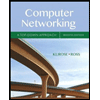
For the first part of this lab, copy your working ArrayStringList code into the GenericArrayList class.(already in the code) Then, modify the class so that it can store any type someone asks for, instead of only Strings. You shouldn't have to change any of the actual logic in your class to accomplish this, only type declarations (i.e. the types of parameters, return types, etc.)
Note:
In doing so, you may end up needing to write something like this (where T is a generic type):
T[] newData = new T[capacity];
...and you will find this causes a compiler error. This is because Java dislikes creating new objects of a generic type. In order to get around this error, you can write the line like this instead:
T[] new Data = (T[]) new Object[capacity]
This creates an array of regular Objects which are then cast to the generic type. It works and it doesn't anger the Java compiler. How amazing!
Once you're done, screenshot or save your code for checkin later.
For the second part of the lab, modify your GenericArrayList so that it can store any type that is comparable to a Point. Remember the Point and Point3D classes? Both of those implement the Comparable<Point> interface, so they both can compared to a Point. In fact, they are the only classes that can be compared to a Point, so after modifying your GenericArrayList, it should only be able to contain these two classes.
In both parts, test your classes by following the directions in the comments. They will ask you to uncomment some code and look for a specific result.
public class GenericArrayList {
/* YOUR CODE HERE
* Copy your code from your ArrayStringList class, and place it within
* this class.
*
* Only copy the code you filled out! Don't copy the main method.
*/
// Place code here
public class ArrayStringList {
private String[] data;
private int size;
private void resizeData(int newSize) {
String[] str = new String[newSize];
for(int i = 0; i < size; i++) {
str[i] = data[i];
}
data=str;
}
public ArrayStringList(int initialCapacity) {
data = new String[initialCapacity];
size = 0;
}
public void add(String str) {
if(size < data.length) {
data[size] = str;
size++;
} else {
resizeData(2 * data.length);
data[size] = str;
size++;
}
}
public void add(int index, String str) {
if(index < data.length && index >= 0) {
data[index] = str;
size++;
}
}
public String get(int index) {
if(index < data.length && index >= 0) {
return data[index];
}
return null;
}
public void remove(int index) {
if(index < data.length && index >= 0) {
for(int i = index; i < data.length; i++) {
if((i + 1) < size) {
data[i] = data[i + 1];
}
}
size--;
}
}
public int size() {
return size;
}
public boolean contains(String str) {
for(int i = 0; i < data.length; i++) {
if(str.equals(data[i])) {
return true;
}
}
return false;
}
public static void main(String[] args) {
/* PART 1:
* Modify the GenericArrayList above so that it can store *any* class,
* not just strings.
* When you've done that, uncomment the block of code below, and see if
* it compiles. If it does, run it. If there are no errors, you did
* it right!
*/
GenericArrayList<Point> pointList = new GenericArrayList<Point>(2);
pointList.add(new Point(0, 0));
pointList.add(new Point(2, 2));
pointList.add(new Point(7, 0));
pointList.add(new Point(19.16f, 22.32f));
pointList.remove(0);
Point p = pointList.get(2);
if (p.x != 19.16f && p.y != 22.32f) {
throw new AssertionError("Your GenericArrayList compiled properly "
+ "but is not correctly storing things. Make sure you didn't "
+ "accidentally change any of your ArrayStringList code, aside "
+ "from changing types.");
}
GenericArrayList<Float> floatList = new GenericArrayList<Float>(2);
for (float f = 0.0f; f < 100.0f; f += 4.3f) {
floatList.add(f);
}
float f = floatList.get(19);
System.out.println("Hurray, everything worked!");
/* PART 2:
* Now, modify your GenericArrayList again so that it can only store
* things that are comparable to a Point.
*
* If you don't know how to do this, reference zybooks and your textbook
* for help.
*
* When you are ready to test it, uncomment the code above and run the
* code below.
*/
/*
GenericArrayList<Point> pointList = new GenericArrayList<Point>(2);
GenericArrayList<Point3D> pointList3D = new GenericArrayList<Point3D>(3);
pointList.add(new Point(0, 0));
pointList.add(new Point(2, 2));
pointList.add(new Point(7, 0));
pointList.add(new Point(19.16f, 22.32f));
pointList3D.add(new Point3D(1.0f, 2.0f, 3.0f));
pointList3D.add(new Point3D(7.3f, 4, 0));
Point p = pointList.get(2);
Point3D p3 = pointList3D.get(0);
// You should get a compilation error on this line!
GenericArrayList<Float> floatList = new GenericArrayList<Float>(2);
*/
}
}
}

In this question we have to modify a Java class called GenericArrayList to make it generic, allowing it to store any type of data.
Initially, the class is based on an existing ArrayStringList class that can only store strings. The goal is to transform it into a more versatile generic class.
Let's understand and hope this helps, If you have any queries please utilize threaded question feature.
