Improve the method printList to print out the details of all publications ordered on the category of the publications, and then on the title within each category. Note: currently there are only 2 categories, i.e. Book and Journal, but the project may be expended by adding other categories, e.g. Newspaper etc. Here are my four classes. public class Database { private ArrayList publicationList; // An array list collection of publications /** * Create a new database */ public Database() { publicationList = new ArrayList(); } /** * Add a publication * * @param publication The publication to be added */ public void addPublication(Publication publication) { if (publicationList.contains(publication)) { System.out.println("This publication has already been added to the list: " + publication); } else { publicationList.add(publication); } } /** * Get the total number of publications * * @return The total number of publications */ public int getTotal() { return publicationList.size(); } public ArrayList getBooksByAuthor(String authorName) { ArrayList authorsBooks = new ArrayList(); for (Publication publication : publicationList) { if (publication instanceof Book) { Book book = (Book) publication; // cast the publication to a book if (book.getAuthor().equals(authorName)) { // check if the author's name matches authorsBooks.add(book); // add the book to the list } } } return authorsBooks; } public int getNumberOfJournals(int month, int year) { int count = 0; for (Publication publication : publicationList) { if (publication instanceof Journal) { Journal journal = (Journal) publication; if (journal.getMonth() == month && journal.getYear() == year) { count++; } } } return count; } public void printList() { for (var aBook: bookList) { System.out.println(aBook); } for (var aJournal: journalList) { System.out.println(aJournal); } System.out.println("Total number of publications: " + getTotal()); } public class Publication { private String title; // The title of the book private int year; // The year when the book was published /** * Parameterized constructor for objects of class Publication * * @param title The title of the publication */ public Publication(String title, int year) { this.title = title; this.year = year; } /** * Get the title of the publication * * @return The title of the publication */ public String getTitle() { return title; } /** * Get the year when the journal was published * * @return The year when the journal was published */ public int getYear() { return year; } public String toString() { return title + ", " + year; } } public class Journal extends Publication { // The year and month when the journal was published private int month; /** * Create a journal. * * @param title The title of the journal * @param month The month when the journal was published * @param year The year when the journal was published */ public Journal(String title, int month, int year) { super(title, year); this.month = month; } /** * Get the month when the journal was published * * @return The month when the journal was published */ public int getMonth() { return month; } /** * Get the details of the journal * * @return The details of the journal, i.e. the title, year and month */ public String toString() { return super.toString() + " (" + getMonthName(month) + ")"; } /** * Check if the journal is the same as the given one. * * @param obj The given object. * * @return true if the journal and the given one have the * same title, year and month; * false otherwise */ public boolean equals(Object obj) { if (this == obj) return true; if ( !(obj instanceof Journal) ) return false; var another = (Journal) obj; return this.getTitle().equals(another.getTitle()) && this.month == another.month && this.getYear() == another.getYear(); } /** * To get the name of a given month * * @param month A given month * @return The month's name */ private String getMonthName(int month) { switch (month) { case 1: return "January"; case 2: return "February"; case 3: return "March"; case 4: return "April"; case 5: return "May"; case 6: return "June"; case 7: return "July"; case 8: return "August"; case 9: return "September"; case 10: return "October"; case 11: return "November"; case 12: return "December"; default: return "Unknown"; } } }
Improve the method printList to print out the details of all publications ordered on the category of the publications, and then on the title within each category. Note: currently there are only 2 categories, i.e. Book and Journal, but the project may be expended by adding other categories, e.g. Newspaper etc.
Here are my four classes.
