in C# Create a Bank class with the following properties: List of bank accounts (List) Create the following methods in the Bank class: AddAccount(BankAccount account): Method to add a new bank account to the list of accounts RemoveAccount(string accountNumber): Method to remove an existing bank account from the list of accounts SearchAccount(string accountNumber): Method to search for an existing bank account and return the account details my code is // This class represents a bank, which has a list of BankAccount objects as one of its properties. public class Bank { // This property holds a list of BankAccount objects belonging to the bank. public List Accounts { get; set; } // This constructor initializes the list of accounts for the bank. public Bank() { Accounts = new List(); } // This method adds a new BankAccount object to the bank's list of accounts. public void AddAccount(BankAccount account) { Accounts.Add(account); } // This method removes a BankAccount object with the given account number from the bank's list of accounts. // It returns true if the account was found and removed, and false otherwise. public bool RemoveAccount(string accountNumber) { // Find the BankAccount object with the given account number. BankAccount accountToRemove = Accounts.Find(account => account.AccountNumber == accountNumber); // If such an account was found, remove it from the list and return true. if (accountToRemove != null) { Accounts.Remove(accountToRemove); return true; } // If no account was found, return false. return false; } // This method searches for a BankAccount object with the given account number in the bank's list of accounts. // It returns the BankAccount object if found, and null otherwise. public BankAccount SearchAccount(string accountNumber) { // Find the BankAccount object with the given account number. BankAccount account = Accounts.Find(account => account.AccountNumber == accountNumber); // Return the found account, or null if no account was found. return account; } } my errors are in the pic
in C#
-
Create a Bank class with the following properties:
- List of bank accounts (List<BankAccount>)
-
Create the following methods in the Bank class:
- AddAccount(BankAccount account): Method to add a new bank account to the list of accounts
- RemoveAccount(string accountNumber): Method to remove an existing bank account from the list of accounts
- SearchAccount(string accountNumber): Method to search for an existing bank account and return the account details
my code is
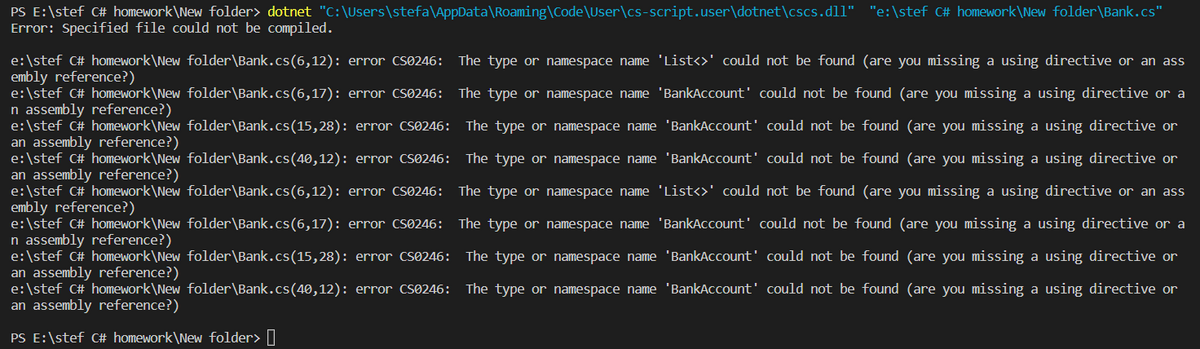

Solution:
Given,
These errors indicate that the compiler is unable to find the definition for the List<>
and BankAccount
types. You need to make sure that you have the necessary using directives and references in your code.
Make sure that you have added the following using directives at the top of your file:
using System.Collections.Generic;
Remark:
Also, make sure that you have a separate file for the BankAccount
class, and that it is defined in the same namespace as the Bank
class. If the BankAccount
class is defined in a different namespace, you will need to add a using directive for that namespace as well.
If you have done these things correctly and are still getting the errors, you may need to check that the file paths and project references in your Visual Studio solution are set up correctly.
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

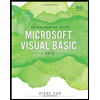
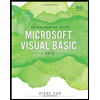