in C# i need to Create an application named TestSoccerPlayer that instantiates and displays a SoccerPlayer object. The SoccerPlayer class contains the following properties: Name - The player’s name ( a string) JerseyNum - The player's jersey number (an integer) Goals - Number of goals scored (an integer) Assists - Number of assists (an integer) my errors are Unit Test Incomplete Set and get the Name property Build Status Build Failed Build Output Compilation failed: 1 error(s), 0 warnings NtTestd94a3da4.cs(12,37): error CS1729: The type `SoccerPlayer' does not contain a constructor that takes `0' arguments TestSoccerPlayer.cs(22,12): (Location of the symbol related to previous error) Test Contents [TestFixture] public class SoccerPlayerClassTest { [Test] public void SoccerPlayerTest() { SoccerPlayer mySoccerPlayer = new SoccerPlayer(); mySoccerPlayer.Name = "Lionel"; mySoccerPlayer.JerseyNum = 9; mySoccerPlayer.Goals = 8; mySoccerPlayer.Assists = 35; Assert.AreEqual("Lionel", mySoccerPlayer.Name, "Unable to set or get the 'SoccerPlayer.Name' value. "); } } Unit TestIncomplete Set and get the JerseyNum property Build Status Build Failed Build Output Compilation failed: 1 error(s), 0 warnings NtTest4182d6f7.cs(12,37): error CS1729: The type `SoccerPlayer' does not contain a constructor that takes `0' arguments TestSoccerPlayer.cs(22,12): (Location of the symbol related to previous error) Test Contents [TestFixture] public class SoccerPlayerClassTest { [Test] public void SoccerPlayerTest() { SoccerPlayer mySoccerPlayer = new SoccerPlayer(); mySoccerPlayer.Name = "Lionel"; mySoccerPlayer.JerseyNum = 9; mySoccerPlayer.Goals = 8; mySoccerPlayer.Assists = 35; Assert.AreEqual(9, mySoccerPlayer.JerseyNum, "Unable to set or get the 'SoccerPlayer.JerseyNum' value. "); } } Unit TestIncomplete Set and get the Goals property Build Status Build Failed Build Output Compilation failed: 1 error(s), 0 warnings NtTestb53c2012.cs(12,37): error CS1729: The type `SoccerPlayer' does not contain a constructor that takes `0' arguments TestSoccerPlayer.cs(22,12): (Location of the symbol related to previous error) Test Contents [TestFixture] public class SoccerPlayerClassTest { [Test] public void SoccerPlayerTest() { SoccerPlayer mySoccerPlayer = new SoccerPlayer(); mySoccerPlayer.Name = "Lionel"; mySoccerPlayer.JerseyNum = 9; mySoccerPlayer.Goals = 8; mySoccerPlayer.Assists = 35; Assert.AreEqual(8, mySoccerPlayer.Goals, "Unable to set or get the 'SoccerPlayer.Goals' value. "); } } Unit TestIncomplete Set and get the Assists property Build Status Build Failed Build Output Compilation failed: 1 error(s), 0 warnings NtTest4c3b83e0.cs(12,37): error CS1729: The type `SoccerPlayer' does not contain a constructor that takes `0' arguments TestSoccerPlayer.cs(22,12): (Location of the symbol related to previous error) Test Contents [TestFixture] public class SoccerPlayerClassTest { [Test] public void SoccerPlayerTest() { SoccerPlayer mySoccerPlayer = new SoccerPlayer(); mySoccerPlayer.Name = "Lionel"; mySoccerPlayer.JerseyNum = 9; mySoccerPlayer.Goals = 8; mySoccerPlayer.Assists = 35; Assert.AreEqual(35, mySoccerPlayer.Assists, "Unable to set or get the 'SoccerPlayer.Assists' value. "); } } this is my code using static System.Console; class TestSoccerPlayer { static void Main() { SoccerPlayer player = new SoccerPlayer("Lionel Messi", 10, 32, 16); WriteLine("Player Name: " + player.Name); WriteLine("Jersey Number: " + player.JerseyNum); WriteLine("Goals: " + player.Goals); WriteLine("Assists: " + player.Assists); } } class SoccerPlayer { public string Name { get; set; } public int JerseyNum { get; set; } public int Goals { get; set; } public int Assists { get; set; } public SoccerPlayer(string name, int jerseyNum, int goals, int assists) { Name = name; JerseyNum = jerseyNum; Goals = goals; Assists = assists; } }
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
in C# i need to
Create an application named TestSoccerPlayer that instantiates and displays a SoccerPlayer object. The SoccerPlayer class contains the following properties:
- Name - The player’s name ( a string)
- JerseyNum - The player's jersey number (an integer)
- Goals - Number of goals scored (an integer)
- Assists - Number of assists (an integer)
my errors are
Set and get the Name property
Set and get the JerseyNum property
Set and get the Goals property
Set and get the Assists property

Algorithm:
Step 1 Start.
Step 2 Create a class "SoccerPlayer" with properties "Name", "JerseyNum", "Goals" and "Assists".
Step 3 Implement a default constructor for the class.
Step 4 Implement another constructor that takes in parameters for "Name", "JerseyNum", "Goals", and "Assists".
Step 5 In the main method, create an instance of the "SoccerPlayer" class and assign values to its properties.
Step 6 Output the values of the properties on the console.
Step 7 End.
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

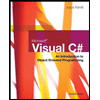
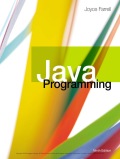
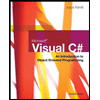
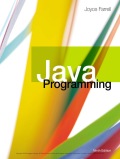
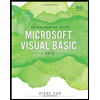
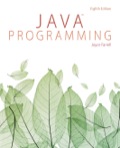