Implement two out of three of the basic sorting algorithms - Bubble Sort, Selection Sort, and Insertion Sort - in a generic List.
Implement two out of three of the basic sorting
Initial code to be completed:
#include <iostream>
#include "linkedlist.h"
void bubbleSort(List*);
void selectionSort(List*);
void insertionSort(List*);
/**
* This activity is focused on using Arrays and Linked Lists as two different
* implementations of List. It follows that you are only to use the methods of
* List and not of the specific array or linkedlist.
*/
int main(void) {
// WARNING! Do not modify main method!
// Doing so will nullify your score for this activity.
char li;
cin >> li;
List* list;
if (li == 'A') {
list = new ArrayList();
} else {
list = new LinkedList();
}
int length;
cin >> length;
int input;
for (int i = 0; i < length; i++) {
cin >> input;
list->add(input);
}
char sym;
cin >> sym;
int arg1;
switch (sym) {
case 'B':
bubbleSort(list);
break;
case 'I':
insertionSort(list);
break;
case 'S':
selectionSort(list);
break;
}
return 0;
};
//Perform two of three sorting algorithms here.
//Reminder: Do not use methods specific to Array or Linked List.
void bubbleSort(List* list) {
};
void selectionSort(List* list) {
};
void insertionSort(List* list) {
};

Step by step
Solved in 2 steps

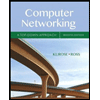
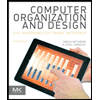
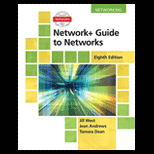
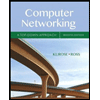
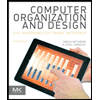
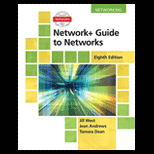
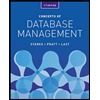
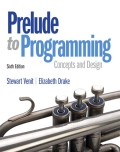
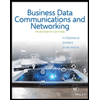