing seems to be working. What am I doing wrong?
This is an adaptation off of Big Java 6th edition Practice probem P9.3



Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

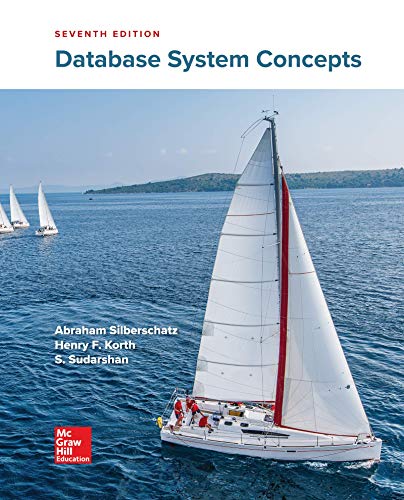
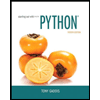
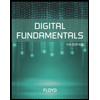
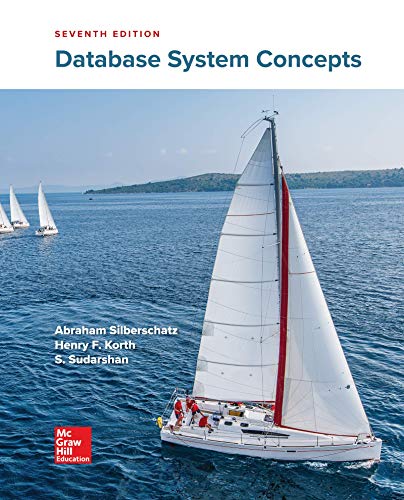
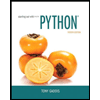
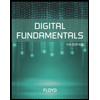
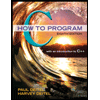
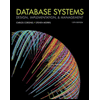
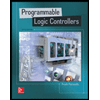