import java.util.Scanner; public class SortedNames { public static void main(String[] args) { String name1; String name2; String name3; Scanner keyboard=new Scanner(System.in); System.out.print("Please Enter First Name: "); name1=keyboard.nextLine(); System.out.print("Please Enter Second Name: "); name2=keyboard.nextLine(); System.out.print("Please Enter Third Name: "); name3=keyboard.nextLine(); if((name2.compareToIgnoreCase(name1)<0)&&(name2.compareToIgnoreCase(name3)<0)) { System.out.println(name2); } if((name1.compareToIgnoreCase(name2)<0)&&(name1.compareToIgnoreCase(name3)<0)) { System.out.println(name1); } if((name3.compareToIgnoreCase(name1)<0)&&(name3.compareToIgnoreCase(name2)<0)) { System.out.println(name3); } } }
Need helped with question 3 screenshot provided & my Java code work is typed below.
Step 1 -
import java.util.Scanner;
public class SortedNames {
public static void main(String[] args) {
String name1;
String name2;
String name3;
Scanner keyboard=new Scanner(System.in);
System.out.print("Please Enter First Name: ");
name1=keyboard.nextLine();
System.out.print("Please Enter Second Name: ");
name2=keyboard.nextLine();
System.out.print("Please Enter Third Name: ");
name3=keyboard.nextLine();
if((name2.compareToIgnoreCase(name1)<0)&&(name2.compareToIgnoreCase(name3)<0))
{
System.out.println(name2);
}
if((name1.compareToIgnoreCase(name2)<0)&&(name1.compareToIgnoreCase(name3)<0))
{
System.out.println(name1);
}
if((name3.compareToIgnoreCase(name1)<0)&&(name3.compareToIgnoreCase(name2)<0))
{
System.out.println(name3);
}
}
}
Step 2 -
Java program source code that prompts the user to enter a year value from the keyboard. Then check if the year is a leap year or not. Then find the year is a leap year or not.
//LeapyearDemo.java
import java.util.Scanner;
public class LeapyearDemo
{
public static void main(String[] args)
{
boolean leapYear=false;
//create an instance of the Scanner class to read input from keyboard
Scanner keyboard=new Scanner(System.in);
System.out.print("Please enter year value : ");
int year=Integer.parseInt(keyboard.nextLine());
/*Checking if year is multiple of 4 and not multiple of 100 then
* set the leapYear to true*/
if((year%4==0) && (year%100!=0))
leapYear=true;
/*checking if year is multiple of 400 then
* set leapYear to true otherwise set false*/
else if(year%400==0)
leapYear=true;
else
leapYear=false;
if(leapYear)
System.out.println(year+" is a leap year.");
else
System.out.println(year+" is not a leap year.");
}
}
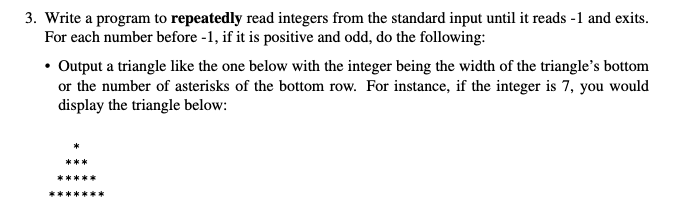

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 4 images

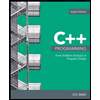
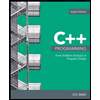