import java.util.*; public class CardGame { static Scanner scanner = new Scanner(System.in); static Random random = new Random(); public static void main(String[] args) { System.out.println("Welcome to the card game! Here are the instructions:"); System.out.println("- You will randomly draw a card from a deck of 52 cards."); System.out.println("- The computer will also draw a card from the same deck."); System.out.println("- If your card has a higher face value, you win. Otherwise, you lose."); System.out.println("- If your card has the same face value as the computer's card, the suit determines the order."); int gamesPlayed = 0; int userWins = 0; int computerWins = 0; boolean[] cards = new boolean[52]; // false means the card is available, true means it's been drawn while (true) { System.out.println("\nNew game!"); int[] userCard = drawCard(cards); int[] computerCard = drawCard(cards); System.out.println("Your card is " + cardToString(userCard)); System.out.println("Computer's card is " + cardToString(computerCard)); int userValue = userCard[1]; int computerValue = computerCard[1]; if (userValue > computerValue) { System.out.println("You win!"); userWins++; } else if (userValue < computerValue) { System.out.println("You lose!"); computerWins++; } else { // same face value, compare suits int userSuit = userCard[0]; int computerSuit = computerCard[0]; if (userSuit > computerSuit) { System.out.println("You win!"); userWins++; } else { System.out.println("You lose!"); computerWins++; } } gamesPlayed++; if (gamesPlayed == 26) { System.out.println("\nYou have played 26 games, which is half of the deck."); System.out.println("Do you want to shuffle the deck and start a new game? (y/n)"); String answer = scanner.next(); if (answer.equals("y")) { Arrays.fill(cards, false); gamesPlayed = 0; userWins = 0; computerWins = 0; } else { break; } } else { System.out.println("\nDo you want to play another game? (y/n)"); String answer = scanner.next(); if (!answer.equals("y")) { break; } } } System.out.println("\nThanks for playing!"); System.out.println("You played " + gamesPlayed + " games."); System.out.println("You won " + userWins + " times."); System.out.println("The computer won " + computerWins + " times."); } public static int[] drawCard(boolean[] cards) { while (true) { int suit = random.nextInt(4) + 1; int value = random.nextInt(13) + 2; int index = (suit - 1) * 13 + (value - 2); if (!cards[index]) { cards[index] = true; return new int[] { suit, value }; } } } public static String cardToString(int[] card) { String suitStr = ""; switch (card[0]) { case 1: suitStr = "club"; break; case 2: suitStr = "diamond"; break; case 3: suitStr = "heart"; break; case 4: suitStr = "spade"; break; } String valueStr; switch (card[1]) { case 11: valueStr = "Jack"; break; case 12: valueStr = "Queen"; break; case 13: valueStr = "King"; break; case 14: valueStr = "Ace"; break; default: valueStr = Integer.toString(card[1]); break; } return valueStr + " of " + suitStr + "s"; } }
import java.util.*; public class CardGame { static Scanner scanner = new Scanner(System.in); static Random random = new Random(); public static void main(String[] args) { System.out.println("Welcome to the card game! Here are the instructions:"); System.out.println("- You will randomly draw a card from a deck of 52 cards."); System.out.println("- The computer will also draw a card from the same deck."); System.out.println("- If your card has a higher face value, you win. Otherwise, you lose."); System.out.println("- If your card has the same face value as the computer's card, the suit determines the order."); int gamesPlayed = 0; int userWins = 0; int computerWins = 0; boolean[] cards = new boolean[52]; // false means the card is available, true means it's been drawn while (true) { System.out.println("\nNew game!"); int[] userCard = drawCard(cards); int[] computerCard = drawCard(cards); System.out.println("Your card is " + cardToString(userCard)); System.out.println("Computer's card is " + cardToString(computerCard)); int userValue = userCard[1]; int computerValue = computerCard[1]; if (userValue > computerValue) { System.out.println("You win!"); userWins++; } else if (userValue < computerValue) { System.out.println("You lose!"); computerWins++; } else { // same face value, compare suits int userSuit = userCard[0]; int computerSuit = computerCard[0]; if (userSuit > computerSuit) { System.out.println("You win!"); userWins++; } else { System.out.println("You lose!"); computerWins++; } } gamesPlayed++; if (gamesPlayed == 26) { System.out.println("\nYou have played 26 games, which is half of the deck."); System.out.println("Do you want to shuffle the deck and start a new game? (y/n)"); String answer = scanner.next(); if (answer.equals("y")) { Arrays.fill(cards, false); gamesPlayed = 0; userWins = 0; computerWins = 0; } else { break; } } else { System.out.println("\nDo you want to play another game? (y/n)"); String answer = scanner.next(); if (!answer.equals("y")) { break; } } } System.out.println("\nThanks for playing!"); System.out.println("You played " + gamesPlayed + " games."); System.out.println("You won " + userWins + " times."); System.out.println("The computer won " + computerWins + " times."); } public static int[] drawCard(boolean[] cards) { while (true) { int suit = random.nextInt(4) + 1; int value = random.nextInt(13) + 2; int index = (suit - 1) * 13 + (value - 2); if (!cards[index]) { cards[index] = true; return new int[] { suit, value }; } } } public static String cardToString(int[] card) { String suitStr = ""; switch (card[0]) { case 1: suitStr = "club"; break; case 2: suitStr = "diamond"; break; case 3: suitStr = "heart"; break; case 4: suitStr = "spade"; break; } String valueStr; switch (card[1]) { case 11: valueStr = "Jack"; break; case 12: valueStr = "Queen"; break; case 13: valueStr = "King"; break; case 14: valueStr = "Ace"; break; default: valueStr = Integer.toString(card[1]); break; } return valueStr + " of " + suitStr + "s"; } }
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
import java.util.*;
public class CardGame {
static Scanner scanner = new Scanner(System.in);
static Random random = new Random();
public static void main(String[] args) {
System.out.println("Welcome to the card game! Here are the instructions:");
System.out.println("- You will randomly draw a card from a deck of 52 cards.");
System.out.println("- The computer will also draw a card from the same deck.");
System.out.println("- If your card has a higher face value, you win. Otherwise, you lose.");
System.out.println("- If your card has the same face value as the computer's card, the suit determines the order.");
int gamesPlayed = 0;
int userWins = 0;
int computerWins = 0;
boolean[] cards = new boolean[52]; // false means the card is available, true means it's been drawn
while (true) {
System.out.println("\nNew game!");
int[] userCard = drawCard(cards);
int[] computerCard = drawCard(cards);
System.out.println("Your card is " + cardToString(userCard));
System.out.println("Computer's card is " + cardToString(computerCard));
int userValue = userCard[1];
int computerValue = computerCard[1];
if (userValue > computerValue) {
System.out.println("You win!");
userWins++;
} else if (userValue < computerValue) {
System.out.println("You lose!");
computerWins++;
} else {
// same face value, compare suits
int userSuit = userCard[0];
int computerSuit = computerCard[0];
if (userSuit > computerSuit) {
System.out.println("You win!");
userWins++;
} else {
System.out.println("You lose!");
computerWins++;
}
}
gamesPlayed++;
if (gamesPlayed == 26) {
System.out.println("\nYou have played 26 games, which is half of the deck.");
System.out.println("Do you want to shuffle the deck and start a new game? (y/n)");
String answer = scanner.next();
if (answer.equals("y")) {
Arrays.fill(cards, false);
gamesPlayed = 0;
userWins = 0;
