In the main class first Create three departments. Ask the user to input the field values. In the main class create 5 students. Ask the user to input the field values. Write a function in the main class that takes two integers as input and Checks if they are equal. Use that function to check if for a depart- ment and a student their DEP ID is equal. (So you have to check if dep1.di=student1.id for example). Create a university in the main again.(Initialize it the way you like).DO NOT ASK THE USER HAVE THEM DEFINED YOUR SELF SO NO SCANNER In the main class ask the user to input a string. Then until you have reached the end of the string iterate on each char and replace each char at location i (the location you are at in that iteration) with the following: If the location was an even location replace it with the lowercase version of the char and if it was odd replace it with its upper case. Example: heLlo: hElLo.
Please help with the following im really struggling, most of the work is done I need help with the last part its in JAVA not arrays or this.keword nor Integer.parseInt. try and keep as simple as possible.
package school;
import java.util.Scanner;
public class School {
/**
* @param args
*
*/
public static void main(String[] args) {
Scanner sc=new Scanner(System.in);
//department 1
System.out.println("Department name");
String dept1name = sc.nextLine();
System.out.println("Department ID");
int dept1id = sc.nextInt();
sc.nextLine();
//department 2
System.out.println("Department name");
String dept2name = sc.nextLine();
System.out.println("Department ID");
int dept2id = sc.nextInt();
sc.nextLine();
//department 3
System.out.println("Department name");
String dept3name = sc.nextLine();
System.out.println("Department ID");
int dept3id = sc.nextInt();
//Student 1
System.out.println("Student ID");
int stud1id = sc.nextInt();
sc.nextLine();
System.out.println("Student name");
String stud1name = sc.nextLine();
System.out.println("Student department Id");
int stud1deptid = sc.nextInt();
//Student 2
System.out.println("Student ID");
int stud2id = sc.nextInt();
sc.nextLine();
System.out.println("Student name");
String stud2name = sc.nextLine();
System.out.println("Student department name");
int stud2deptid = sc.nextInt();
//Student 3
System.out.println("Student ID");
int stud3id = sc.nextInt();
sc.nextLine();
System.out.println("Student name");
String stud3name = sc.nextLine();
System.out.println("Student department name");
int stud3deptid = sc.nextInt();
//Student 4
System.out.println("Student ID");
int stud4id = sc.nextInt();
sc.nextLine();
System.out.println("Student name");
String stud4name = sc.nextLine();
System.out.println("Student department ID");
int stud4deptid = sc.nextInt();
//Student 5
System.out.println("Student ID");
int stud5id = sc.nextInt();
sc.nextLine();
System.out.println("Student name");
String stud5name = sc.nextLine();
System.out.println("Student department ID");
int stud5deptid = sc.nextInt();
}
}
Department
public class Department {
private String Dep_Name;
private int Dep_Id;
public Department(String name, int id) {
Dep_Name = name;
Dep_Id = id;
}
/**
* Setters
*/
public void setDepName(String name) {
Dep_Name = name;
}
public void setDepId(int id) {
Dep_Id = id;
}
/**
* Getters
*/
public String getDepName() {
return Dep_Name;
}
public int getDepId() {
return Dep_Id;
}
}
Student
public class Student {
/**
* @param Private attributes
*
*/
private int ID;
private String name;
private int Dep_Id;
/**
* constructor for set attributes
*/
public Student(int id, String n, int dep) {
ID = id;
name = n;
Dep_Id = dep;
}
/**
* Setters
*/
public void setID(int id) {
ID = id;
}
public void setName(String n) {
name = n;
}
public void setDepId(int dep) {
Dep_Id = dep;
}
/**
* Getters
*/
public int getID() {
return ID;
}
public String getName() {
return name;
}
public int getDepId() {
return Dep_Id;
}
}
University
public class University{
/**
* Private attributes
*/
private String name;
private int population;
private double budget;
/**
* constructor for set attributes
*/
public University(String n, int p, double b){
name =n;
population =p;
budget = b;
}
/**
* Setters
*/
public void setName(String n){
name=n;
}
public void setPopulation(int p){
population=p;
}
public void setBudget(double b){
budget=b;
}
/**
* Getters
*/
public String getName(){
return name;
}
public int getPopulation(){
return population;
}
public double getBudget(){
return budget;
}
}
Part missing is in the picture
and here is what I tried but I can't make it work.