public class
private ArrayList<Publication> publicationList; // An array list collection of publications
/**
* Create a new database
*/
public Database() {
publicationList = new ArrayList<Publication>();
}
/**
* Add a publication
*
* @param publication The publication to be added
*/
public void addPublication(Publication publication) {
if (publicationList.contains(publication)) {
System.out.println("This publication has already been added to the list: " + publication);
} else {
publicationList.add(publication);
}
}
/**
* Get the total number of publications
*
* @return The total number of publications
*/
public int getTotal() {
return publicationList.size();
}
public ArrayList<Book> getBooksByAuthor(String authorName) {
ArrayList<Book> authorsBooks = new ArrayList<Book>();
for (Publication publication : publicationList) {
if (publication instanceof Book) {
Book book = (Book) publication; // cast the publication to a book
if (book.getAuthor().equals(authorName)) { // check if the author's name matches
authorsBooks.add(book); // add the book to the list
}
}
}
return authorsBooks;
}
public int getNumberOfJournals(int month, int year) {
int count = 0;
for (Publication publication : publicationList) {
if (publication instanceof Journal) {
Journal journal = (Journal) publication;
if (journal.getMonth() == month && journal.getYear() == year) {
count++;
}
}
}
return count;
}
public void printList() {
for (var aBook: bookList) {
System.out.println(aBook);
}
for (var aJournal: journalList) {
System.out.println(aJournal);
}
System.out.println("Total number of publications: " + getTotal());
}
public class Publication {
private String title; // The title of the book
private int year; // The year when the book was published
/**
* Parameterized constructor for objects of class Publication
*
* @param title The title of the publication
*/
public Publication(String title, int year) {
this.title = title;
this.year = year;
}
/**
* Get the title of the publication
*
* @return The title of the publication
*/
public String getTitle() {
return title;
}
/**
* Get the year when the journal was published
*
* @return The year when the journal was published
*/
public int getYear()
{
return year;
}
public String toString() {
return title + ", " + year;
}
}
public class Journal extends Publication
{
// The year and month when the journal was published
private int month;
/**
* Create a journal.
*
* @param title The title of the journal
* @param month The month when the journal was published
* @param year The year when the journal was published
*/
public Journal(String title, int month, int year)
{
super(title, year);
this.month = month;
}
/**
* Get the month when the journal was published
*
* @return The month when the journal was published
*/
public int getMonth()
{
return month;
}
/**
* Get the details of the journal
*
* @return The details of the journal, i.e. the title, year and month
*/
public String toString()
{
return super.toString() + " (" + getMonthName(month) + ")";
}
/**
* Check if the journal is the same as the given one.
*
* @param obj The given object.
*
* @return true if the journal and the given one have the
* same title, year and month;
* false otherwise
*/
public boolean equals(Object obj) {
if (this == obj) return true;
if ( !(obj instanceof Journal) ) return false;
var another = (Journal) obj;
return this.getTitle().equals(another.getTitle()) &&
this.month == another.month &&
this.getYear() == another.getYear();
}
/**
* To get the name of a given month
*
* @param month A given month
* @return The month's name
*/
private String getMonthName(int month) {
switch (month) {
case 1: return "January";
case 2: return "February";
case 3: return "March";
case 4: return "April";
case 5: return "May";
case 6: return "June";
case 7: return "July";
case 8: return "August";
case 9: return "September";
case 10: return "October";
case 11: return "November";
case 12: return "December";
default: return "Unknown";
}
}
}

Step by step
Solved in 3 steps with 1 images

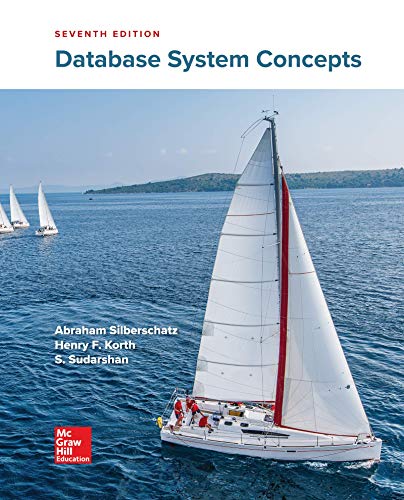
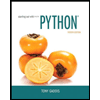
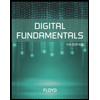
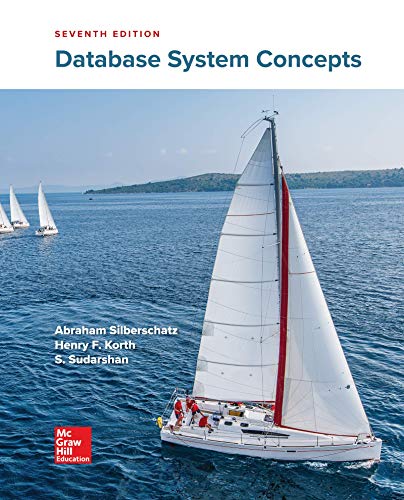
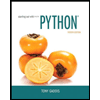
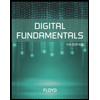
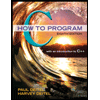
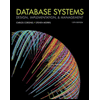
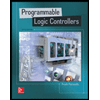