computerWins = 0;
} else {
break;
}
} else {
System.out.println("\nDo you want to play another game? (y/n)");
String answer = scanner.next();
if (!answer.equals("y")) {
break;
}
}
}
System.out.println("\nThanks for playing!");
System.out.println("You played " + gamesPlayed + " games.");
System.out.println("You won " + userWins + " times.");
System.out.println("The computer won " + computerWins + " times.");
}
public static int[] drawCard(boolean[] cards) {
while (true) {
int suit = random.nextInt(4) + 1;
int value = random.nextInt(13) + 2;
int index = (suit - 1) * 13 + (value - 2);
if (!cards[index]) {
cards[index] = true;
return new int[] { suit, value };
}
}
}
public static String cardToString(int[] card) {
String suitStr = "";
switch (card[0]) {
case 1:
suitStr = "club";
break;
case 2:
suitStr = "diamond";
break;
case 3:
suitStr = "heart";
break;
case 4:
suitStr = "spade";
break;
}
String valueStr;
switch (card[1]) {
case 11:
valueStr = "Jack";
break;
case 12:
valueStr = "Queen";
break;
case 13:
valueStr = "King";
break;
case 14:
valueStr = "Ace";
break;
default:
valueStr = Integer.toString(card[1]);
break;
}
return valueStr + " of " + suitStr + "s";
}
}
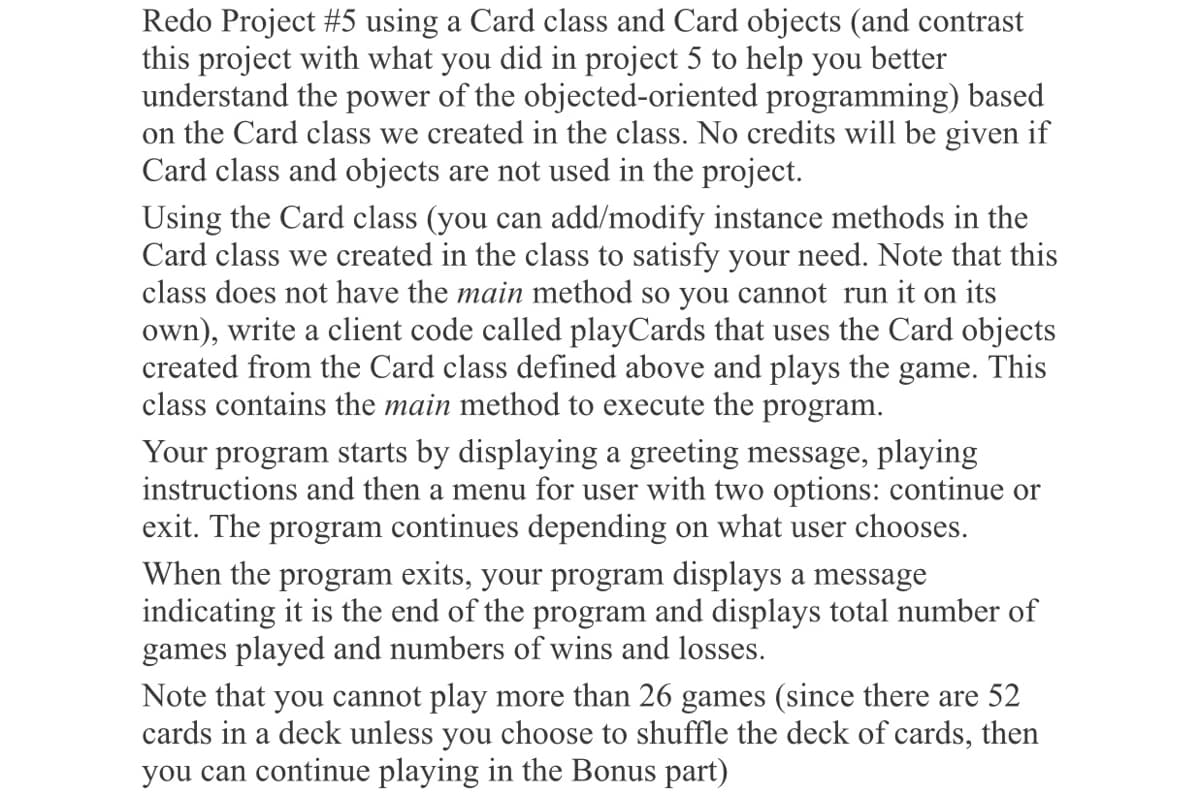
Transcribed Image Text:Redo Project #5 using a Card class and Card objects (and contrast
this project with what you did in project 5 to help you better
understand the power of the objected-oriented programming) based
on the Card class we created in the class. No credits will be given if
Card class and objects are not used in the project.
Using the Card class (you can add/modify instance methods in the
Card class we created in the class to satisfy your need. Note that this
class does not have the main method so you cannot run it on its
own), write a client code called playCards that uses the Card objects
created from the Card class defined above and plays the game. This
class contains the main method to execute the program.
Your program starts by displaying a greeting message, playing
instructions and then a menu for user with two options: continue or
exit. The program continues depending on what user chooses.
When the program exits, your program displays a message
indicating it is the end of the program and displays total number of
games played and numbers of wins and losses.
Note that you cannot play more than 26 games (since there are 52
cards in a deck unless you choose to shuffle the deck of cards, then
you can continue playing in the Bonus part)
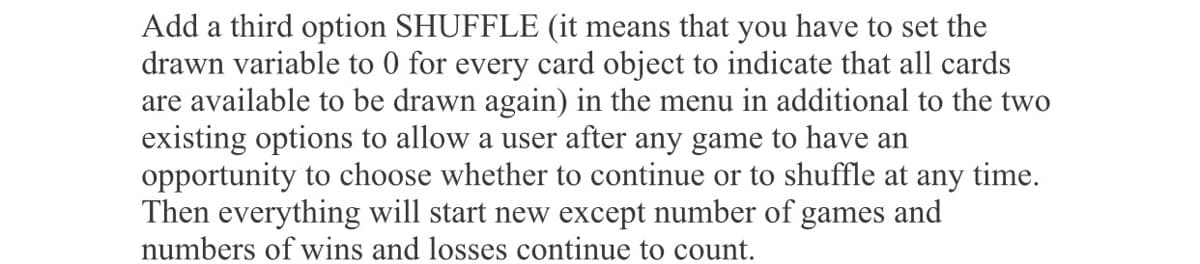
Transcribed Image Text:Add a third option SHUFFLE (it means that you have to set the
drawn variable to 0 for every card object to indicate that all cards
are available to be drawn again) in the menu in additional to the two
existing options to allow a user after any game to have an
opportunity to choose whether to continue or to shuffle at any time.
Then everything will start new except number of games and
numbers of wins and losses continue to count.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 5 steps with 6 images

Recommended textbooks for you
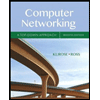
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
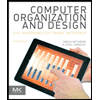
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
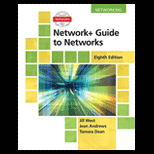
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
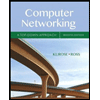
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
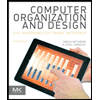
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
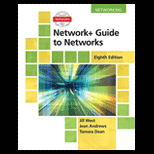
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
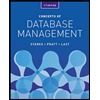
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
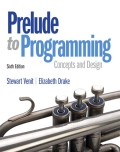
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
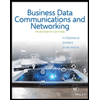
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY