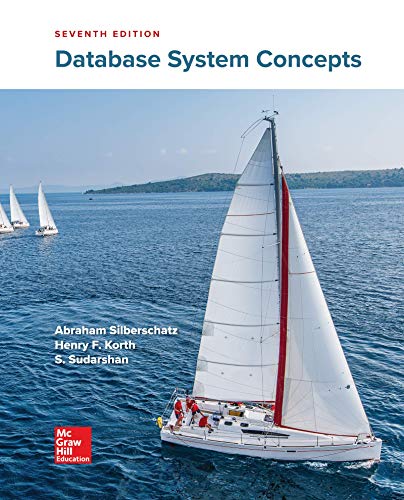
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
Write a Java program with at least two classes. Use a circle object to represent it. The class must offer at least one (non-static) setter method and one (non-static) getter method. Implement at least one test that invokes the setter method and the corresponding getter method so that the value out == the value in. Also use assert.Equal(60, circle1.getCircumference(), 10); and assert.Equals(10, circle1.get Radius(), 0.1); for radius
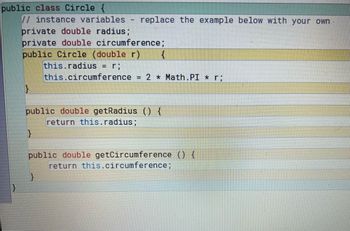
Transcribed Image Text:public class Circle {
// instance variables
}
private double radius;
private double circumference;
public Circle (double r) {
this.radius = r;
L
}
replace the example below with your own.
public double getRadius () {
return this.radius;
}
this.circumference = 2 * Math.PI * r;
public double getCircumference () {
return this.circumference;
Expert Solution

arrow_forward
Step 1
In the above program, setters basically take the input from the program. Whereas through the getter we can show take the output or display.
In the program get methods are implemented already. We have to implement the setters' methods based on the condition and call them. Below the complete code is given -
Step by stepSolved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Please write a Java program with at least two classes. Use a circle to represent it! The class should have at least one non-static setter method and one non-static getter. Implement at least one method test that invokes the setter method and the corresponding getter method so that the value out == the value in!arrow_forwardWrite a java program to complete the following parts: Part-1: Create a class Car and perform the following: - Declare public instance variables (name, model, color) as (String, int, and String) respectively. - Define set() method to set a car's name, model, and color. Define show() method to print out the car's name, model, and color. Part-2: In the main method: Declare an array of the defined class Car with length 5. Create three objects from Car and add them into the array. Set the objects' values (name, model, color) using set method. Print out the object's values (name, model, color) using show method.arrow_forwardWrite a java class method named capitalizeWords that takes one parameter of type String. The method should print its parameter, but with the first letter of each word capitalized. For example, the call capitalizeWords ("abstract window toolkit"); will produce the following output: Abstract Window Toolkitarrow_forward
- 1) Implement a class Moth that models a moth flying along a straight line. The moth hasa position, which is the distance from a fixed origin. When the moth moves toward apoint of light, its new position is halfway between its old position and the position ofthe light source. Find the instance variable(s), define and implement the constructor(s), then supplythe following methods:- public void moveToLight(double lightPosition) - public double getPosition()2) Document your class, generate Javadoc documentation and produce a test program ‘MothTester’that construct a moth, move it toward a couple of light sources, and check that the moth’s positionis as expected. 3) Add a comment to your class Moth that explains how you can make the Moth immobile after 10moves.arrow_forwardA java program, with a class Rectangle with attributes length and width , each of which defaults to 2. Providemethods that calculate the rectangle’s perimeter and area. It has set and get methods for bothlength and width . The set methods should verify that length and width are each floating-pointnumbers larger than 10.0 and less than 40.0. Write a program to test class Rectangle .arrow_forwardin java Given main(), define the Team class (in file Team.java). For class method getWinPercentage(), the formula is:wins / (wins + losses). Note: Use casting to prevent integer division. For class method printStanding(), output the win percentage of the team with two digits after the decimal point and whether the team has a winning or losing average. A team has a winning average if the win percentage is 0.5 or greater. Ex: If the input is: Ravens 13 3 where Ravens is the team's name, 13 is the number of team wins, and 3 is the number of team losses, the output is: Win percentage: 0.81 Congratulations, Team Ravens has a winning average! Ex: If the input is: Angels 80 82 the output is: Win percentage: 0.49 Team Angels has a losing average.arrow_forward
