write c++ program Employee class has the following data members: Employee ID. Employee Name Department Employee Salary Static data member which will hold the value of total no. of employees Employee class must have the following member functions: Function Description Employee() Default constructor for Employee class, which will set employee id to 0, employee name to NULL, employee department to NULL and salary to 0.0 Employee(int, char *, char *, float); It will take employee id, employee name, department and salary as arguments and initialize their values using member initializer list Employee(Employee &); Copy constructor which will initialize one object with another object using deep copy Setter functions You have to define setter function for each data member which will take a value as an argument and set their values Getter functions You have to define getter function for each data member which will return the values of their data members calcNetPay() This function will calculate the net pay of an employee based on his salary display() This function will display the values of all data members of class ~Employee() Destructor of Employee class which will decrement the value of static data member of class calcNetPay(): This function will calculate net pay of an employee based on his salary as: If salary is less than and equal to 10,000 then tax is 0. If salary is greater than 10,000 and less than equal to 20,000 then tax is 5%. If salary is greater than 20,000 and less than equal to 30,000 then tax is 7%. The tax on the salary greater than 30,000 is 10%. Note: On creation of each object, the value of static data member should be incremented. Within main () function, create an array of 5 objects of class Employee. Second object should be initialized with the first object. Now, use for loop to call calcNetPay() and display() functions for each object. You can create objects as follow: Employee emp1(5, "Ahmad", "Accounts", 20000 ); Employee emp2(emp1) ; Employee emp3(10, "Ayesha", "Accounts", 25000); Employee emp4(3, "Hassan", "Administration", 10000); Employee emp5(6, "Arsalan", "Administration", 35000)
write c++ program
Employee class has the following data members:
- Employee ID.
- Employee Name
- Department
- Employee Salary
- Static data member which will hold the value of total no. of employees
Employee class must have the following member functions:
Function |
Description |
Employee() |
Default constructor for Employee class, which will set employee id to 0, employee name to NULL, employee department to NULL and salary to 0.0 |
Employee(int, char *, char *, float); |
It will take employee id, employee name, department and salary as arguments and initialize their values using member initializer list |
Employee(Employee &); |
Copy constructor which will initialize one object with another object using deep copy |
Setter functions |
You have to define setter function for each data member which will take a value as an argument and set their values |
Getter functions |
You have to define getter function for each data member which will return the values of their data members |
calcNetPay() |
This function will calculate the net pay of an employee based on his salary |
display() |
This function will display the values of all data members of class |
~Employee() |
Destructor of Employee class which will decrement the value of static data member of class |
calcNetPay(): This function will calculate net pay of an employee based on his salary as:
If salary is less than and equal to 10,000 then tax is 0.
If salary is greater than 10,000 and less than equal to 20,000 then tax is 5%.
If salary is greater than 20,000 and less than equal to 30,000 then tax is 7%.
The tax on the salary greater than 30,000 is 10%.
Note: On creation of each object, the value of static data member should be incremented.
Within main () function, create an array of 5 objects of class Employee. Second object should be initialized with the first object.
Now, use for loop to call calcNetPay() and display() functions for each object.
You can create objects as follow:
Employee emp1(5, "Ahmad", "Accounts", 20000 );
Employee emp2(emp1) ;
Employee emp3(10, "Ayesha", "Accounts", 25000);
Employee emp4(3, "Hassan", "Administration", 10000);
Employee emp5(6, "Arsalan", "Administration", 35000)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

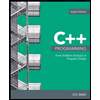
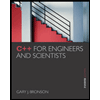
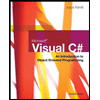
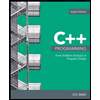
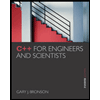
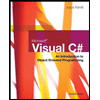