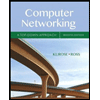
Write a C++ program using object-oriented principles that will display the National Champion of NCAA College Football for the year the user enters. The valid years that may be entered are from 1998 - 2022.
An input file - named NationalChampionship.txt - has been created that contains: the year and the school team that won the National Championship. It should be located in the current directory.
To begin, the program should declare two arrays: one array to hold the year and another array to hold the school and team name.
Populate the arrays with the values read in from the input file. Close the input file.
Next, the program should prompt the user to enter a year. Validate the user's input value. If the user enters an invalid year, display an error message and let the user retry as many times as needed.
When a good input year is entered, the program must find the corresponding year in the first array. Then, the program must look in the second array to find the name of the team that won the national championship for that year. Display that value.
Continue to prompt the user for another year until the user wishes to stop. Make it clear how you would like for the user to stop.
A sample output:
National Championship Inquiry Reading the input file... Enter a year between 1998 - 2022 to find the champion (press -99 to stop): 2008 The Florida Gators were the National Champions in 2008. Enter a year between 1998 - 2022 to find the champion (press -99 to stop): -1999 Invalid input, try again: two thousand Invalid input, try again: tell me!!!! Invalid input, try again: 2016 The Clemson Tigers were the National Champions in 2016. Enter a year between 1998 - 2022 to find the champion (press -99 to stop): -99 New Year, New Team, One Dream! |
At a minimum, the class should have:
private member variables:
an array that holds the year
an array to hold the school and team name
the input file
private member functions:
file maintenance (opening the file, testing the file opened properly, reading the file, closing the file)
display method
public member functions:
a constructor - if needed
driver method
main() should only contain the instantiated object of the class, a call to the driver method, a system pause command, and a return statement. Add comments.
NOTE: Any submitted program that does not use OOP appropriately will result in a grade of 0.
To Do:
- Upload your cpp file to the Project 7 Drop Box.
- Be sure to check the Rubrics before you submit your Project. Rubrics tell you exactly what must be included in each Project and how much each item is worth.
- Check the Calendar for the due date!
- Be sure to use the template code (the file called template code under Visual Studio in Modules).

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

- I am confused on how to go about writing this python program.arrow_forwardPROGRAM NEEDS TO MATCH EXAMPLE PHOTO Write a program ( lab6.cpp ) that gives and takes advice on program writing. The program starts by writing a piece of advice to the screen and asking the user to type in a different piece of advice. The program then ends. The next person to run the program receives the advice given by the person who last ran the program. The advice is kept in a file, and the contents of the file change after each run of the program. You can use your editor to enter the initial piece of advice in the file so that the first person who runs the program receives some advice. Allow the user to type in advice of any length so that it can be any number of lines long. The user is told to end his or her advice by pressing the Return key two times. Your program can then test to see that it has reached the end of the input by checking to see when it reads two consecutive occurrences of the character ‘\n’. Hints:- You need to look at each character as it is read to see if it is…arrow_forwardThis Python Lab 9 Lab: Write a file copying program. The program asks for the name of the file to copy from (source file) and the name of the file to copy to (destination file). The program opens the source file for reading and the destination file for writing. As the program reads each line from the source file and it writes the line to the destination file. When every line from the source file has been written to the destination file, it close both files and print “Copy is successful.” In the sample run, “add.py” is the source file and “add-copy.py” is the destination file. Note that both “add-copy.py” is identical to “add.py” because “add-copy.py” is a copy of “add.py”. Sample run: Enter file to copy from: add.py Enter file to copy to : add-copy.py Copy is successful. Source file: add.py print("This program adds two numbers") a = int(input("Enter first number: ")) b = int(input("Enter second number: ")) print(f"{a} + {b} = {a+b}") Destination file:…arrow_forward
