Implement the Solver class. The point of the solver class is the solve method which takes a board/puzzle configuration represented as a 2D array of bytes and returns a byte array containing a minimal sequence of moves that will lead to the solved board. The 2D array of bytes is “triangular” and represents a valid board configuration. Namely, the 2D array has 5 rows (0 – 4) and the size of every row is 1 more than its index.The array contains one 0, five 1s, four 2s and five 3s. The solve method then returns an array of bytes representing a minimal sequence of moves that solves the puzzle. In other words, if the numbers from the returned array are used in order as inputs to the move method on the Board object representing the initial configuration, the resulting board configuration represents the solved board. If the input to the solve method is a board configuration that is already solved, then solution requires no moves and an array of size 0 must be returned. impliment the solver class using the provided code. import java.util.ArrayList; import java.util.Arrays; public class Solver { public Solver() { // TODO } public byte[] solve(byte[][] start) { // Flatten the 2D array to simplify manipulation byte[] flatStart = flatten(start); // If the board is already solved, return an empty array if (isSolved(flatStart)) { return new byte[0]; } // Find the index of 0 in the flattened array int zeroIndex = indexOfZero(flatStart); // Initialize an ArrayList to store the moves ArrayList moves = new ArrayList<>(); // Apply moves to solve the puzzle while (!isSolved(flatStart)) { // Move the 0 to the next row int nextRowStart = (zeroIndex / (flatStart.length / 5) + 1) * (flatStart.length / 5); swap(flatStart, zeroIndex, nextRowStart); moves.add((byte) (nextRowStart - zeroIndex)); // Move the 0 to the correct column int targetColumn = (zeroIndex % (flatStart.length / 5)) + 1; int targetIndex = nextRowStart + targetColumn; swap(flatStart, zeroIndex, targetIndex); moves.add((byte) (targetIndex - zeroIndex)); } // Convert ArrayList to array byte[] result = new byte[moves.size()]; for (int i = 0; i < result.length; i++) { result[i] = moves.get(i); } return result; } // Helper method to flatten the 2D array private byte[] flatten(byte[][] array2D) { return Arrays.stream(array2D) .flatMapToInt(Arrays::stream) .mapToByte(b -> b) .toArray(); } // Helper method to check if the board is already solved private boolean isSolved(byte[] board) { return Arrays.equals(board, getSolution(board.length)); } // Helper method to get the solution for a given board size private byte[] getSolution(int size) { byte[] solution = new byte[size]; int currentNumber = 1; for (int i = 0; i < 5; i++) { for (int j = 0; j <= i; j++) { solution[i * (i + 1) / 2 + j] = (byte) currentNumber; currentNumber++; } } return solution; } // Helper method to find the index of 0 in the array private int indexOfZero(byte[] array) { for (int i = 0; i < array.length; i++) { if (array[i] == 0) { return i; } } throw new IllegalArgumentException("Array does not contain 0"); } // Helper method to swap two elements in the array private void swap(byte[] array, int i, int j) { byte temp = array[i]; array[i] = array[j]; array[j] = temp; } }
Implement the Solver class. The point of the solver class is the solve method which takes a board/puzzle configuration represented as a 2D array of bytes and returns a byte array containing a minimal sequence of moves that will lead to the solved board. The 2D array of bytes is “triangular” and represents a valid board configuration. Namely, the 2D array has 5 rows (0 – 4) and the size of every row is 1 more than its index.The array contains one 0, five 1s, four 2s and five 3s. The solve method then returns an array of bytes representing a minimal sequence of moves that solves the puzzle. In other words, if the numbers from the returned array are used in order as inputs to the move method on the Board object representing the initial configuration, the resulting board configuration represents the solved board. If the input to the solve method is a board configuration that is already solved, then solution requires no moves and an array of size 0 must be returned.
impliment the solver class using the provided code.
import java.util.ArrayList;
import java.util.Arrays;
public class Solver {
public Solver() {
// TODO
}
public byte[] solve(byte[][] start) {
// Flatten the 2D array to simplify manipulation
byte[] flatStart = flatten(start);
// If the board is already solved, return an empty array
if (isSolved(flatStart)) {
return new byte[0];
}
// Find the index of 0 in the flattened array
int zeroIndex = indexOfZero(flatStart);
// Initialize an ArrayList to store the moves
ArrayList<Byte> moves = new ArrayList<>();
// Apply moves to solve the puzzle
while (!isSolved(flatStart)) {
// Move the 0 to the next row
int nextRowStart = (zeroIndex / (flatStart.length / 5) + 1) * (flatStart.length / 5);
swap(flatStart, zeroIndex, nextRowStart);
moves.add((byte) (nextRowStart - zeroIndex));
// Move the 0 to the correct column
int targetColumn = (zeroIndex % (flatStart.length / 5)) + 1;
int targetIndex = nextRowStart + targetColumn;
swap(flatStart, zeroIndex, targetIndex);
moves.add((byte) (targetIndex - zeroIndex));
}
// Convert ArrayList to array
byte[] result = new byte[moves.size()];
for (int i = 0; i < result.length; i++) {
result[i] = moves.get(i);
}
return result;
}
// Helper method to flatten the 2D array
private byte[] flatten(byte[][] array2D) {
return Arrays.stream(array2D)
.flatMapToInt(Arrays::stream)
.mapToByte(b -> b)
.toArray();
}
// Helper method to check if the board is already solved
private boolean isSolved(byte[] board) {
return Arrays.equals(board, getSolution(board.length));
}
// Helper method to get the solution for a given board size
private byte[] getSolution(int size) {
byte[] solution = new byte[size];
int currentNumber = 1;
for (int i = 0; i < 5; i++) {
for (int j = 0; j <= i; j++) {
solution[i * (i + 1) / 2 + j] = (byte) currentNumber;
currentNumber++;
}
}
return solution;
}
// Helper method to find the index of 0 in the array
private int indexOfZero(byte[] array) {
for (int i = 0; i < array.length; i++) {
if (array[i] == 0) {
return i;
}
}
throw new IllegalArgumentException("Array does not contain 0");
}
// Helper method to swap two elements in the array
private void swap(byte[] array, int i, int j) {
byte temp = array[i];
array[i] = array[j];
array[j] = temp;
}
}

Step by step
Solved in 4 steps with 3 images

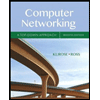
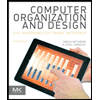
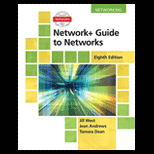
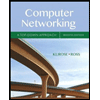
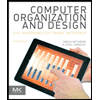
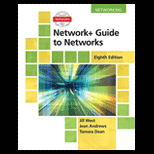
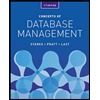
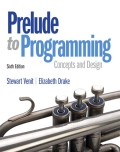
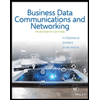