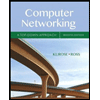
Concept explainers
Write a program that generates a random number in the range of 1 through 1000, and asks
the user to guess what the number is. If the user guess is higher than the random number,
the program should display Too high, try again. If the users guess is lower than the
random number, the program should display Too low, try again. If the user guesses the
number, the application should congratulate the user and generate a new random number
so the game can start over. If the user doesn't want to continue the game the user should type an appropriate stop symbol to terminate the program (such stop conditions were discussed in the class). For each game iteration, count the number of guesses made by the user and display them. The program should contain the following functions:
check_guess(): this function checks if the user's guess is lower, higher or equal to the random number.
random_gen(): function that generates one random number for one game iteration
main(): the function where the program execution should begin and end.
The number of function arguments and parameters are left for your own design choice.

Step by stepSolved in 4 steps with 2 images

- C CODE: create a program that will test the user’s proficiency in solving different types of math problems. The program will be menu-driven. The user will select either addition, subtraction, multiplication, or division problems. The program will then display a problem, prompt the user for an answer, and then check the answer displaying an appropriate message to the user whether their answer was correct or incorrect. The user will be allowed 3 tries at each problem. The program will also keep statistics on how many problems were answered correctly. add functions to allow the user to select problems of varying degrees of difficulty In the next Week, you'll need to add functionality to also keep statistics on the number of correct answers vs. the total number of problems attempted. So now let’s add the code for the remaining functions: We’ll start by inserting code to ask the user if they want to see problems that are easy, medium, or difficult. We’ll do this by prompting them to…arrow_forwardImplement the EvenOdd application that asks a user to enter an integer. Display a statement that indicates whether the integer is even or odd. An example of the program is shown below: Enter a number >> 372 372 is even The EvenOdd program properly classifies numbers as even or odd. 4 Click the checkbox above to attempt this task. Checks Test CaseIncomplete Odd number test with 23 Test CaseIncomplete Odd number test with 451 Test CaseIncomplete Even number test with 12 Test CaseIncomplete Even number test with 0 import java.util.Scanner; class EvenOdd { public static void main(String[] args) { // Write your code here } public static boolean isEven(int number) { } }arrow_forwardTrue or False: Adding more control variables will always increase the R 2 value. True Falsearrow_forward
- Design and implement an application that presents three buttons and a label to the user. The buttons should be labeled Increment, Decrement, and Randomize. Display a numeric value (initially 50) using the label. Each time the Increment button is pushed, increment the value displayed. Likewise, each time the Decrement button is pushed, decrement the value displayed. When the Randomize button is pushed, the number displayed should be randomized between 1 and 100, inclusive.arrow_forwardHello Sir.Good Evening. I have a question in my home work related computer programming lesson. The following below is my question (12.15). Please advice. Thank youarrow_forwardWrite method IsEven that uses the remainder operator (%) to determine whether an integer is even. The method should take an integer argument and return true if the integer is even and false otherwise. Incorporate this method into an app that inputs a sequence of integers (one at a time) and determines whether each is even or odd.arrow_forward
- The game of Nim starts with a random number of stones between 15 and 30. Two players alternate turns and on each turn may take either 1, 2, or 3 stones from the pile. The player forced to take the last stone loses. Create a nim application in python that allows the user to play against the computer. In this version of the game, the application generates the number of stones to begin with, the number of stones the computer takes, and the user goes first. The Nim application code should: prevent the user and the computer from taking an illegal number of stones. For example, neither should be allowed to take three stones when there are only 1 or 2 left. include an is_valid_entry() function to check user input. include a draw_stones() function that generates a random number from 1 to 3 for the number of stones the computer draws. include separate functions to handle the user’s turn and the computer’s turn. My problem is that the code is not outputting like it's supposed to.…arrow_forwardWrite the pseudocode to create a lotto game. The user will select three numbers from 1 to 9. If the user enters a number outside of this range, then they must beprompted to re-enter the number until it meets the criteria. The numbers must be unique and must not be duplicated. Generate three random numbers from 1 to 9 in value and compare the three random numbers against the user's selection of numbers. If they are equal to the random number, then that is match.arrow_forwardIn python Expense tracker: the user should be able to enter expenses per category (food, clothing, entertainment, rent, etc) and the application should track the expenses per week or month. The user should be able to see their total expenses for a month of their choice for each category and a total monthly expense. The monthly expense report should include average expenses for each category for the year and indicate if the user expense for the month selected is lower or higher than the annual average. Also, the report should display the percentage of expenses from each category out of the total monthly expenses. Project application requirements: Requirements description Basic Points: * Project should have a functioning menu * Project should have adequate functions (minimum 3 functions) * Project should make use of files to save the data * Project should handle adequate exceptions * Project should display information formatted adequately * Project should make use of lists or…arrow_forward
- Write a JAVA program that can be used to assign seats for a commercial airplane. The airplane has 13 rows, with 6 seats in each row. Rows 1 and 2 are first class, rows 3 to 7 are business class, and rows 8 to 13 are economy class. Your program prompts the user to enter the following information: Ticket type (first class, business class, or economy class) Desired seat ‘*’ indicates that the seat is available; ‘X’ indicates that the seat has been assigned. Make this a menu-driven program; show the user’s choices and allow the user to make the appropriate choices.arrow_forwardAssume that a gallon of paint covers about 350 square feet of wall space. Create an application with a main() method that prompts the user for the length, width, and height of a rectangular room. Pass these three values to a method that does the following: Calculates the wall area for a room Passes the calculated wall area to another method that calculates and returns the number of gallons of paint needed Displays the number of gallons needed Computes the price based on a paint price of $32 per gallon, assuming that the painter can buy any fraction of a gallon of paint at the same price as a whole gallon Returns the price to the main() method The main() method displays the final price. For example: You will need 2.0 gallons The price to paint the room is $64.0 import java.util.Scanner; public class PaintCalculator { public static void main (String args[]) { // Write your code here } public static double computeArea(double length, double width, double height)…arrow_forwardMark Daniels is a carpenter who creates personalized house signs. He wants an application to compute the price of any sign a customer orders, based on the following factors: The minimum charge for all signs is $30. If the sign is made of oak, add $15. No charge is added for pine. The first six letters or numbers are included in the minimum charge; there is a $3 charge for each additional character. Black or white characters are included in the minimum charge; there is an additional $12 charge for gold-leaf lettering. Design a flowchart or pseudocode for the following: a. A program that accepts data for an order number, customer name, wood type, number of characters, and color of characters. Display all the entered data and the final price for the sign. b. A program that continuously accepts sign order data and displays all the relevant information for oak signs with five white letters. c. A program that continuously accepts sign order data and displays all the relevant information for…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
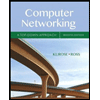
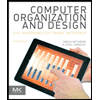
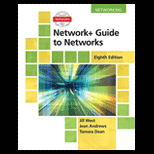
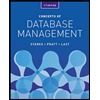
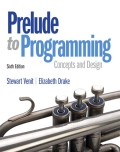
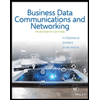