In c++. Please need rescurive arrays such as in picture from level 0 to level 1 to level 2. split into recursive functions. Using argumentmanger.h. here it is. #include #include #include #include using namespace std; // This is a class that can parse the commnad line arguments we use in COSC 2430 homework. class ArgumentManager { private: map m_argumentMap; public: ArgumentManager() { } ArgumentManager(int argc, char *argv[], char delimiter=';'); ArgumentManager(string rawArguments, char delimiter=';'); void parse(int argc,char*argv[],char delimiter=';'); void parse(string rawArguments,char delimiter=';'); string get(string argumentName); string toString(); friend ostream&operator<<(ostream &out, ArgumentManager &am); }; void ArgumentManager::parse(string rawArguments, char delimiter) { stringstream currentArgumentName; stringstream currentArgumentValue; bool argumentNameFinished =false; for(unsignedint i=0; i<=rawArguments.length(); i++){ if(i == rawArguments.length()|| rawArguments[i]== delimiter){ if(currentArgumentName.str()!=""){ m_argumentMap[currentArgumentName.str()] = currentArgumentValue.str(); } // reset currentArgumentName.str(""); currentArgumentValue.str(""); argumentNameFinished = false; } elseif(rawArguments[i]=='='){ argumentNameFinished = true; } else{ if(argumentNameFinished){ currentArgumentValue << rawArguments[i]; } else{ // ignore any spaces in argument names. if(rawArguments[i]==' ') continue; currentArgumentName << rawArguments[i]; } } } } void ArgumentManager::parse(int argc, char *argv[], char delimiter) { if(argc >1){ for(int i=1; i::iterator iter = m_argumentMap.find(argumentName); //If the argument is not found, return a blank string. if(iter == m_argumentMap.end()){ return""; } else{ return iter->second; } } string ArgumentManager::toString() { stringstream ss; for(map::iterator iter = m_argumentMap.begin(); iter != m_argumentMap.end(); iter++){ ss << "Argument name: " << iter->first << endl; ss << "Argument value: " << iter->second << endl; } return ss.str(); } ostream& operator << (ostream &out, ArgumentManager &am) { out << am.toString(); return out; }
In c++. Please need rescurive arrays such as in picture from level 0 to level 1 to level 2. split into recursive functions. Using argumentmanger.h. here it is. #include #include #include #include using namespace std; // This is a class that can parse the commnad line arguments we use in COSC 2430 homework. class ArgumentManager { private: map m_argumentMap; public: ArgumentManager() { } ArgumentManager(int argc, char *argv[], char delimiter=';'); ArgumentManager(string rawArguments, char delimiter=';'); void parse(int argc,char*argv[],char delimiter=';'); void parse(string rawArguments,char delimiter=';'); string get(string argumentName); string toString(); friend ostream&operator<<(ostream &out, ArgumentManager &am); }; void ArgumentManager::parse(string rawArguments, char delimiter) { stringstream currentArgumentName; stringstream currentArgumentValue; bool argumentNameFinished =false; for(unsignedint i=0; i<=rawArguments.length(); i++){ if(i == rawArguments.length()|| rawArguments[i]== delimiter){ if(currentArgumentName.str()!=""){ m_argumentMap[currentArgumentName.str()] = currentArgumentValue.str(); } // reset currentArgumentName.str(""); currentArgumentValue.str(""); argumentNameFinished = false; } elseif(rawArguments[i]=='='){ argumentNameFinished = true; } else{ if(argumentNameFinished){ currentArgumentValue << rawArguments[i]; } else{ // ignore any spaces in argument names. if(rawArguments[i]==' ') continue; currentArgumentName << rawArguments[i]; } } } } void ArgumentManager::parse(int argc, char *argv[], char delimiter) { if(argc >1){ for(int i=1; i::iterator iter = m_argumentMap.find(argumentName); //If the argument is not found, return a blank string. if(iter == m_argumentMap.end()){ return""; } else{ return iter->second; } } string ArgumentManager::toString() { stringstream ss; for(map::iterator iter = m_argumentMap.begin(); iter != m_argumentMap.end(); iter++){ ss << "Argument name: " << iter->first << endl; ss << "Argument value: " << iter->second << endl; } return ss.str(); } ostream& operator << (ostream &out, ArgumentManager &am) { out << am.toString(); return out; }
Programming Logic & Design Comprehensive
9th Edition
ISBN:9781337669405
Author:FARRELL
Publisher:FARRELL
Chapter8: Advanced Data Handling Concepts
Section: Chapter Questions
Problem 19RQ
Related questions
Question
In c++. Please need rescurive arrays such as in picture from level 0 to level 1 to level 2. split into recursive functions. Using argumentmanger.h. here it is.
