IN C++ You are required to build a class to represent the a cup of coffee. Call your class CoffeeCup. A coffee cup will have following characteristics 1. type (sting) // can be mocha, cupaccino, etc 2. temperature (float): represents the measure of temperature in degrees 3. volume (float):// the volume of coffee in the cup in ml liters 4. sugar (int):// no of teaspoons of sugar added to the coffee Provide these methods 1. parameterized constructor CoffeeCup(string t, float temp float vol, int sug) 2. Provide setters for temperature, and sugar and volume. 3. Provide getters of temperature, sugar, volume and type. 4. void heatUp(): //this method represents the process of heating the coffee, the current temperature of coffee goes up by one degree if it is below 100 and stays the same otherwise 5. void cooldown(): the process of cooling down. The temperature goes down by one degree but will not go below 0 6. bool isSweet():// returns true if this coffee has any amount of sugar in it and 0 if not at all 7. bool hasMore(): //this method checks if the cup has coffee in it that a person can take a sip from. For the implementation assume that one sip consumes a volume of 0.3 ml. So you will do some calculations in this method to check if it has any sips left depending upon the volume available. 8. bool isEmpty(): // returns true if a person can take a sip from this cup and false otherwise 9. bool takeASip():// this method represents the actual act of sipping. One sip is taken i.e. the volume equivalent to one sip is taken away. 10. Provide a method print, that displays the status of the cup with respect to all data members.
IN C++
You are required to build a class to represent the a cup of coffee. Call your class
CoffeeCup. A coffee cup will have following characteristics
1. type (sting) // can be mocha, cupaccino, etc
2. temperature (float): represents the measure of
temperature in degrees
3. volume (float):// the volume of coffee in the cup in ml
liters
4. sugar (int):// no of teaspoons of sugar added to the coffee
Provide these methods
1. parameterized constructor CoffeeCup(string t, float temp float vol, int sug)
2. Provide setters for temperature, and sugar and volume.
3. Provide getters of temperature, sugar, volume and type.
4. void heatUp(): //this method represents the process of heating the coffee, the current
temperature of coffee goes up by one degree if it is below 100 and stays the same otherwise
5. void cooldown(): the process of cooling down. The temperature goes down by one degree but
will not go below 0
6. bool isSweet():// returns true if this coffee has any amount of sugar in it and 0 if not at all
7. bool hasMore(): //this method checks if the cup has coffee in it that a person can take a sip
from. For the implementation assume that one sip consumes a volume of 0.3 ml. So you will do
some calculations in this method to check if it has any sips left depending upon the volume
available.
8. bool isEmpty(): // returns true if a person can take a sip from this cup and false otherwise
9. bool takeASip():// this method represents the actual act of sipping. One sip is taken i.e. the
volume equivalent to one sip is taken away.
10. Provide a method print, that displays the status of the cup with respect to all data members.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

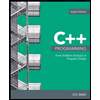
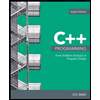