//create University instance
University u1 = new University("Oxford",4000,25000);
System.out.println("Student number 1 in Department 1" );
System.out.print("Input a String: ");
String str1=sc.nextLine(); //input string from user
String temp="";
for(int i=0;i<str1.length();i++){ //from 0 to last index
if(i%2==0) //if even position
temp+=Character.toLowerCase(str1.charAt(i)); //conavrt to lower case and add to temp
else //if odd position
temp+=Character.toUpperCase(str1.charAt(i)); //convert to upper case and add to temp
}
System.out.println(temp);
}
public static boolean equals(int n1,int n2){
return n1==n2; //return true if both are same else return false
try not to change any of what I have done in the classes It would be really nice if you could help me.
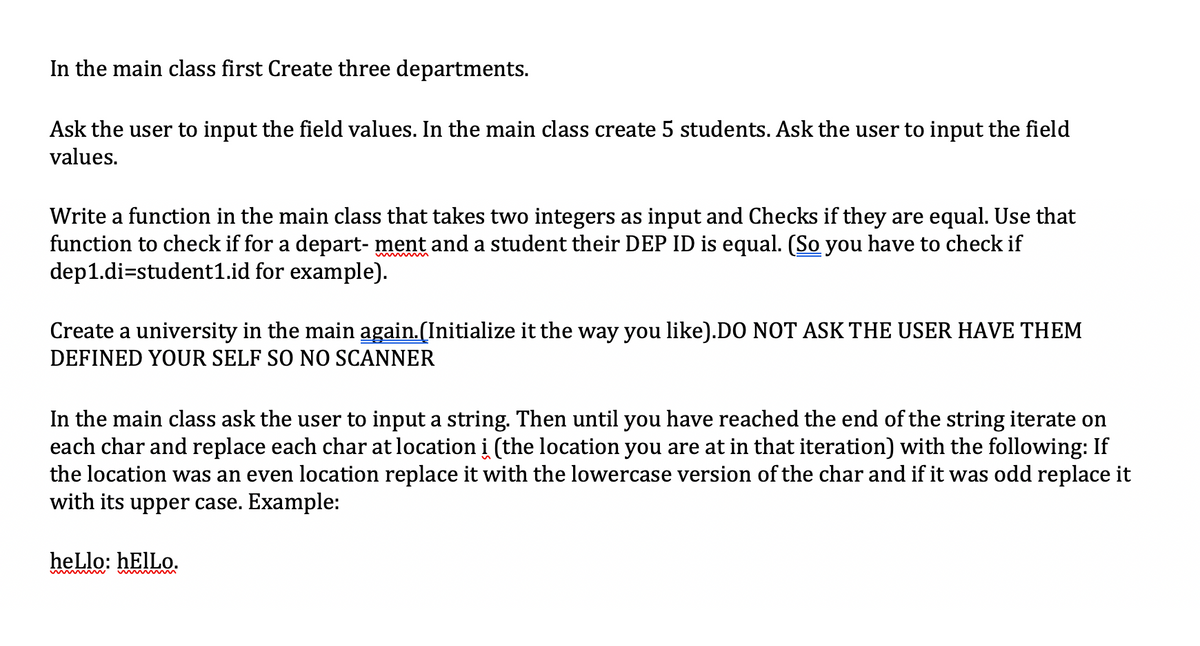

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

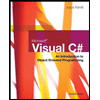
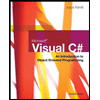