#include <map>
#include <string>
#include <iostream>
#include <sstream>
using namespace std;
// This is a class that can parse the commnad line arguments we use in COSC 2430 homework.
class ArgumentManager {
private:
map<string, string> m_argumentMap;
public:
ArgumentManager() { }
ArgumentManager(int argc, char *argv[], char delimiter=';');
ArgumentManager(string rawArguments, char delimiter=';');
void parse(int argc,char*argv[],char delimiter=';');
void parse(string rawArguments,char delimiter=';');
string get(string argumentName);
string toString();
friend ostream&operator<<(ostream &out, ArgumentManager &am);
};
void ArgumentManager::parse(string rawArguments, char delimiter) {
stringstream currentArgumentName;
stringstream currentArgumentValue;
bool argumentNameFinished =false;
for(unsignedint i=0; i<=rawArguments.length(); i++){
if(i == rawArguments.length()|| rawArguments[i]== delimiter){
if(currentArgumentName.str()!=""){
m_argumentMap[currentArgumentName.str()] = currentArgumentValue.str();
}
// reset
currentArgumentName.str("");
currentArgumentValue.str("");
argumentNameFinished = false;
}
elseif(rawArguments[i]=='='){
argumentNameFinished = true;
}
else{
if(argumentNameFinished){
currentArgumentValue << rawArguments[i];
}
else{
// ignore any spaces in argument names.
if(rawArguments[i]==' ')
continue;
currentArgumentName << rawArguments[i];
}
}
}
}
void ArgumentManager::parse(int argc, char *argv[], char delimiter) {
if(argc >1){
for(int i=1; i<argc; i++){
parse(argv[i], delimiter);
}
}
}
ArgumentManager::ArgumentManager(int argc, char *argv[], char delimiter) {
parse(argc, argv, delimiter);
}
ArgumentManager::ArgumentManager(string rawArguments, char delimiter) {
parse(rawArguments, delimiter);
}
string ArgumentManager::get(string argumentName) {
map<string, string>::iterator iter = m_argumentMap.find(argumentName);
//If the argument is not found, return a blank string.
if(iter == m_argumentMap.end()){
return"";
}
else{
return iter->second;
}
}
string ArgumentManager::toString() {
stringstream ss;
for(map<string, string>::iterator iter = m_argumentMap.begin(); iter != m_argumentMap.end(); iter++){
ss << "Argument name: " << iter->first << endl;
ss << "Argument value: " << iter->second << endl;
}
return ss.str();
}
ostream& operator << (ostream &out, ArgumentManager &am) {
out << am.toString();
return out;
}
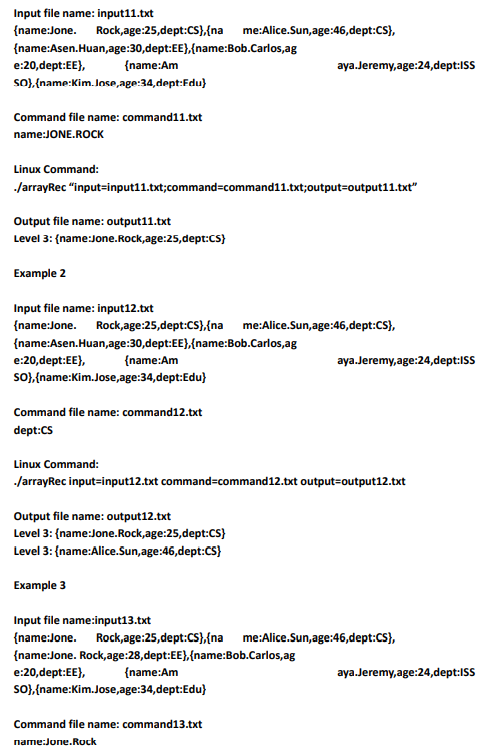
Transcribed Image Text:Input file name: input11.txt
{name:Jone. Rock,age:25,dept:CS},{na me:Alice.Sun,age:46,dept:CS},
{name:Asen.Huan,age:30,dept:EE},{name:Bob.Carlos,ag
e:20,dept:EE},
{name:Am
aya.Jeremy,age:24,dept:ISS
SO), {name:Kim. Inse,age:34,dept:Fdu)
Command file name: command11.txt
name:JONE.ROCK
Linux Command:
JarrayRec "input=input11.txt;command=command11.txt;output=output11.txt"
Output file name: output11.txt
