in c++ i need to implement the first public function and the driver code Implement a BigNumber.cpp to go with the following BigNumber.h. Meaning of operations is the usual meaning as for ints. Upload your BigNumber.cpp along with t BigNumber.h and a driver that tests each function at least once. For the multiplication, division and remainder operators you may use repeated addition and subtraction. You'll get up to 10 points extra credit if you implement those operations more efficiently. As a testcase for multiplication you should try 449! (That's a number with just under 1000 digits.) For operator>> and the string constructor, just skip over characters that aren't legal (e.g. a nondigit within the number). The only nondigit that's allowed is a single minus sign in front to indicate that the number is negative. You may modify BigNumber.h by adding prototypes for helper functions, if needed. But don't change prototypes for the functions that are already there #ifndef BIGNUMBER_H #define BIGNUMBER_H #include #include #include using namespace std; class BigNumber { friend ostream& operator<<(ostream& out, const BigNumber& c); friend istream& operator>>(istream& in, BigNumber& c); public: static const int MAXDIGITS = 1000; private: char digits[MAXDIGITS]; // The number may have up to MAXDIGITS digits bool negative; // true for negative numbers and false otherwise. Always false if the number is 0. int numDigits; // You may want to give numdigits the value 0 if the number is 0. public: BigNumber(int value = 0); BigNumber(string s); void setValue(int n); void setValue(const string& s); // e.g. if s is "-45" then the number is -45. int getNumDigits() const; double toDouble() const; // Return a double that approximates the current object. BigNumber& operator++(); BigNumber operator++(int n); bool operator> (const BigNumber& c) const; bool operator< (const BigNumber& c) const; bool operator>= (const BigNumber& c) const; bool operator<= (const BigNumber& c) const; bool operator== (const BigNumber& c) const; bool operator!= (const BigNumber& c) const; BigNumber operator-() const; // unary minus (return BigNumber that's additive opposite of *this, e.g. -20 --> 20) BigNumber& operator--(); BigNumber operator--(int n); BigNumber& operator+=(const BigNumber& c); BigNumber operator+(const BigNumber& c); BigNumber& operator-=(const BigNumber& c); BigNumber operator-(const BigNumber& c); BigNumber& operator*=(const BigNumber& c); BigNumber operator*(const BigNumber& c); BigNumber& operator/=(const BigNumber& c); BigNumber operator/(const BigNumber& c); BigNumber& operator%=(const BigNumber& c); BigNumber operator%(const BigNumber& c); }; #endif
in c++ i need to implement the first public function and the driver code
Implement a BigNumber.cpp to go with the following BigNumber.h. Meaning of operations is the usual meaning as for ints. Upload your BigNumber.cpp along with t BigNumber.h and a driver that tests each function at least once.
For the multiplication, division and remainder operators you may use repeated addition and subtraction. You'll get up to 10 points extra credit if you implement those operations more efficiently. As a testcase for multiplication you should try 449! (That's a number with just under 1000 digits.)
For operator>> and the string constructor, just skip over characters that aren't legal (e.g. a nondigit within the number). The only nondigit that's allowed is a single minus sign in front to indicate that the number is negative.
You may modify BigNumber.h by adding prototypes for helper functions, if needed. But don't change prototypes for the functions that are already there
#ifndef BIGNUMBER_H
#define BIGNUMBER_H
#include <istream>
#include <ostream>
#include <string>
using namespace std;
class BigNumber
{
friend ostream& operator<<(ostream& out, const BigNumber& c);
friend istream& operator>>(istream& in, BigNumber& c);
public:
static const int MAXDIGITS = 1000;
private:
char digits[MAXDIGITS]; // The number may have up to MAXDIGITS digits
bool negative; // true for negative numbers and false otherwise. Always false if the number is 0.
int numDigits; // You may want to give numdigits the value 0 if the number is 0.
public:
BigNumber(int value = 0);
BigNumber(string s);
void setValue(int n);
void setValue(const string& s); // e.g. if s is "-45" then the number is -45.
int getNumDigits() const;
double toDouble() const; // Return a double that approximates the current object.
BigNumber& operator++();
BigNumber operator++(int n);
bool operator> (const BigNumber& c) const;
bool operator< (const BigNumber& c) const;
bool operator>= (const BigNumber& c) const;
bool operator<= (const BigNumber& c) const;
bool operator== (const BigNumber& c) const;
bool operator!= (const BigNumber& c) const;
BigNumber operator-() const; // unary minus (return BigNumber that's additive opposite of *this, e.g. -20 --> 20)
BigNumber& operator--();
BigNumber operator--(int n);
BigNumber& operator+=(const BigNumber& c);
BigNumber operator+(const BigNumber& c);
BigNumber& operator-=(const BigNumber& c);
BigNumber operator-(const BigNumber& c);
BigNumber& operator*=(const BigNumber& c);
BigNumber operator*(const BigNumber& c);
BigNumber& operator/=(const BigNumber& c);
BigNumber operator/(const BigNumber& c);
BigNumber& operator%=(const BigNumber& c);
BigNumber operator%(const BigNumber& c);
};
#endif

Trending now
This is a popular solution!
Step by step
Solved in 4 steps

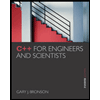
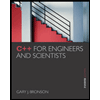