- Java allows for methods to be chained together. Consider the following message from the captain of a pirate ship: String msg1 = " Maroon the First Mate with a flagon of water and a pistol! "; We want to change the message to read the message msg1: String msg2= “Maroon the Quartermaster with a flagon of water.” Three changes need to be made to adjust the string as desired: Trim the leading and trailing whitespace. Replace the substring First Mate with Quartermaster. Remove "and a pistol!" Add a period at the end of the sentence. A “chaining1” method which will apply in sequence 4 operations to perform the above. We will use the trim, replace, and substring methods, in this order. Thus the chaining1 method will receive a string msg1 and return a string msg2. Make sure msg2 is printed. A “chaining2” method which will apply the 4 operations above in one single statement. Thus the chaining2 method will receive a string msg1 and return as string msg2. Make sure msg2 is printedarrow_forwardWrite a Java class called Rectangle that represents a rectangular, two-dimensional region. In thislab you learn overriding a system method. Create a default, empty constructor.Set the double values for the ‘x’ ‘y’ ‘height’ ‘width’ in the Rectangle objects whose top-leftcorner is specified by the given ‘x’ and ‘y’ coordinates and by the width and height. Your classwill need to use setters and getters with these 4 values. Recall we discussed in class lectures toput values into the instantiated object.Override the ‘toString()’ base method to produce the following information inside the singlequotes. EXAMPLE OF OUTPUT=‘Rectangle [x=2.5, y=13.0, height=14.0, width=4.0] Area is 54.0’Write a test program called TestRectangle that creates objects of the Rectangle class called rect1and rect2. Assign values to the fields of these objects. Print out these Rectangle objects usingSystem.out.println() from inside the main() method (Hint: this will utilize the overridden‘toString()’ in the Rectangle…arrow_forwardModify class Time2 (pasted below) to include a tick method that increments the time stored in a Time2 object by one second. Provide method incrementMinute to increment the minute by one and method incrementHour to increment the hour by one. Write a program that tests the tick method, the incrementMinute method and the incrementHour method to ensure that they work correctly. Be sure to test the following cases: a. incrementing into the next minute, b. incrementing into the next hour and c. incrementing into the next day (i.e., 11:59:59 PM to 12:00:00 AM). public class Time2 {private int hour; // 0 - 23private int minute; // 0 - 59private int second; // 0 - 59 //no-argument constructor that initializes each instance variable to zeropublic Time2() {this(0, 0, 0); //invoke constructor with three arguments}//constructor, hour supplied, min and sec defaulted to 0public Time2(int hour) {this(hour, 0, 0); //invoke constructor}//hour and min suppliedpublic Time2(int hour, int minute)…arrow_forward
- In Java, please. Complete the Course class by implementing the findStudentHighestGpa() method, which returns the Student object with the highest GPA in the course. Assume that no two students have the same highest GPA. Given classes: Class LabProgram contains the main method for testing the program. Class Course represents a course, which contains an ArrayList of Student objects as a course roster. (Type your code in here.) Class Student represents a classroom student, which has three fields: first name, last name, and GPA. (Hint: getGPA() returns a student's GPA.) Note: For testing purposes, different student values will be used. Ex. For the following students: Henry Nguyen 3.5 Brenda Stern 2.0 Lynda Robison 3.2 Sonya King 3.9 the output is: Top student: Sonya King (GPA: 3.9) LabProgram.java import java.util.Scanner; public class LabProgram { public static void main(String args[]) { Scanner scnr = new Scanner(System.in); Course course = new Course(); int…arrow_forwardUse Java program to add a method printReceipt to the CashRegister class. The method should print the prices of all purchased items and the total amount due. Hint: You will need to form a string of all prices. Use the concat method of the String class to add additional items to that string. To turn a price into a string, use the call String.valueOf(price). Also, the payment information will need to be the same as the price information. Here is the CashRegister class: /** A cash register totals up sales and computes change due.*/public class CashRegister{ private double purchase; private double payment; /** Constructs a cash register with no money in it. */ public CashRegister() { purchase = 0; payment = 0; } /** Records the sale of an item. @param amount the price of the item */ public void recordPurchase(double amount) { purchase = purchase + amount; } /** Processes a payment received from the customer. @param…arrow_forwardCan you do this please? Thank youarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
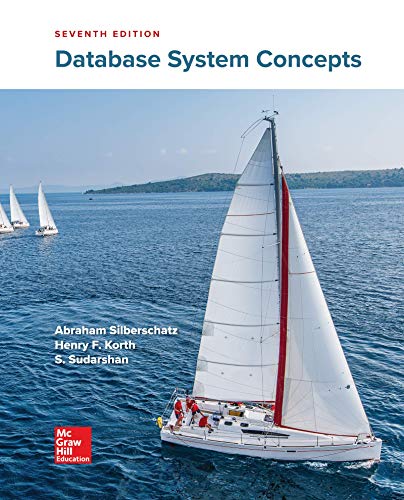
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
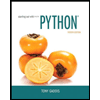
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
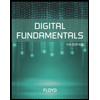
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
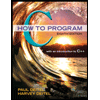
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
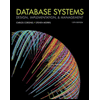
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
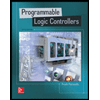
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education