Level 3: {name:Jone.Rock,age:25,dept:CS)
Example 2
Input file name: input12.txt
{name:Jone. Rock,age:25,dept:CS},{na me:Alice.Sun,age:46,dept:CS),
{name:Asen.Huan,age:30,dept:EE},{name:Bob.Carlos,ag
e:20,dept:EE),
So),(name:Kim.Jose,age:34,dept:Edu}
{name:Am
aya.Jeremy,age:24,dept:ISS
Command file name: command12.txt
dept:CS
Linux Command:
/arrayRec input=input12.txt command=command12.txt output=output12.txt
Output file name: output12.txt
Level 3: {name:Jone.Rock,age:25,dept:Cs}
Level 3: {name:Alice.Sun,age:46,dept:CS}
Example 3
Input file name:input13.txt
{name:Jone. Rock,age:25,dept:CS},{na me:Alice.Sun,age:46,dept:CS),
{name:Jone. Rock,age:28,dept:EE},{name:Bob.Carlos,ag
e:20,dept:EE},
{name:Am
aya.Jeremy,age:24,dept:ISS
so),{name:Kim.Jose,age:34,dept:Edu}
Command file name: command13.txt
name:Jone.Rock
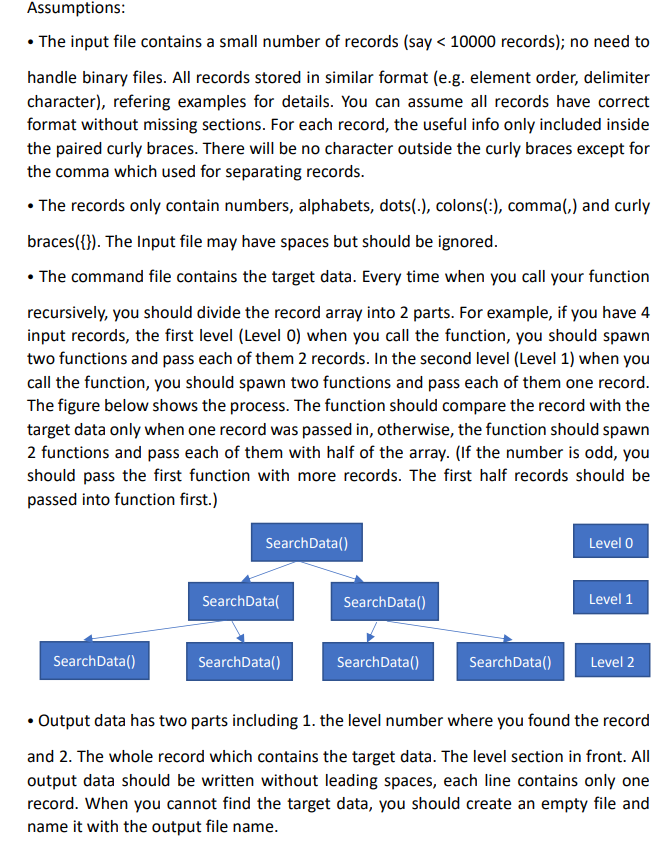
Transcribed Image Text:Assumptions:
• The input file contains a small number of records (say < 10000 records); no need to
handle binary files. All records stored in similar format (e.g. element order, delimiter
character), refering examples for details. You can assume all records have correct
format without missing sections. For each record, the useful info only included inside
the paired curly braces. There will be no character outside the curly braces except for
the comma which used for separating records.
• The records only contain numbers, alphabets, dots(.), colons(:), comma(,) and curly
braces({}). The Input file may have spaces but should be ignored.
• The command file contains the target data. Every time when you call your function
recursively, you should divide the record array into 2 parts. For example, if you have 4
input records, the first level (Level 0) when you call the function, you should spawn
two functions and pass each of them 2 records. In the second level (Level 1) when you
call the function, you should spawn two functions and pass each of them one record.
The figure below shows the process. The function should compare the record with the
target data only when one record was passed in, otherwise, the function should spawn
2 functions and pass each of them with half of the array. (If the number is odd, you
should pass the first function with more records. The first half records should be
passed into function first.)
SearchData()
Level 0
SearchData(
SearchData()
Level 1
SearchData()
SearchData()
SearchData()
SearchData()
Level 2
• Output data has two parts including 1. the level number where you found the record
and 2. The whole record which contains the target data. The level section in front. All
output data should be written without leading spaces, each line contains only one
record. When you cannot find the target data, you should create an empty file and
name it with the output file name.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage