in c++ Part 1: Design a class called NumDays. The class’s purpose is to store a value that represents a number of work hours and convert it to a number of days. For example, 8 hours would be converted to 1 day, 12 hours would be converted to 1.5 days, and 18 hours would be converted to 2.25 days. The class should have a constructor that accepts a number of hours, as well as member functions for storing and retrieving the hours and days. The class should also have the following overloaded operators: • (+) Addition operator. When two NumDays objects are added together, the over- loaded + operator should return the sum of the two objects’ hours members. • (-) Subtraction operator. When one NumDays object is subtracted from another, the overloaded − operator should return the difference of the two objects’ hours members. Part 2: Design a class named TimeOff. The purpose of the class is to track an employee’s sick leave, vacation, and unpaid time off. It should have, as members, the following instances of the NumDays class described in part 1: • maxSickDays: A NumDays object that records the maximum number of days of sick leave the employee may take. • sickTaken: A NumDays object that records the number of days of sick leave the employee has already taken. • maxVacation: A NumDays object that records the maximum number of days of paid vacation the employee may take. • vacTaken: A NumDays object that records the number of days of paid vacation the employee has already taken. • maxUnpaid: A NumDays object that records the maximum number of days of unpaid vacation the employee may take. • unpaidTaken: A NumDays object that records the number of days of unpaid leave the employee has taken. Additionally, the class should have members for holding the employee’s name and identification number. It should have an appropriate constructor and member functions for storing and retrieving data in any of the member objects. Input Validation: Company policy states that an employee may not accumulate more than 240 hours of paid vacation. The class should not allow the maxVacation object to store a value greater than this amount. Part 3: Write a program that uses an instance of the TimeOff class you designed in Parts 1 and 2. The program should ask the user to enter the number of months an employee has worked for the company. It should then use the TimeOff object to calculate and display the employee’s maximum number of sick leave and vacation days. Employees earn 12 hours of vacation leave and 8 hours of sick leave per month.
in c++
Part 1: Design a class called NumDays. The class’s purpose is to store a value that represents a number of work hours and convert it to a number of days. For example, 8 hours would be converted to 1 day, 12 hours would be converted to 1.5 days, and 18 hours would be converted to 2.25 days. The class should have a constructor that accepts a number of hours, as well as member functions for storing and retrieving the hours and days. The class should also have the following overloaded operators: • (+) Addition operator. When two NumDays objects are added together, the over- loaded + operator should return the sum of the two objects’ hours members. • (-) Subtraction operator. When one NumDays object is subtracted from another, the overloaded − operator should return the difference of the two objects’ hours members. Part 2:
Design a class named TimeOff. The purpose of the class is to track an employee’s sick leave, vacation, and unpaid time off. It should have, as members, the following instances of the NumDays class described in part 1:
• maxSickDays: A NumDays object that records the maximum number of days of sick leave the employee may take. • sickTaken: A NumDays object that records the number of days of sick leave the employee has already taken. • maxVacation: A NumDays object that records the maximum number of days of paid vacation the employee may take. • vacTaken: A NumDays object that records the number of days of paid vacation the employee has already taken. • maxUnpaid: A NumDays object that records the maximum number of days of unpaid vacation the employee may take. • unpaidTaken: A NumDays object that records the number of days of unpaid leave the employee has taken. Additionally, the class should have members for holding the employee’s name and identification number. It should have an appropriate constructor and member functions for storing and retrieving data in any of the member objects. Input Validation: Company policy states that an employee may not accumulate more than 240 hours of paid vacation. The class should not allow the maxVacation object to store a value greater than this amount.
Part 3: Write a

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

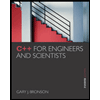
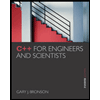