Step by stepSolved in 3 steps

- Hi, I am making a elevator simulation and I need help in making the passenger classes using polymorphism, I am not sure what to implement in the comment that says logic. Any help is appreciated. There are 4 types of passengers in the system:Standard: This is the most common type of passenger and has a request percentage of 70%. Standard passengers have no special requirements.VIP: This type of passenger has a request percentage of 10%. VIP passengers are given priority and are more likely to be picked up by express elevators.Freight: This type of passenger has a request percentage of 15%. Freight passengers have large items that need to be transported and are more likely to be picked up by freight elevators.Glass: This type of passenger has a request percentage of 5%. Glass passengers have fragile items that need to be transported and are more likely to be picked up by glass elevators. This is what i have so far public abstract class Passenger { protected String type; protected int…arrow_forwardDeeper Class Design - the Square Class In this section, your job will be to write a new class that will adhere to the methods and data outlined in the “members” section below (said another way, your classes will implement a specific set of functions (called an interface) that we’ll describe contractually, ahead of time). These classes will all be shapes, with actions (methods) that are appropriate to shape objects, such as “getArea()”, or “draw()”. First we build a Square class that contains the following data and methods listed below, and then later, we will copy it to quickly make a Circle class. Note: when considering each data item below, think about what type of data would best represent the concept we’re trying to model in our software. For example, all shapes will have a coordinate pair indicating their location in a Cartesian coordinate system. This x and y pair may be modeled as ints or floats, and you might choose between the two depending on what the client application will…arrow_forwardPig Laten: Pig Latin is a farcical "language" used to entertain children, but also to teach them some valuable language concepts along the way. Translating English to Pig Latin is simple: Your challenge is to implement the method `pigLatinize` that takes a string phrase and translates it to Pig Latin. You're free to add additional classes, variables, and methods if you would like.arrow_forward
- Say we are about to build an ArrayList. Your ArrayList should guarantee that the array capacity is at most four times the number of elements. What would you like to do to maintain such a limit on the capacity? What is the benefit of using iterators? Can you describe your first experience of GUIs? And could you describe what is the advantage of using GUIs over Command-Line Interface (CLI) operations?arrow_forwardPlease look at the images for the programming question. I am not sure sure where I should start on this. All coding is done in Java, using IntelliJ Idea.arrow_forwardFor this exercise, we are going to revisit the Pie superclass that we saw as an example in the last lesson. We have added the Pumpkin class, which takes the number of slices and a boolean to indicate if it is made with canned pumpkin. We also added a getType() method in the Pie class. For this exercise, you need to create either an Array or an ArrayList and add 3 pies to that list, an Apple, a Pumpkin, and a third type using the Pie class. After adding these to your list, loop through and print out the type using the getType() method in the pie class. Sample Output Pie: Pumpkin Pie: Apple Pie: Blueberryarrow_forward
- A for construct is used to build a loop that processes a list of elements in programming. To do this, it continues to operate forever as long as there are objects to process. Is this assertion truthful or false? Explain your response.arrow_forwardComplete the Course class by implementing the courseSize() method, which returns the total number of students in the course. Given classes: Class LabProgram contains the main method for testing the program. Class Course represents a course, which contains an ArrayList of Student objects as a course roster. (Type your code in here.) Class Student represents a classroom student, which has three fields: first name, last name, and GPA. Ex. For the following students: Henry Bendel 3.6 Johnny Min 2.9 the output is: Course size: 2 public class LabProgram { public static void main (String [] args) { Course course = new Course(); // Example students for testing course.addStudent(new Student("Henry", "Bendel", 3.6)); course.addStudent(new Student("Johnny", "Min", 2.9)); System.out.println("Course size: " + course.courseSize()); } } public class Student { private String first; // first name private String last; // last name private…arrow_forwardWrite a method for the farmer class that allows a farmer object to pet all cows on the farm. Do not use arrays.arrow_forward
- Override the testOverriding() method in the Triangle class. Make it print “This is theoverridden testOverriding() method”. (add it to triangle class don't make a new code based on testOverriding in SimpleGeometricObject class.) (this was the step before this one) Add a void testOverriding() method to the SimpleGeometricObject class. Make it print“This is the testOverriding() method of the SimpleGeometricObject class”arrow_forward4. Say we wanted to get an iterator for an ArrayList and use it to loop over all items and print them to the console. What would the code look like for this? 5. Write a method signature for a method called foo that takes an array as an argument. The return type is void. 6. What is the difference between remove and clear in ArrayLists.arrow_forwardjava please dont take other website'answer. rthis is actually practice question ANIMALCLASS Create an Animal class. Each animal has a name, an x and y integer coordinate. The Animal class should have at minimum the following methodsbelowbut you may want to add more if necessary: Also note, everyanimal will need to have to have “z”passed to it so that it knows how big the map is. •A constructor that starts the animal at 0,0 with a name of "Unknown Animal"and only accepts a single int value (int mapSize). •A parameter constructor that allows the programmerto input all 4pieces of information.(x,y, name, mapSize)oCheck the parameters for valid input based on the constraints. Ifany of the input valuesis invalid, adjust it any way you deem necessary in order to make it valid. •getX()and getY() •getName() •toString(). o This should print out the name and coordinates of the animal. •touching(Animal x) This method should determine if the animal is on the same spot as a secondanimal(x). It…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
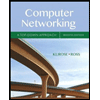
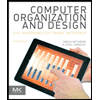
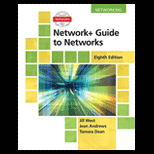
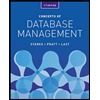
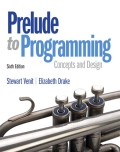
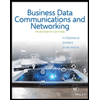