- >> classicVinyls.cpp For the following program, you will use the text file called “vinyls.txt” attached to this assignment. The file stores information about a collection of classic vinyls. The records in the file are like the ones on the following sample: Led_Zeppelin Led_Zeppelin 1969 1000.00 The_Prettiest_Star David_Bowie 1973 2000.00 Speedway Elvis_Presley 1968 5000.00 Spirit_in_the_Night Bruce_Springsteen 1973 5000.00 … Write a declaration for a structure named vinylRec that is to be used to store the records for the classic collection system. The fields in the record should include a title (string), an artist (string), the yearReleased (int), and an estimatedPrice(double). Create the following…arrow_forwardwrite in c++Write a program that would allow the user to interact with a part of the IMDB movie database. Each movie has a unique ID, name, release date, and user rating. You're given a file containing this information (see movies.txt in "Additional files" section). The first 4 rows of this file correspond to the first movie, then after an empty line 4 rows contain information about the second movie and so forth. Format of these fields: ID is an integer Name is a string that can contain spaces Release date is a string in yyyy/mm/dd format Rating is a fractional number The number of movies is not provided and does not need to be computed. But the file can't contain more than 100 movies. Then, it should offer the user a menu with the following options: Display movies sorted by id Display movies sorted by release date, then rating Lookup a release date given a name Lookup a movie by id Quit the Program The program should perform the selected operation and then re-display the menu. For…arrow_forwardIn C++ PLEASE Use the text file from Chapter 12, forChap12.txt. SEE BELOW Write a program that opens a specified text file then displays a list of all the unique words found in the file. Addition to the text book specifications, print the total of unique words in the file. Text File = forChap12.txt No one is unaware of the name of that famous English shipowner, Cunard. In 1840 this shrewd industrialist founded a postal service between Liverpool and Halifax, featuring three wooden ships with 400-horsepower paddle wheels and a burden of 1,162 metric tons. Eight years later, the company's assets were increased by four 650-horsepower ships at 1,820 metric tons, and in two more years, by two other vessels of still greater power and tonnage. In 1853 the Cunard Co., whose mail-carrying charter had just been renewed, successively added to its assets the Arabia, the Persia, the China, the Scotia, the Java, and the Russia, all ships of top speed and, after the Great Eastern, the biggest ever…arrow_forward
- Can this be done in Java and not C++arrow_forwardJava Your program must read a file called personin.txt. Each line of the file will be a person's name, the time they arrived at the professor's office, and the amount of time they want to meet with the professor. These entries will be sorted by the time the person arrived. Your program must then print out a schedule for the day, printing each person's arrival, and printing when each person goes in to meet with the professor. You need to print the events in order of the time they happen. In other words, your output will be sorted by the arrival times and the times the person goes into the professor's office. In your output you need to print out a schedule. In the schedule, new students go to the end of the line. Whenever the professor is free, the professor will either meet with the first person in line, or meet with the first person in line if nobody is waiting. Assume no two people arrive at the same time. You should solve this problem using a stack and a queue. You can only…arrow_forwardDesign an application for the ABC Company that will process inventory from a file called ABC_Inventory.txt (attached to this assignment). The file contains Item ID, Description and list price stored on a separate line in the file. The program should display the contents of each record and then calculate and display the average list price. it is important that this is done in Thonny for python warrow_forward
- It contains data for one movie on each line.Each Movie contains the following data items:Title(String), Year(int-4 digits), Runtime(double). Write Java program(s) to do the following:1) Read the movies.txt Text file and write the data as a Serialized file called movies.ser to the local directory(same as movies.txt).2) Read the movies.ser serialized file and output the data on to the Console in the format shown below. The spacing for the output is: Title(20), Year (8) and Runtime (10.2)Title and Year are left-justified, Runtime is right-justified.(You can use your own text file to test your program) Sample output: Title Year Runtime aaaaaaaaaaa aaaa aa.aa...... ...... ........... ...... .....xxxxxxxx xxxx xx.xxyyyyyyy yyyy yyy.yyarrow_forwardWAP to read 4 student objects and write them into a file, then print total number of student number in file. After that read an integer and print details of student stored at this number in the file using random access and file manipulators.arrow_forwardIn Python Write a program that consists of (at least) two function: A function that creates and saves data in a file. The saved data represents exam grades. In the function, you will create n random numbers in the range [0,100], where n is the number of students. The function can be called as follows: createFile(filename, n) Main function, in which the user inputs the file name and the number of students, then the main calls function createFile. The main should perform validation for n (should be > 0), and the filename (should end with .txt). I will explain how to validate strings in the coming lecture. Hint: a good developer will write four functions to solve the problem.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
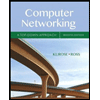
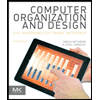
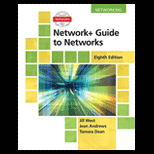
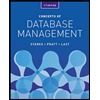
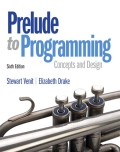
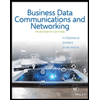